How to Wrap an Element with Another Div Using jQuery?
Last Updated :
29 Feb, 2024
We will see how to wrap an element with another div in jQuery. We will simply wrap an element inside a div tag in jQuery.
These are the following approaches:
- We include the jQuery library in the <head> section.
- We have a <div> with the ID elementToWrap, which contains the content you want to wrap.
- In the jQuery script, we use the .wrap() method to wrap #elementToWrap with another <div> with the class wrapper.
- Optional CSS is provided to style the wrapper <div> for demonstration purposes.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width
, initial-scale = 1 .0">
< title >Wrap Element with Another Div</ title >
< script src =
< script >
$(document).ready(function () {
// Wrap the element with another < div >
$('#elementToWrap').wrap('< div class = "wrapper" ></ div >');
});
</ script >
< style >
/* Styling for wrapper and wrapped divs */
.wrapper {
border: 2px solid #ccc;
padding: 10px;
margin: 20px;
/* Added margin for spacing */
}
#elementToWrap {
background-color: #f0f0f0;
padding: 10px;
}
</ style >
</ head >
< body >
< div id = "elementToWrap" >
Wrapped content
</ div >
</ body >
</ html >
|
Output:
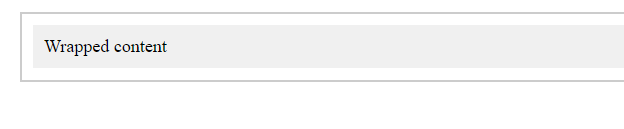
In this appraoch, we wrap the content inside a <div> with the ID elementToWrap using jQuery’s .wrapInner() method, applying styles to the wrapper and wrapped <div> for visual distinction, including borders, background colors, padding, margin, font size, and box shadow.
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Wrap Element with Another Div</ title >
< script src =
< script >
$(document).ready(function () {
// Wrap the content inside the
// element with another < div >
$('#elementToWrap').wrapInner('< div class = "wrapper" ></ div >');
});
</ script >
< style >
/* Styling for wrapper and wrapped divs */
.wrapper {
border: 2px solid #007bff;
/* Blue border */
background-color: #f0f0f0;
/* Light gray background */
padding: 10px;
margin: 20px;
/* Added margin for spacing */
}
#elementToWrap {
font-size: 16px;
/* Larger font size */
color: #333;
/* Dark text color */
background-color: #fff;
/* White background */
padding: 20px;
/* Increased padding */
border: 1px solid #ccc;
/* Light gray border */
border-radius: 5px;
/* Rounded corners */
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
/* Box shadow */
}
</ style >
</ head >
< body >
< div id = "elementToWrap" >
This is the content you want to wrap.
</ div >
</ body >
</ html >
|
Output:
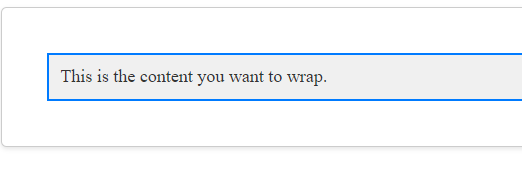
Share your thoughts in the comments
Please Login to comment...