How to use Try Catch and Finally in TypeScript ?
Last Updated :
16 Jan, 2024
The try, catch and finally blocks are used for error handling and state management while performing asynchronous tasks. It is an effective way to handle errors and state on the web page. This tutorial will explain to you the use of the try, catch, and finally statements practically.
Explanation:
- try: The try block will run a block of code and check for its correctness and errors.
- catch: The catch block will catch the error thrown by the try block and print it to your desired location.
- finally: The final statement runs always irrespective of try and catch, it is independent of them.
Syntax:
try{
// A block of code to check for errors
}
catch(error){
// Displays error thrown by the try block
}
finally{
// Runs irrespective of try and catch blocks
}
Example: The below code example implements the try-and-catch blocks in TypeScript.
Javascript
function codeForTryBlock(a: number, b: number) {
const num1: number = a;
const num2: number = b;
if (num1 === num2) {
console.log( "Provided Numbers are same!!" );
}
else {
throw new Error( "Provided numbers are not same!!" );
}
}
function demoImplementation() {
try {
codeForTryBlock(3, 5);
} catch (error: any) {
console.error( "An error Occured: " , error.message);
} finally {
console.log(
"This text is from finally block it runs irrespective of try-catch blocks." );
}
}
demoImplementation();
|
Output:
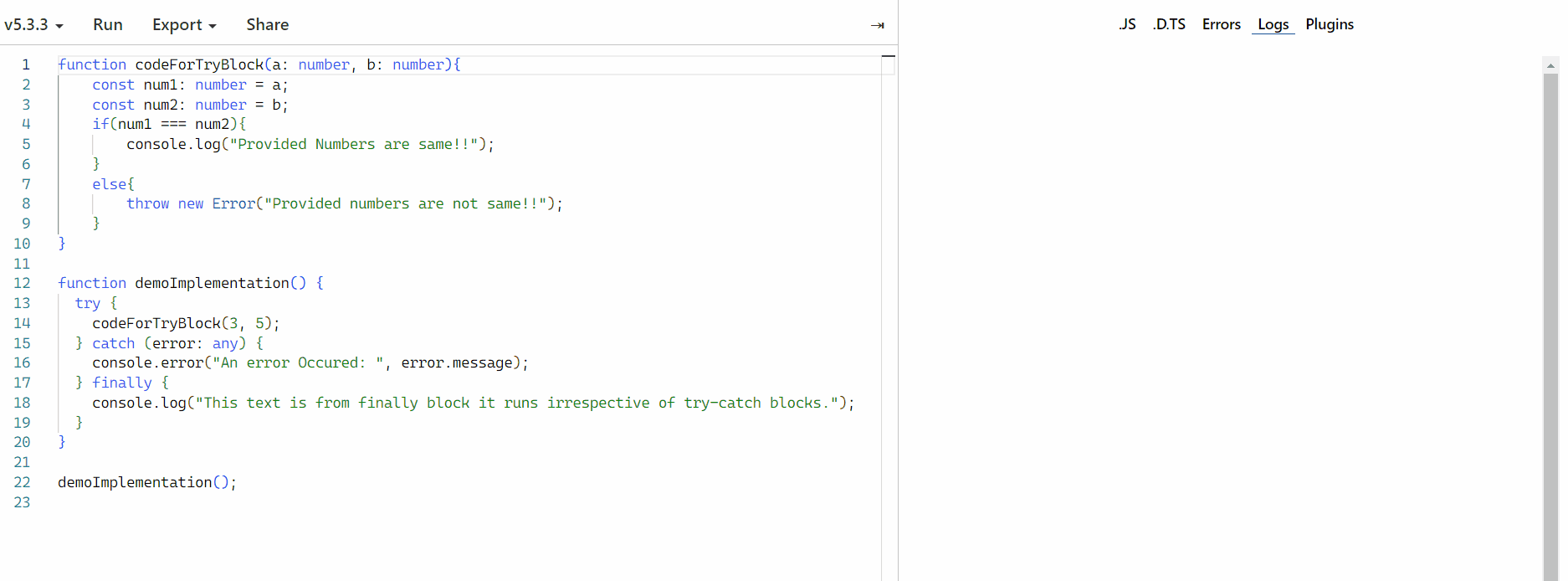
Example: The below code example implements the try, catch and finally to fetch data from an API.
Javascript
async function fetchGET(url: string): Promise<any> {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(
`Unable to Fetch Data, Please check
URL or Network connectivity!!`
);
}
const data = await response.json();
return data.results[0];
}
catch (error: any) {
console.error( "An Error Occured: " , error.message)
}
finally {
console.log(
"This code is from finally block of fetchGET function and it will run always." );
}
}
async function fetchData() {
const returnedData =
console.log(returnedData);
}
fetchData();
|
Output:
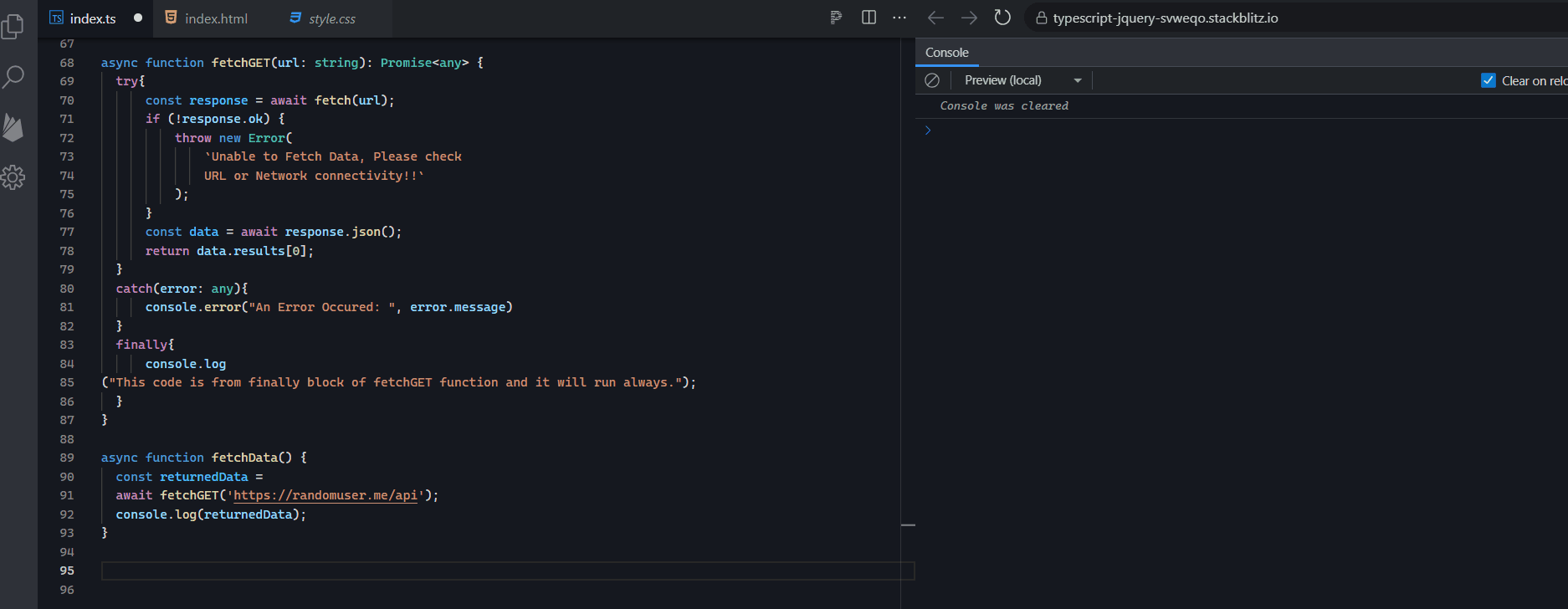
Share your thoughts in the comments
Please Login to comment...