How to style even and odd elements using CSS ?
Last Updated :
09 Jan, 2024
In this article, we will learn how to style even and odd elements using CSS. Styling every element uniformly may not always be the best approach. you’ll explore multiple approaches to differentiate between even and odd elements. This is particularly valuable for designing lists, tables, or structured content, where alternating styles enhance readability.
The following techniques can be used to style the elements in an alternative order:
We will explore the above approaches with the help of suitable examples.
Using the nth-child Selector
The nth-child selector is a powerful CSS tool that allows us to style elements based on their position within a group.
Example: In this example, we will see the implementation of styling even and odd elements using the nth-child selector.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< link rel = "stylesheet"
href = "style.css" >
< title >Even and Odd Styling</ title >
</ head >
< body >
< h1 style = "text-align: center;" >
GeeksforGeeks
</ h1 >
< div class = "container" >
< div class = "box" >Box 1</ div >
< div class = "box" >Box 2</ div >
< div class = "box" >Box 3</ div >
< div class = "box" >Box 4</ div >
</ div >
< p style = "text-align: center;" >
Example of "Using the nth-child Selector"
</ p >
</ body >
</ html >
|
CSS
.container {
margin-top : 40px ;
display : flex;
justify- content : space-around;
align-items: center ;
margin-bottom : 40px ;
}
.box {
width : 100px ;
height : 100px ;
text-align : center ;
line-height : 100px ;
}
.box:nth-child(even) {
background-color : green ;
}
.box:nth-child(odd) {
background-color : red ;
}
|
Output:
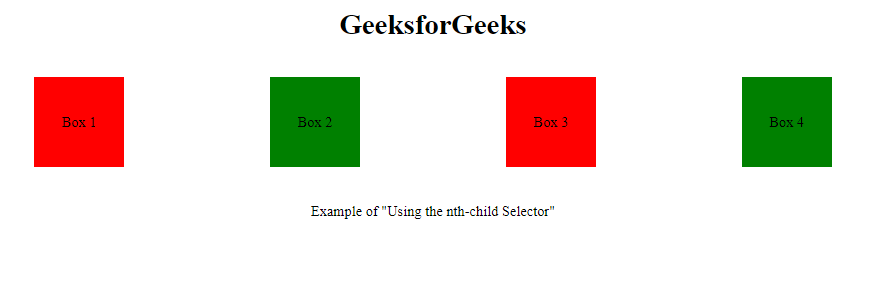
Using Classes
Assigning classes to even and odd elements provides a more explicit and flexible styling method and we can easily access the element by using ( . ) dot.
Example: In this example, we will see the implementation of styling even and odd elements using classes.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< link rel = "stylesheet"
href = "style.css" >
< title >Even and Odd Styling</ title >
</ head >
< body >
< h1 style = "text-align: center;" >
GeeksforGeeks
</ h1 >
< div class = "container" >
< div class = "box even" >Box 1</ div >
< div class = "box odd" >Box 2</ div >
< div class = "box even" >Box 3</ div >
< div class = "box odd" >Box 4</ div >
</ div >
< p style = "text-align: center;" >
Example of "Using Classes"
</ p >
</ body >
</ html >
|
CSS
.container {
display : flex;
justify- content : space-around;
}
.box {
width : 100px ;
height : 100px ;
text-align : center ;
line-height : 100px ;
padding : 10px ;
}
.even {
background-color : blue ;
}
.odd {
background-color : magenta;
}
|
Output:
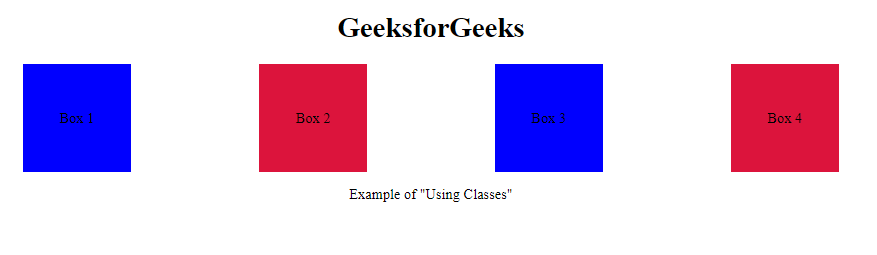
Using JavaScript
Leveraging JavaScript offers dynamic styling control based on element positions, ideal for complex scenarios.
Example: In this example, we will see the implementation of styling even and odd elements using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< link rel = "stylesheet"
href = "style.css" >
< title >Even and Odd Styling</ title >
</ head >
< body >
< h1 style = "text-align: center;" >
GeeksforGeeks
</ h1 >
< div class = "container" >
< div class = "box" >Box 1</ div >
< div class = "box" >Box 2</ div >
< div class = "box" >Box 3</ div >
< div class = "box" >Box 4</ div >
</ div >
< p style = "text-align: center;" >
Example of "Using JavaScript"
</ p >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
.container {
display : flex;
justify- content : space-around;
}
.box {
width : 100px ;
height : 100px ;
text-align : center ;
line-height : 100px ;
padding : 10px ;
}
|
Javascript
document.addEventListener( 'DOMContentLoaded' , function () {
const boxes = document.querySelectorAll( '.box' );
boxes.forEach((box, index) => {
if (index % 2 === 0) {
box.style.backgroundColor = 'gray' ;
} else {
box.style.backgroundColor = 'yellow' ;
}
});
});
|
Output:
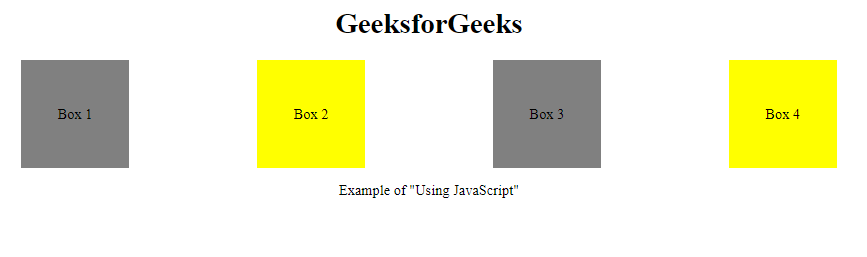
Share your thoughts in the comments
Please Login to comment...