How to Setup MySQL Database in Visual Studio 2022 For a C++ Application?
Last Updated :
02 Nov, 2023
Databases play a crucial role in storing, retrieving, and managing data efficiently. MySQL, a popular open-source Relational Database Management System, is widely used for this purpose. C++ acts as the intermediary connecting user interfaces and MySQL databases, enabling developers to easily retrieve, edit, and store data. It provides the essential resources and libraries for establishing connections, running queries, and managing data smoothly.
This article provides a comprehensive guide on setting up a MySQL database with C++ in Visual Studio 2022 to enable database functionality for an application.
Configuring MySQL For C++ Application in Visual Studio
Essentially, the partnership between C++ and MySQL is a valuable collaboration that empowers developers to effortlessly build efficient, data-focused applications. This feature allows developers to tap into the complete potential of their data and deliver strong solutions to their users.
Follow the further steps to connect MySQL database with C++.
Step 1: Install Visual Studio 2022 Community Edition.
- Select and Install C++ Components for your desired project development.
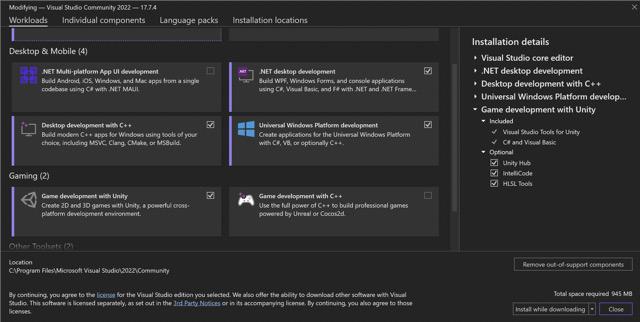
Step 2: Install MySQL Connector/C++ Library.
- Download the MySQL Connector/C++ library from the MySQL website.
- Download Connector/C++ library zip for your system and placed it in a secure directory.
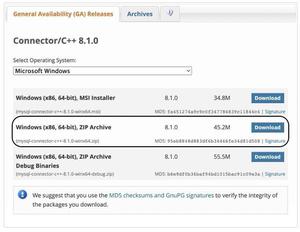
Step 3: Open Visual Studio 2022 and Select Create a new Project.
- Select a Empty C++ Project.
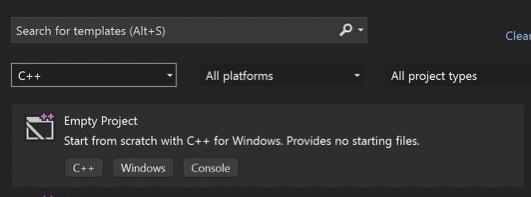
- Provide a name for your project and click Create.
- Right Click on Project Solution -> Add -> New Item . Add a new CPP file to project.
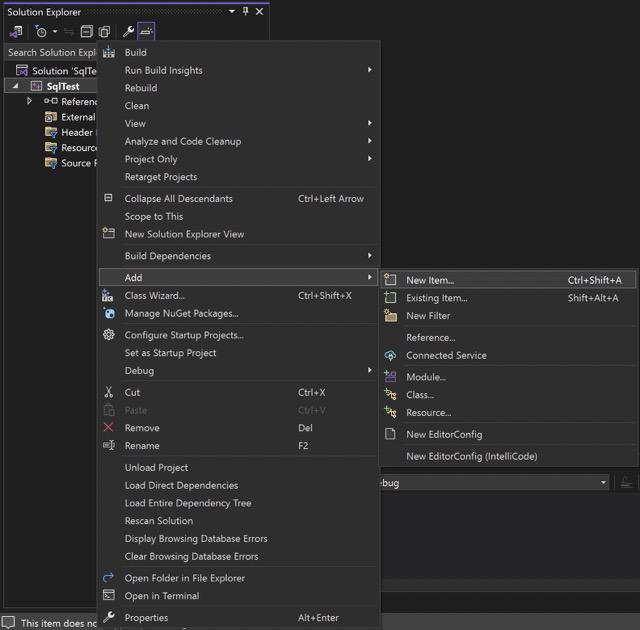
- Select appropriate Solution configuration (Release) and Platform (x64) as per downloaded Connector file.

Checkout this sample code for test purpose.
Example:
C++
#include <cppconn/driver.h>
#include <cppconn/exception.h>
#include <cppconn/statement.h>
#include <iostream>
#include <mysql_connection.h>
#include <mysql_driver.h>
using namespace std;
int main()
{
try {
sql::mysql::MySQL_Driver* driver;
sql::Connection* con;
driver = sql::mysql::get_mysql_driver_instance();
"root" , "" );
con->setSchema( "test" );
sql::Statement* stmt;
stmt = con->createStatement();
string createTableSQL
= "CREATE TABLE IF NOT EXISTS GFGCourses ("
"id INT NOT NULL AUTO_INCREMENT PRIMARY KEY,"
"courses VARCHAR(255) NOT NULL"
")" ;
stmt->execute(createTableSQL);
string insertDataSQL
= "INSERT INTO GFGCourses (courses) VALUES "
"('DSA'),('C++'),('JAVA'),('PYTHON')" ;
stmt->execute(insertDataSQL);
string selectDataSQL = "SELECT * FROM GFGCourses" ;
sql::ResultSet* res
= stmt->executeQuery(selectDataSQL);
int count = 0;
while (res->next()) {
cout << " Course " << ++count << ": "
<< res->getString( "courses" ) << endl;
}
delete res;
delete stmt;
delete con;
}
catch (sql::SQLException& e) {
std::cerr << "SQL Error: " << e.what() << std::endl;
}
return 0;
}
|
- Now RUN the project. You will see many error will appear including missing header files. Let’ s move to the next step.
Step 4: Linking Connector/C++ Libraries with Project.
In this procedure we are setting up the environment for the application to locate necessary dependencies and linking libraries and drivers for the project which are necessary part of compilation process.
- Click on Project located at top-right corner , then select Project Properties (last option) .
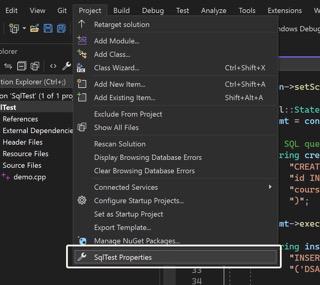
- A pop up for Project configuration menu appears.
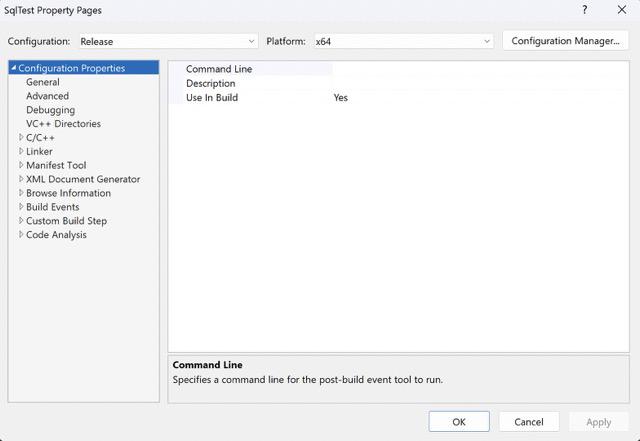
In the “Configuration Properties,” configure the following settings:
- Verify the Configuration (Release) and Platform (x64) exactly as per project.
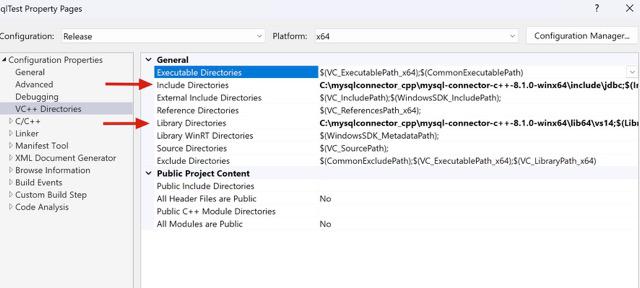
- Under “VC++ Directories” add the path to the MySQL Connector/C++ header files in “Include Directories.”
( sql header files path ).
Header File Directories : your-downloaded-folder-path...\mysql-connector-c++-8.1.0-winx64\include\jdbc
- Under “VC++ Directories” add the path to the MySQL Connector/C++ library files in “Library Directories.” ( .lib files path ).
Library Directories : your-downloaded-folder-path...\mysql-connector-c++-8.1.0-winx64\lib64\vs14
In this process we are linking necessary header files and libraries which helps to establish connection and link all MySQL drivers for the project.
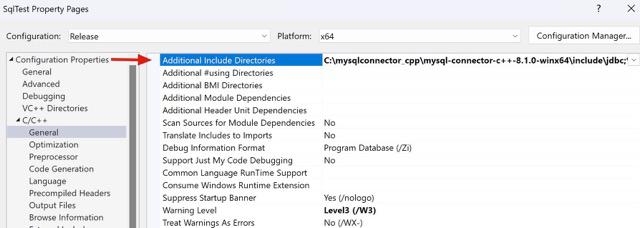
- Under “C/C++” add the path to the MySQL Connector/C++ header files in to “Additional Include Directories.”
Additional Include Directories : your-downloaded-folder-path...\mysql-connector-c++-8.1.0-winx64\include\jdbc
- In “C/C++” set the “Preprocessor Definitions” to include _CRT_SECURE_NO_WARNINGS; (just before NDEBUG; with semicolon ) to suppress warnings related to the use of certain C standard library functions.
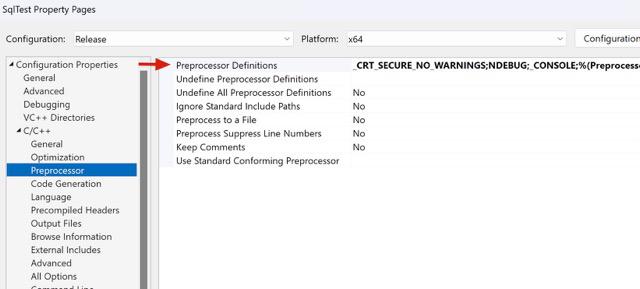
Above processes ensures the compiler to relocate necessary MySQL files required for compilation and suppress unnecessary warnings.
- Under “Linker” choose ” Input ” add library ” mysqlcppconn.lib” ( just file name ) to “Additional Dependencies” .
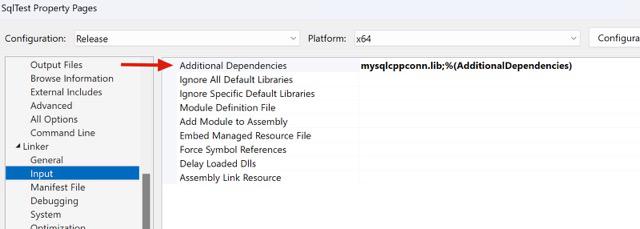
Adding Mysql Connection library to prepare database and application to ready for execution.
- Click “Apply” and then “OK“
Step 5: Now Rebuilt and Run the Project.
Step 6: There is a chance of getting an error of missing ” .DLL ” files .
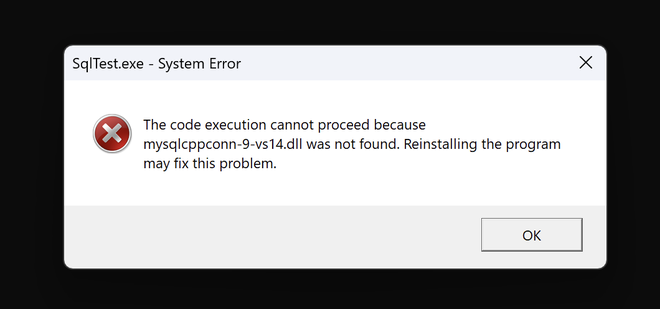
What are .DLL files ?
DLL files contain code and data that multiple programs can use simultaneously. These files allow programs to share common code and resources. DLLs contain functions, classes, and resources that can be shared across multiple applications. This means that developers can create a single DLL file that performs specific tasks (e.g., handling graphics or database operations) and then have multiple programs use that DLL to perform those tasks without duplicating the code.
Follow the below instructions.
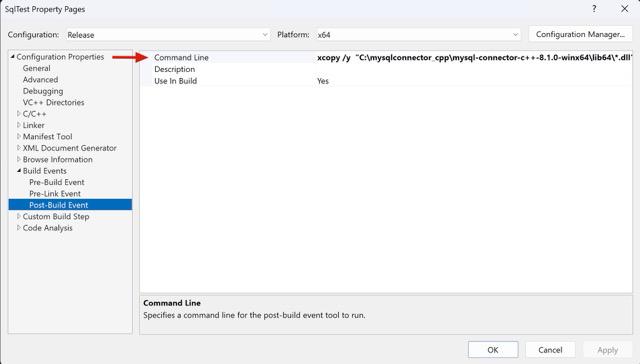
- Again click on Project located at top-right corner , then select Project Properties (last option) .
- In your project’s properties, go to “Configuration Properties” > “Build Events” > “Post-Build Event.”
- Under Command Line add a command to copy all DLLs to the output directory.
Command : xcopy /y "your-folder-path\mysql-connector-c++-8.1.0-winx64\lib64\*.dll"
This command will copy all DLLs from the specified directory to the output directory, ensuring they are available when you run your application from Visual Studio.
- Click on ” Apply “ and ” OK “.
Step 5: Rebuilt and Run the project.
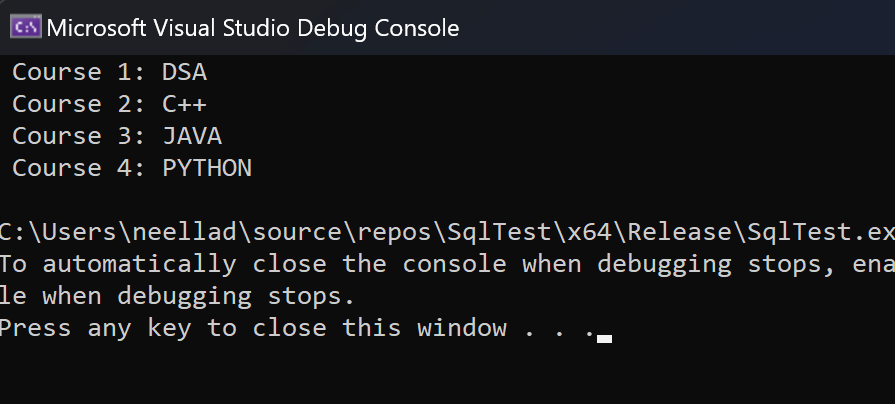
- Updated Records in Database.
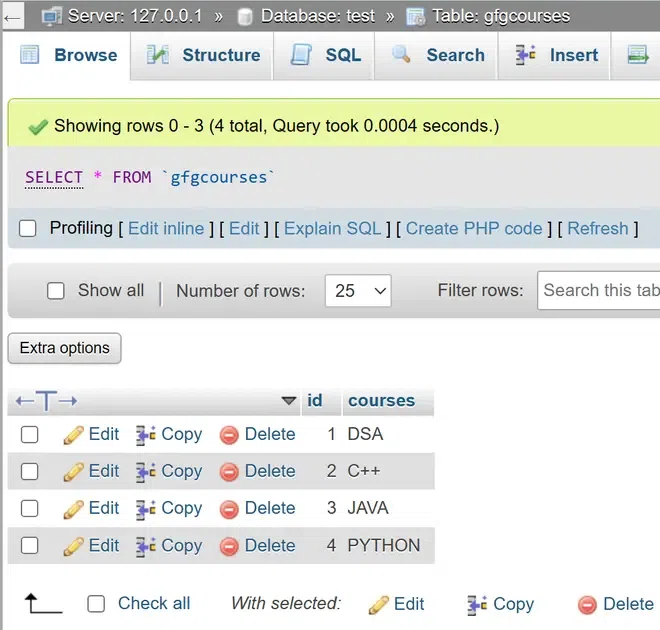
Share your thoughts in the comments
Please Login to comment...