How to Select the Nth Row in a SQLite Database Table?
Last Updated :
26 Mar, 2024
In SQLite, selecting a specific row from a table can be a common requirement, especially when dealing with large datasets. In this article, we will explore different methods to select the nth row from a SQLite database table.
Whether we’re a beginner or an experienced developer understanding these methods can help us efficiently retrieve specific data from our SQLite database.
How to Select nth Row in a SQLite Table?
When working with an SQLite database table there may be scenarios where we need to select a specific row based on its position in the table such as selecting the 5th row, the 10th row or any other nth row.
SQLite does not have a built-in function to directly select the nth row like some other databases. Below is the method that helps us to Select the nth Row in an SQLite Table as follows:
Let’s set up an Environment
- Using LIMIT and OFFSET
- Using Subqueries
- Using Window Functions
To understand how to Select the nth Row in a SQLite Table we need a table on which we will perform various operations and queries. Here we will consider a table called sample_table which contains the id and name as Columns.
CREATE TABLE sample_table (
id INTEGER PRIMARY KEY,
name TEXT
);
Now, let’s insert some sample data into the sample_table.
INSERT INTO sample_table (name) VALUES
('Alice'),
('Bob'),
('Charlie'),
('David'),
('Emma'),
('Frank');
Output:
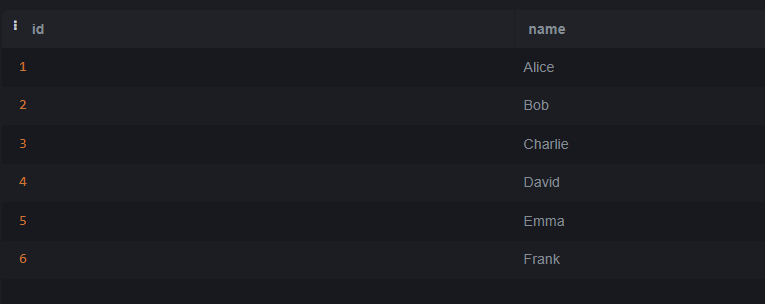
1. Using LIMIT and OFFSET
This method involves using the LIMIT and OFFSET clauses in the SQL query to select a specific row. LIMIT is used to restrict the number of rows returned by the query, while OFFSET skips a specified number of rows before beginning to return rows.
SELECT * FROM sample_table LIMIT 1 OFFSET 2;
Output:

Explanation: This query retrieves one row from the sample_table starting from the third row. It’s important to note that OFFSET is zero-based, so OFFSET 2 skips the first two rows.
2. Using Subqueries
Subqueries involve nesting a query within another query. In this method a subquery is used to retrieve the rowid of the desired row, and then the outer query selects the row based on that rowid.
SELECT * FROM sample_table WHERE rowid = (SELECT rowid FROM sample_table LIMIT 1 OFFSET 2);
Output:

Explanation: The inner subquery (SELECT rowid FROM sample_table LIMIT 1 OFFSET 2) retrieves the rowid of the third row. Then, the outer query selects the row from sample_table where the rowid matches the value obtained from the subquery.
3. Using Window Functions
Window functions are a feature introduced in SQLite version 3.25.0 that provide additional analytical capabilities. This method utilizes the ROW_NUMBER() window function to assign a sequential integer to each row allowing us to easily select a specific row based on its row number.
SELECT * FROM (SELECT *, ROW_NUMBER() OVER() AS row_num FROM sample_table) WHERE row_num = 3;
Output:

Explanation:
- The inner subquery (SELECT *, ROW_NUMBER() OVER() AS row_num FROM sample_table) adds a column row_num to each row, assigning a sequential integer to represent its position.
- The outer query then selects the row where row_num equals 3 effectively retrieving the third row from the sample_table.
Each of these queries will return the 3rd row from the sample_table which contains the name ‘Charlie’. We can replace 2 with any desired value of n to select the nth row.
Conclusion
Overall, To select the nth row from a SQLite database table, you can use methods such as LIMIT and OFFSET clauses, the ROWID column, or a subquery. The LIMIT and OFFSET clauses are straightforward, allowing you to specify the number of rows to skip and the number of rows to return, respectively. The ROWID column represents the unique identifier assigned to each row by SQLite and can be used to directly access a specific row. Using a subquery is another option, where you first select the rowid of the nth row and then use it to fetch the corresponding row.
Share your thoughts in the comments
Please Login to comment...