How to Run Selenium Test on Firefox?
Last Updated :
11 Dec, 2023
Selenium is a popular open-source tool for automating web browser interactions. Firefox is a fast and secure web browser developed by Mozilla. Firefox uses GeckoDriver to control and interact with Firefox during automated testing. GeckoDriver is a component of the Selenium automation framework that allows communication between the Selenium WebDriver and the Firefox web browser.
What is GeckoDriver?
GekoDriver is designed for interacting with the Mozilla Firefox web browser, it acts as a bridge between the Selenium WebDriver API and the internal communication protocols of Firefox. Allowing developers and testers to automate interaction with Firefox.
Why is GeckoDriver Used?
GeckoDriver is used to automate web testing with the Firefox browser.
- It allows you to control browser behavior and perform actions on web elements.
- GeckoDriver can be used with various programming languages, such as Java, Python, Ruby, etc.
Running Tests on Firefox
Step 1: Setup Test EnvironmentÂ
1. Install Java on your system.
2. After installing Java on your system or already installing, verify the installation by opening a command prompt and typing:
Java -version
3. Install Eclipse IDE on your system.
4. Install Selenium WebDriver for Java on your system.
Step 2: Download GeckoDriver
1. Â Go to the Mozilla GeckoDriver GitHub page.
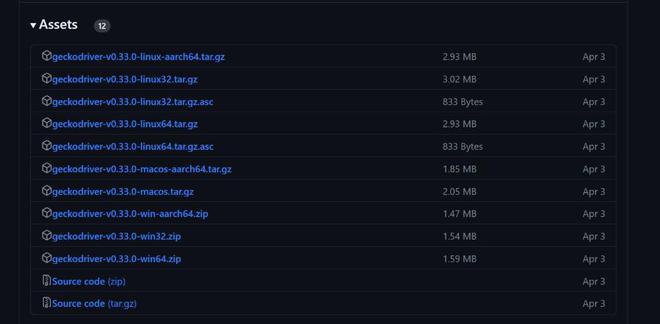
2. Download the latest version of GeckoDriver that is compatible with your installed version of Firefox. Extract the zip file and copy the path of the geckodriver.exe (e.g., D:/geckodriver-v0.33.0-win64/geckodriver.exe).
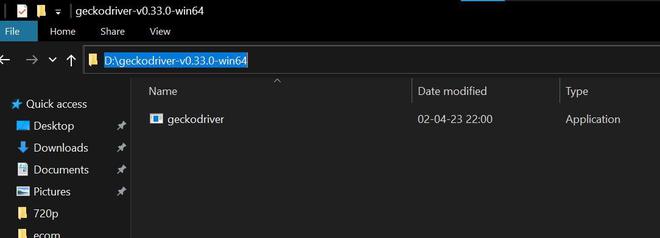
Step 3: Configure the Project
1. Create a new Java project in Eclipse IDE. In Eclipse, go to File > New > Java Project. Give a name to your project, such as `SeleniumTest`, and click Finish.
2. Add the downloaded Selenium JAR files to your Java project’s build path. Select all the jar files and click Apply and close.
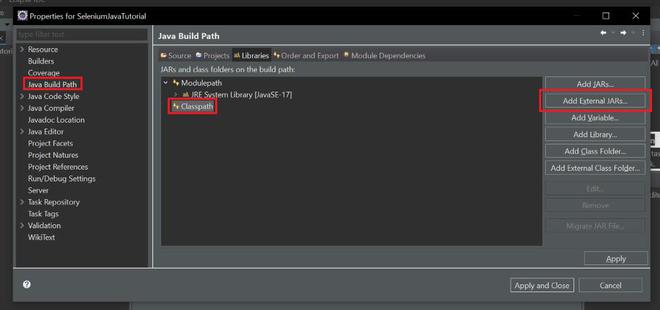
3. Create a new Java class in the project. In Eclipse, right-click on the src folder under your project name and select New > Class. Give a name to your class, such as `TestFirefox`, and click Finish.
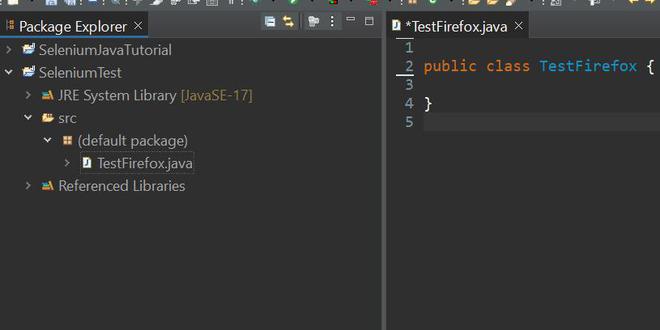
3. Import the required packages
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
4. Set the system property for FirefoxDriver (path to firefoxdriver executable).
(e.g.,D:/geckodriver-v0.33.0-win64/geckodriver.exe).
System.setProperty("webdriver.gecko.driver", "D:/geckodriver-v0.33.0-win64/geckodriver.exe");
5. Create an instance of FirefoxDriver.
WebDriver driver = new FirefoxDriver();
Below is the Java program to search on Firefox:
Java
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
public class TestFirefox {
public static void main(String[] args)
{
System.setProperty(
"webdriver.gecko.driver" ,
"D:/geckodriver-v0.33.0-win64/geckodriver.exe" );
WebDriver driver = new FirefoxDriver();
WebElement element
= driver.findElement(By.name( "q" ));
element.sendKeys( "GeeksforGeeks" );
element.submit();
try
{
Thread.sleep( 5000 );
}
catch (InterruptedException e)
{
e.printStackTrace();
}
List<WebElement> searchResults
= driver.findElements(By.xpath(
"//div[@class='tF2Cxc']//a[@href]" ));
if (searchResults.size() > 0 )
{
searchResults.get( 0 ).click();
}
else
{
System.out.println( "No search results found." );
}
String title = driver.getTitle();
System.out.println( "Page Title: " + title);
driver.quit();
}
}
|
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...