How to run Node/Express on a specific port?
Last Updated :
17 Dec, 2023
Port in Node is the numerical endpoint that is assigned to the process to allow communication over the network. Each application is identified uniquely by using this port number. There are port numbers that range from 0 to 65535, some of the ports are already assigned to the common services. In this article, we are going to learn how we can run a node on a specific port rather than using the default port. We will run a node on a specific port by using the two approaches.
Prerequisites
We will discuss the following two approaches to run Node/Express on a specific port
Steps to use middleware chaining in Express JS
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express
Step 4: Now create the below Project Structure of our project which includes the file as app.js.
Project Structure:
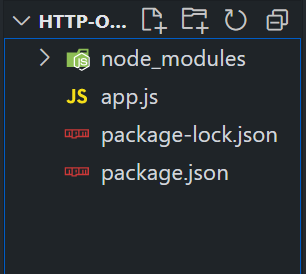
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2"
}
Approach 1: By Specifying Port in Code
We will specify the port explicitly in the code within the Node.js application. The server is then configured to listen in this predetermined port by setting and communicating the desired port for the application.
Syntax:
const port = 3000; // Specify the desired port
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Example: In the below example, we have specified the predetermined port in the code which is set to 4000. So the node application is running on Port 4000.
Javascript
const http = require( 'http' );
const port = 4000;
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end(`Hello, GeeksforGeeks! Server is running on port ${port}`);
});
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Output:
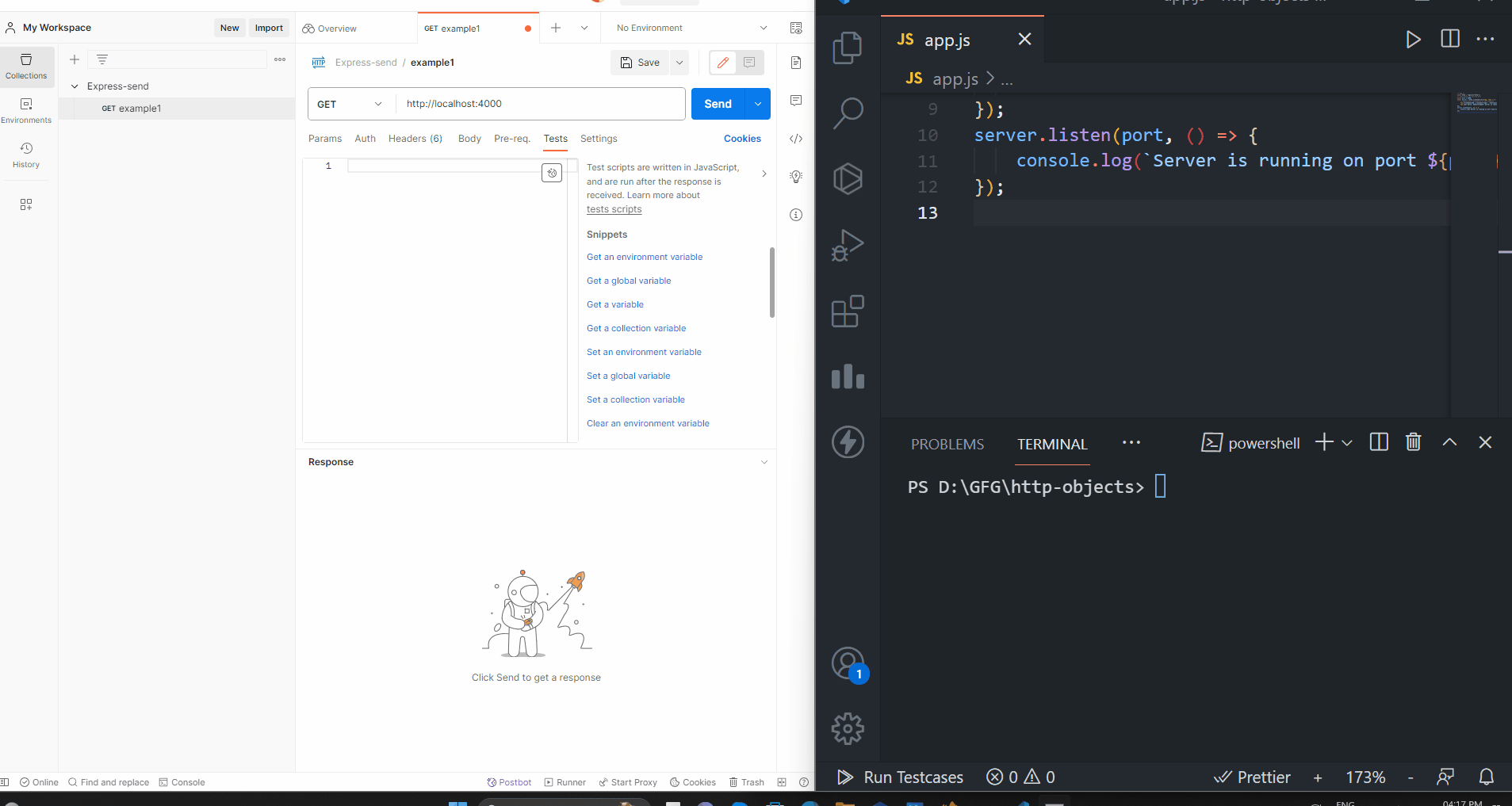
Approach 2: Using Environment Variable
We will be using the Environment Variable to dynamically determine the port rather than modifying the actual source code. Using this method, we can properly specify the port in execution time rather than predetermining.
Syntax:
const port = process.env.PORT || 3000;
Example: In the below example, we are running the application on Port number 6000 which is set by using the Environment Variable in terminal.
Javascript
const http = require( 'http' );
const port = process.env.PORT || 3000;
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end(`Hello, Geek! Server is running on port ${port}`);
});
server.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Output:
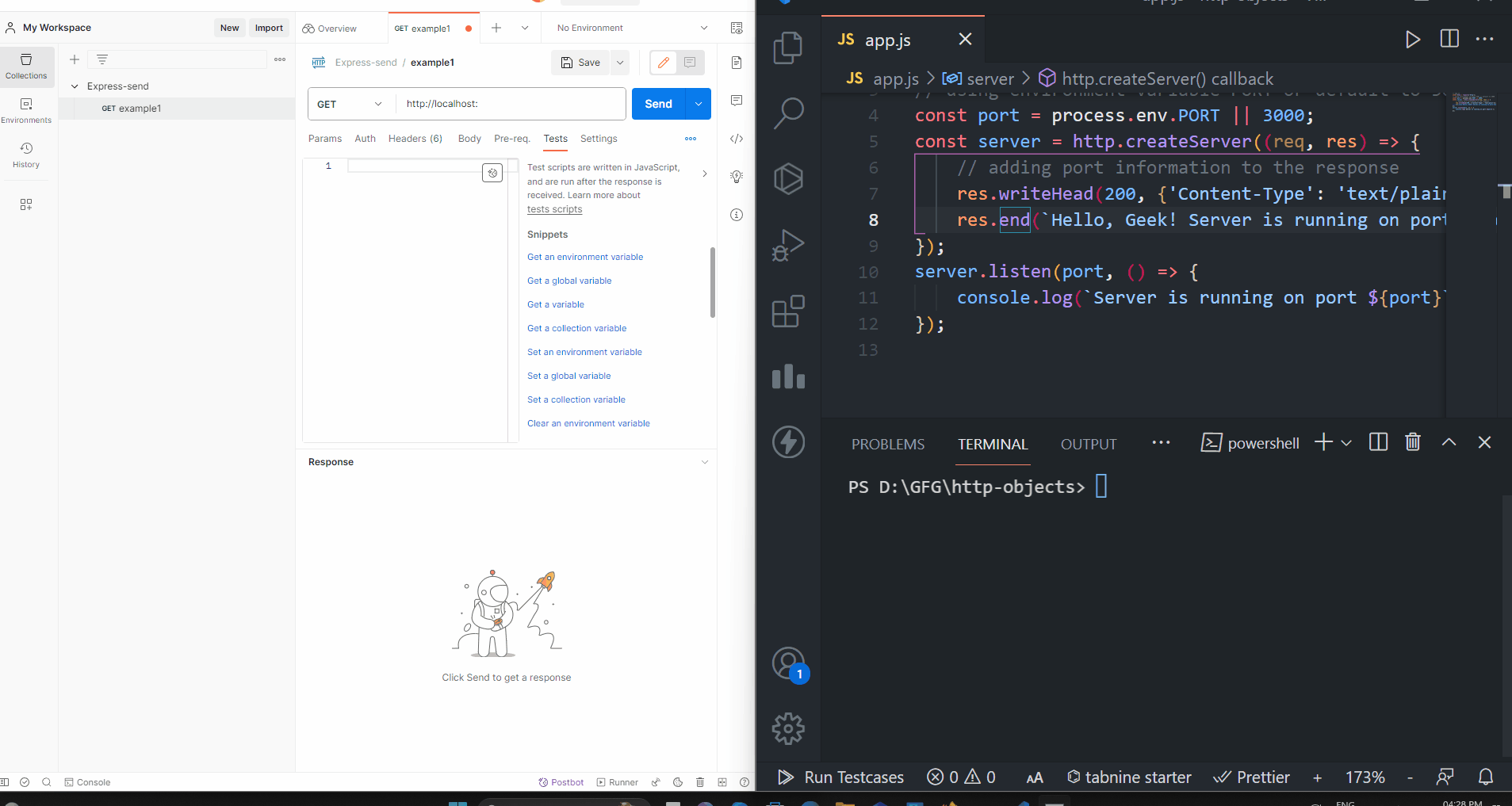
Share your thoughts in the comments
Please Login to comment...