How to Resolve form-data is getting undefined in Node/Express API ?
Last Updated :
14 Jan, 2024
Working with forms and handling data submissions is a basic requirement in web development. When using Node and Express for building APIs, you might get an error that the FormData object is undefined.
In this article, we’ll explore how to resolve form data getting undefined in a Node/Express API.
The FormData object is a part of the Web API and is commonly used to construct a set of key/value pairs representing form fields and their values. It is often used in the frontend to send form data to a server, especially when dealing with file uploads.
There may be several reasons for this issue:
- Missing Middleware
- Incorrect Content-Type Header
- Body Parsing Configuration
We will discuss the following approaches to resolve this error
Approach 1: Install and Use body-parser Middleware
If you encounter issues parsing incoming POST request data, one effective solution is to utilize the body-parser middleware in your Node/Express application. This middleware facilitates the parsing of request bodies, enabling you to extract data from incoming requests.
Run the following command to install the body parser.
npm install body-parser
Basic syntax in ExpressJS application.
Javascript
const express = require( "express" );
const bodyParser = require( "body-parser" );
const app = express();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.post( "/parse-data" , (req, res) => {
console.log(req.body);
res.send( "Data parsed successfully!" );
});
app.listen(3000, () => {
console.log( "Server is running on port 3000" );
});
|
Approach 2: Submit Data Using Raw JSON Format in Postman
If the issue is specific to Postman, consider submitting data using raw JSON format instead of form data. Postman allows you to send requests with various data formats, and opting for raw JSON can help ensure the correct interpretation of your data on the server side.
Steps:
- When making a POST request in Postman, select the raw JSON option in the request body.
- Input your data in JSON format.
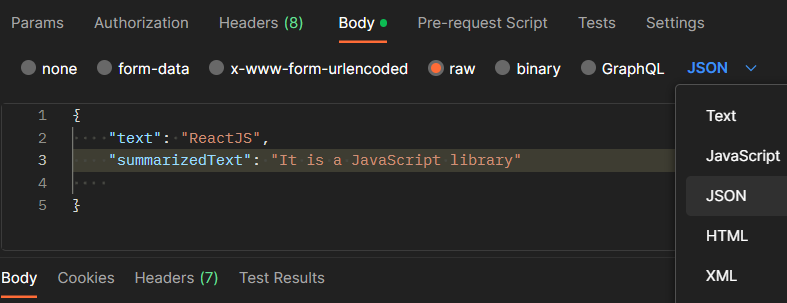
Approach 3: Verify and Use the Correct Middleware
Incorrectly choosing or configuring middleware to parse incoming request data can lead to parsing failures. Ensure that you are using the appropriate middleware for your specific use case, whether it’s for JSON or URL-encoded data.
Steps:
- Confirm the use of body-parser middleware.
- Configure it according to the data format you expect (JSON or URL-encoded).
Javascript
const express = require( "express" );
const bodyParser = require( "body-parser" );
const app = express();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.post( "/correct-middleware" , (req, res) => {
console.log(req.body);
res.send( "Data parsed with correct middleware!" );
});
app.listen(3000, () => {
console.log( "Server is running on port 3000" );
});
|
The Content-Type header in incoming requests specifies the type of data being sent. An incorrect Content-Type header can lead to parsing errors. Ensure that the header is set to either application/json or application/x-www-form-urlencoded based on the type of data being transmitted.
Steps:
- Inspect the Content-Type header in incoming requests.
- Adjust it accordingly.
Javascript
const express = require( "express" );
const bodyParser = require( "body-parser" );
const app = express();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.post( "/check-content-type" , (req, res) => {
console.log(req.body);
res.send( "Content-Type header checked successfully!" );
});
app.listen(3000, () => {
console.log( "Server is running on port 3000" );
});
|
Share your thoughts in the comments
Please Login to comment...