How to reset selected value to default using jQuery ?
Last Updated :
23 Aug, 2021
The task is to reset the value of the select element to its default value with the help of jQuery. There are two approaches that are discussed below:
Approach 1: First select the options using jQuery selectors then use prop() method to get access to its properties. If the property is selected then return the default value by using defaultSelected property.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Reset select value to
default with JQuery
</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" ></ p >
< select id = "select" >
< option value = "GFG_1" >GFG_1</ option >
< option value = "GFG_2" selected = "selected" >
GFG_2
</ option >
< option value = "GFG_3" >GFG_3</ option >
</ select >
< button onclick = "GFG_Fun()" >
Click Here
</ button >
< p id = "GFG_DOWN" ></ p >
< script >
var el_up = document.getElementById('GFG_UP');
var el_down = document.getElementById('GFG_DOWN');
el_up.innerHTML = "Select a different option "
+ "and then click on the button to "
+ "perform the operation";
function GFG_Fun() {
$('#select option').prop('selected', function () {
return this.defaultSelected;
});
el_down.innerHTML = "Default selected";
}
</ script >
</ body >
</ html >
|
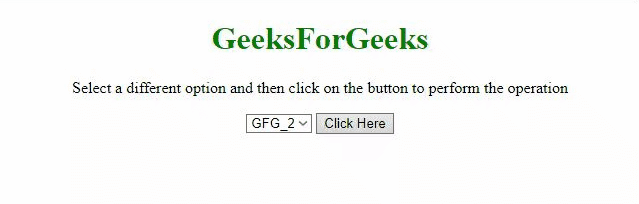
Approach 2: First, select the options using jQuery selectors then use each() method to get access to its properties. If the property is selected then return the default value by defaultSelected property.
html
<!DOCTYPE HTML>
< html >
< head >
< title >
Reset select value to
default with JQuery
</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p id = "GFG_UP" ></ p >
< select id = "select" >
< option value = "GFG_1" >GFG_1</ option >
< option value = "GFG_2" selected = "selected" >
GFG_2
</ option >
< option value = "GFG_3" >GFG_3</ option >
</ select >
< button onclick = "GFG_Fun()" >
Click Here
</ button >
< p id = "GFG_DOWN" ></ p >
< script >
var el_up = document.getElementById('GFG_UP');
var el_down = document.getElementById('GFG_DOWN');
el_up.innerHTML = "Select a different option "
+ "and then click on the button to "
+ "perform the operation";
function GFG_Fun() {
$('#select option').each(function () {
if (this.defaultSelected) {
this.selected = true;
return false;
}
});
el_down.innerHTML = "Default selected";
}
</ script >
</ body >
</ html >
|
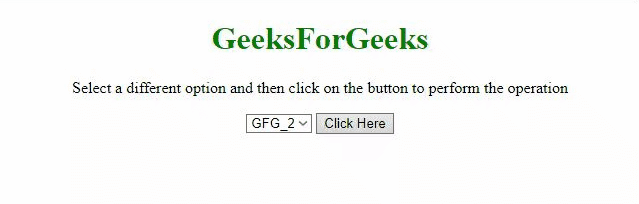
jQuery is an open source JavaScript library that simplifies the interactions between an HTML/CSS document, It is widely famous with it’s philosophy of “Write less, do more”.
You can learn jQuery from the ground up by following this jQuery Tutorial and jQuery Examples.
Share your thoughts in the comments
Please Login to comment...