How to remove a Class name from an Element using JavaScript ?
Last Updated :
09 Feb, 2024
Removing a class name from an HTML element using JavaScript is often used to dynamically modify the styling or behavior of an element based on user interactions, events, or other conditions. The operation can be achieved using classList
property, that allows you to add, remove, or toggle classes.
Syntax
paragraph.classList.remove('custom-background');
paragraph.classList.toggle('custom-background');
Using the remove( ) Method
To remove a class name from an element with JavaScript. First style the paragraph with background color. The JavaScript function removeBackgroundColor
is defined inside the <script>
block. This function is triggered when the button is clicked (onclick="removeBackgroundColor()"
). The remove()
is called on classList
to remove a specified class. It retrieves the paragraph element with the ID myParagraph
and removes the class custom-background
from it.
Example: Illustration of removing class from paragraph element.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Using remove() Method</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width,initial-scale=1.0" >
< style >
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
.card {
width: 300px;
padding: 20px;
text-align: center;
border: 3px solid #755757;
border-radius: 20px;
}
h2 {
color: #3cb369;
}
.custom-background {
background-color: #d7f1a7;
padding: 10px;
}
button {
margin-top: 10px;
padding: 8px;
cursor: pointer;
border-radius: 10px;
}
</ style >
</ head >
< body >
< div class = "card" id = "myCard" >
< h2 >GeeksforGeeks</ h2 >
< p id = "myParagraph" class = "custom-background" >
The background color will be removed
</ p >
< div >Click the button to remove the
"custom-background" class from
the < p > element
</ div >
< button onclick = "removeBackgroundColor()" >
Click to Remove Color
</ button >
</ div >
< script >
function removeBackgroundColor() {
const paragraph = document.
getElementById('myParagraph');
paragraph.classList
.remove('custom-background');
}
</ script >
</ body >
</ html >
|
Output:
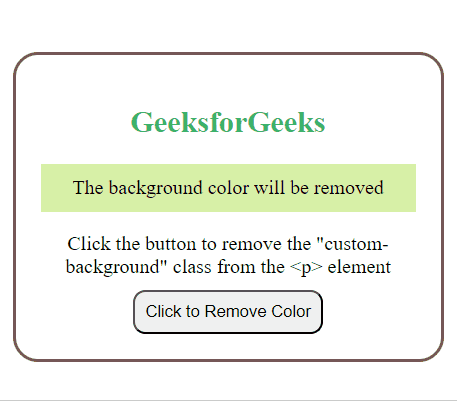
Output
Using toggle() Method
To remove a class name from an element with JavaScript. First style the paragraph with background color. The JavaScript function removeBackgroundColor
is defined inside the <script>
block. This function is triggered when the button is clicked (onclick="removeBackgroundColor()"
). The toggle()
is called on classList
to remove and add a specified class. It retrieves the paragraph element with the ID myParagraph
and removes the class if present and add if not present.
Example: Illustration of removing a class name from an element with JavaScript using toggle method.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Using toggle() Method</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width,initial-scale=1.0" >
< style >
@import url(
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
font-family: 'Poppins', sans-serif;
}
.card {
width: 300px;
padding: 20px;
text-align: center;
border: 3px solid #755757;
border-radius: 20px;
background-color: antiquewhite;
}
h2 {
color: #0e833b;
}
.custom-background {
background-color: #688338;
color: aliceblue;
padding: 10px;
}
button {
margin-top: 10px;
padding: 8px;
cursor: pointer;
border-radius: 10px;
}
</ style >
</ head >
< body >
< div class = "card" id = "myCard" >
< h2 >GeeksforGeeks</ h2 >
< p id = "myParagraph" class = "custom-background" >
The background color will be removed
</ p >
< div >Click the button to remove the
"custom-background" class from
the < p > element
</ div >
< button onclick = "removeBackgroundColor()" >
Click
</ button >
</ div >
< script >
function removeBackgroundColor() {
const paragraph = document.
getElementById('myParagraph');
paragraph.classList
.toggle('custom-background');
}
</ script >
</ body >
</ html >
|
Output:
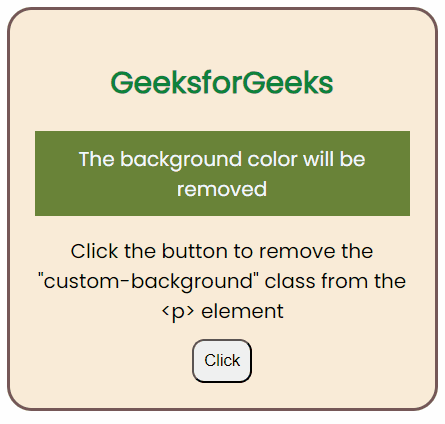
Output
Share your thoughts in the comments
Please Login to comment...