How to refresh Web Page By WebDriver When Waiting For Specific Condition?
Last Updated :
11 Oct, 2023
Selenium is an open-source program that automates web browsers. It is widely used for functional, performance, and regression testing. Selenium supports multiple programming languages, including Java, Python, C#, and Ruby, making it accessible to a wide range of developers. Here, we will be using Selenium with Python.
Prerequisites
- Selenium python package which can be installed using a pip command.
- Chrome Webdriver to use Selenium with Chrome browser and can be downloaded from its official website and extracted to a folder.
Problem Statement
There are quite a few times when we want our code to wait for some condition to be fulfilled for example, a particular element to be visible in the webpage, URL to contain a specific string, etc., and then perform an action when the condition is satisfied in this case refreshing a page.
Solution
In Selenium, we can wait for a condition to be satisfied and then perform an action.
Below three extra imports are required to achieve the same.
from selenium.webdriver.support import expected_conditions from selenium.webdriver.support.ui import WebDriverWaitfrom selenium.webdriver.common.by import By
For waiting for a specific condition, we can use the WebDriverWait function which takes arguments as the webdriver instance and the timeout value of wait in seconds. This function returns an instance of wait.
Syntax:
WebDriverWait(browser, 30)
Now, to wait for a specific condition any method can be used from the expected_conditions module in combination with the wait.until() method. One example function in the expected_conditions module is text_to_be_present_in_element_value() which takes in two arguments – a locator of the element and the text value expected in the element. The locator can be defined by using some attribute like id, class name, xpath, link text, etc. of the element.
Syntax:
wait.until(expected_conditions.text_to_be_present_in_element_value(locator, text)
After the waiting condition is satisfied, we can refresh the browser. This can be achieved by four approaches:
1.refresh() method – This method refreshes the current page.
2.sendKeys() method – This method is used for pressing some keys for some element in the webpage.
3. get() method – This method is used for opening some URL which is provided as the argument to the method.
4. Javascript Executor – This can be used to run some Javascript code on the webpage
All of these four are described and implemented below:
1. refresh() method:
This method can be directly used with the driver instance and does not take any arguments. It just refreshes the page. It is like pressing the refresh button on the browser. It does not take any arguments and does not return anything.
Syntax:
browser.refresh()
Code:
Python
from selenium import webdriver
import time
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
PATH = r "C:\Users\VRINDA\Desktop\chromedriver_win32\chromedriver.exe"
options = webdriver.ChromeOptions();
options.add_experimental_option( 'excludeSwitches' , [ 'enable-logging' ]);
try :
browser = webdriver.Chrome(PATH, options = options);
browser.get(URL);
browser.maximize_window();
wait = WebDriverWait(browser, 30 )
wait.until(expected_conditions.text_to_be_present_in_element_value((By.XPATH,
'/html/body/header/section/div/div[1]/h1[1]' ),
"I am Vrinda Ahuja." ))
browser.refresh();
time.sleep( 5 );
finally :
browser.quit()
|
Output:
.gif)
Refreshing a webpage with a refresh method
2. send_keys() method:
This method takes the argument of keys to be pressed on the keyboard for the Web element.
This method only works on a WebElement inside the webpage and not on the driver instance directly. It is basically like pressing a key on the keyboard for that element. To use this method, we need to find an appropriate element that is actionable and then provide the F5 key to the send_keys() function. This will refresh the page. Instead of using F5 directly, we can also use its ASCII value which is “\uE035”.
Syntax:
element.send_keys(Keys.F5);
Code:
Python
from selenium import webdriver
import time
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
PATH = r "C:\Users\VRINDA\Desktop\chromedriver_win32\chromedriver.exe"
options = webdriver.ChromeOptions();
options.add_experimental_option( 'excludeSwitches' , [ 'enable-logging' ]);
try :
browser = webdriver.Chrome(PATH, options = options);
browser.get(URL);
browser.maximize_window();
wait = WebDriverWait(browser, 30 )
wait.until(expected_conditions.text_to_be_present_in_element_value((By.XPATH,
'/html/body/header/section/div/div[1]/h1[1]' ),
"I am Vrinda Ahuja." ))
element = browser.find_element( "id" , "email" );
element.send_keys(Keys.F5);
time.sleep( 5 );
finally :
browser.quit()
|
Output:
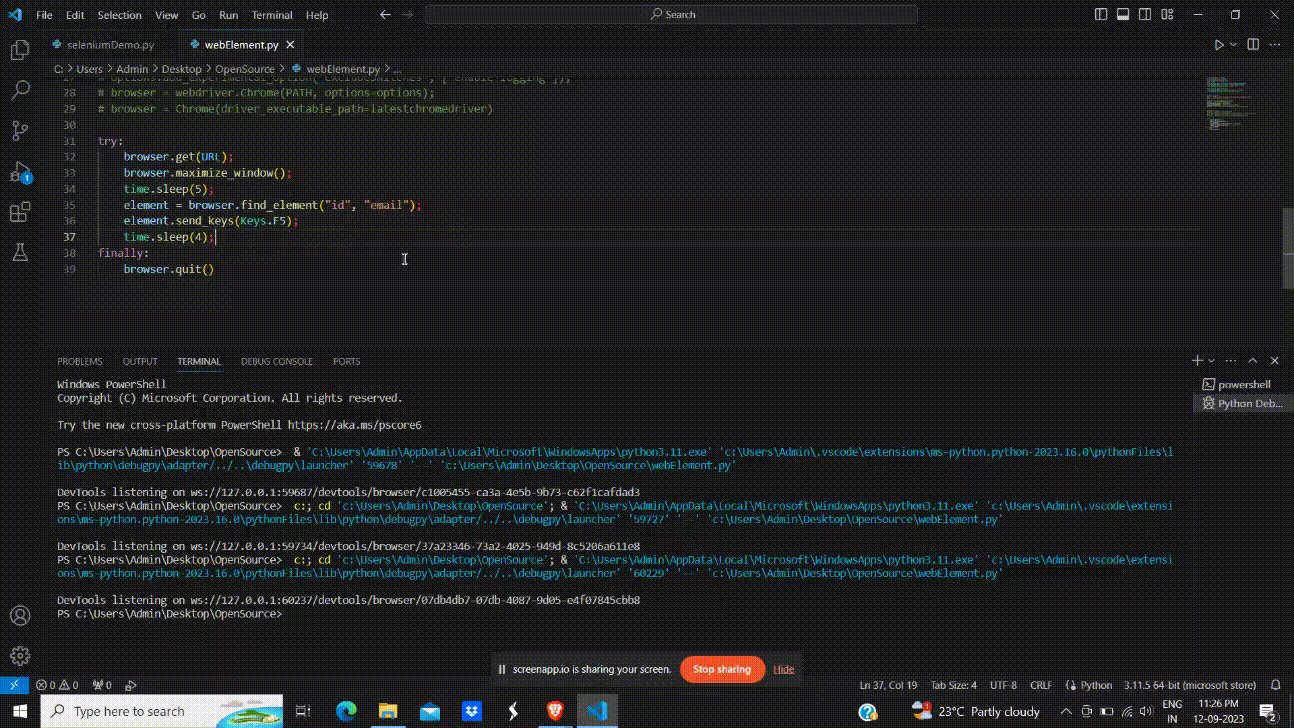
Refreshing using send keys
3. The get() method :
The get method can also be used to refresh the current page by providing the argument as the current URL of the browser. This will automatically redirect to the same page thus making an illusion of refreshing the page. This method is similar to navigating.to(url) method which is present in Java and Javascript.
Syntax:
browser.get(browser.current_url);
Code:
Python
from selenium import webdriver
import time
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
PATH = r "C:\Users\VRINDA\Desktop\chromedriver_win32\chromedriver.exe"
options = webdriver.ChromeOptions();
options.add_experimental_option( 'excludeSwitches' , [ 'enable-logging' ]);
try :
browser = webdriver.Chrome(PATH, options = options);
browser.get(URL);
browser.maximize_window();
wait = WebDriverWait(browser, 30 )
wait.until(expected_conditions.text_to_be_present_in_element_value((By.XPATH,
'/html/body/header/section/div/div[1]/h1[1]' ),
"I am Vrinda Ahuja." ))
browser.get(browser.current_url);
time.sleep( 5 );
finally :
browser.quit()
|
Output:
.gif)
Get method refresh
4. Using Javascript Executor:
We can also refresh the page by using a javascript executor and executing a script to go back to the current page by using history. go[0] as the script that is executed to go back to the page and thus refresh it.
Syntax:
browser.execute_script(‘history.go[0]’);
Code:
Python
from selenium import webdriver
import time
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
PATH = r "C:\Users\VRINDA\Desktop\chromedriver_win32\chromedriver.exe"
options = webdriver.ChromeOptions();
options.add_experimental_option( 'excludeSwitches' , [ 'enable-logging' ]);
try :
browser = webdriver.Chrome(PATH, options = options);
browser.get(URL);
browser.maximize_window();
wait = WebDriverWait(browser, 30 )
wait.until(expected_conditions.text_to_be_present_in_element_value((By.XPATH,
'/html/body/header/section/div/div[1]/h1[1]' ),
"I am Vrinda Ahuja." ))
browser.execute_script( 'history.go[0]' );
time.sleep( 5 );
finally :
browser.quit()
|
Output:
.gif)
Execute script refresh
Share your thoughts in the comments
Please Login to comment...