How to redirect browser window back using JavaScript ?
Last Updated :
15 Dec, 2023
In this article, we will redirect the browser window back using JavaScript.
There are two approaches used to redirect the browser window back:
The back() method of the window.history object is used to go back to the previous page in the current session history. In case there is no previous page, this method does not call anything. The onclick event can be specified with this method to go back one page in history.Â
Syntax:
history.back();
Example: This example uses history.back() method to redirect the browser to the previous page.Â
HTML
<!DOCTYPE html>
< html >
< head >
< title >DOM History.back() Method</ title >
< style >
h1 {
color: green;
}
body {
text-align: center;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h2 >DOM History.back() Method</ h2 >
< p >For going to the previous URL in the history,
double-click the "Go back" button: </ p >
< button onClick = "history_back()" >Go back</ button >
< script >
function history_back() {
window.history.back();
}
</ script >
</ body >
</ html >
|
Output:
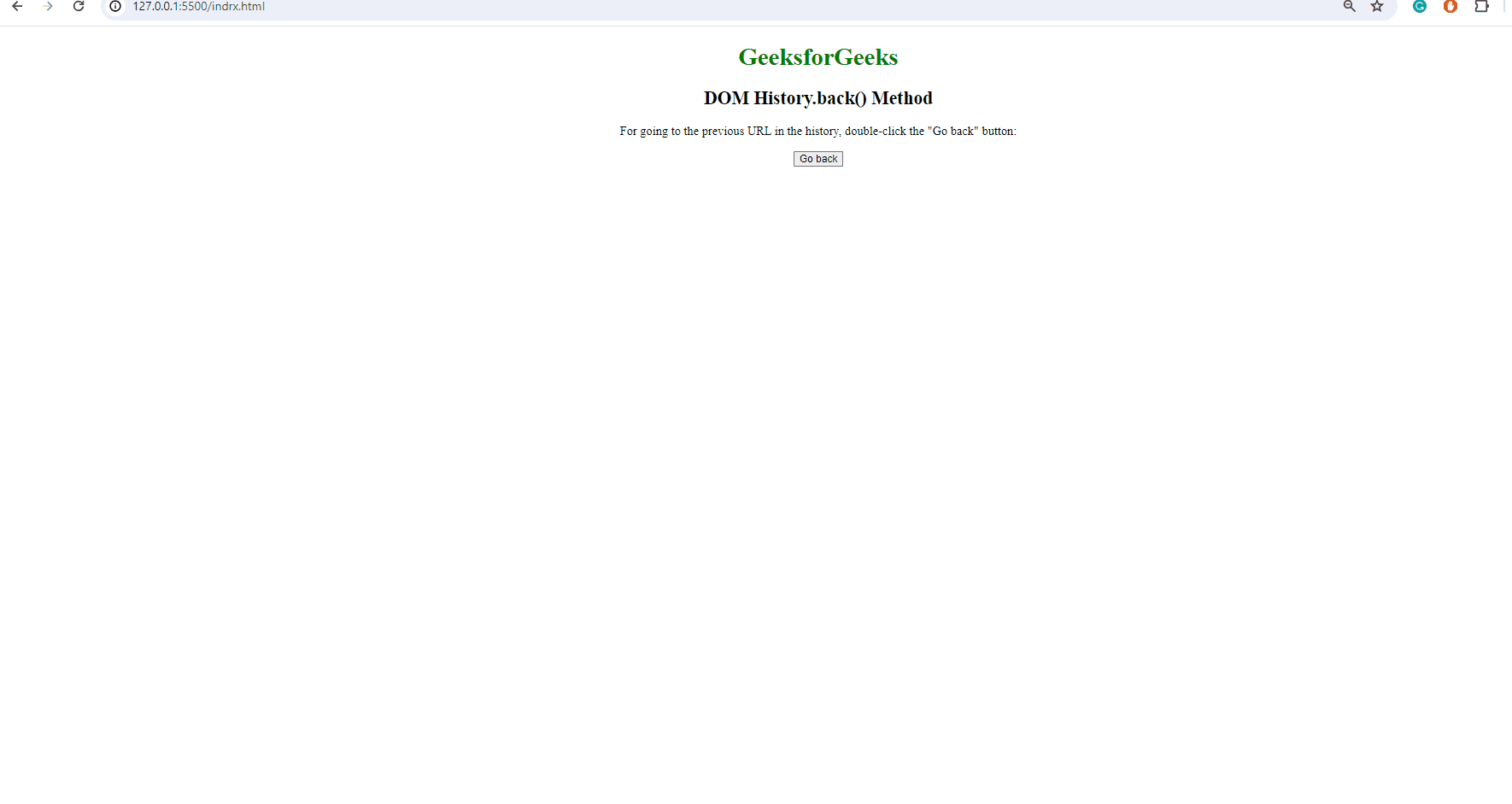
Output
The history.go() method of the window.history object is used to load a page from the session history. It can be used to move forward or backward using the value of the delta parameter. A positive delta parameter means that the page would go forward in history. Similarly, a negative delta value would make the page go back to the previous page. This method can be used with ‘-1’ as the delta value to go back one page in history. The onclick event can be specified with the method to go back one page in history.Â
Syntax:
history.go(number\URL);
Example: This example uses window.history.go() method to redirect the browser into previous page.Â
HTML
<!DOCTYPE html>
< html >
< head >
< title >DOM History.go() Method</ title >
< style >
h1 {
color: green;
}
body {
text-align: center;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< h2 >DOM History.go() Method</ h2 >
< p >
For going to the previous URL in the
history, double click the "Go to the
previous URL" button:
</ p >
< button onClick = "history_goback()" >
Go to the previous URL
</ button >
< script >
function history_goback() {
window.history.go(-1);
}
</ script >
</ body >
</ html >
|
Output:
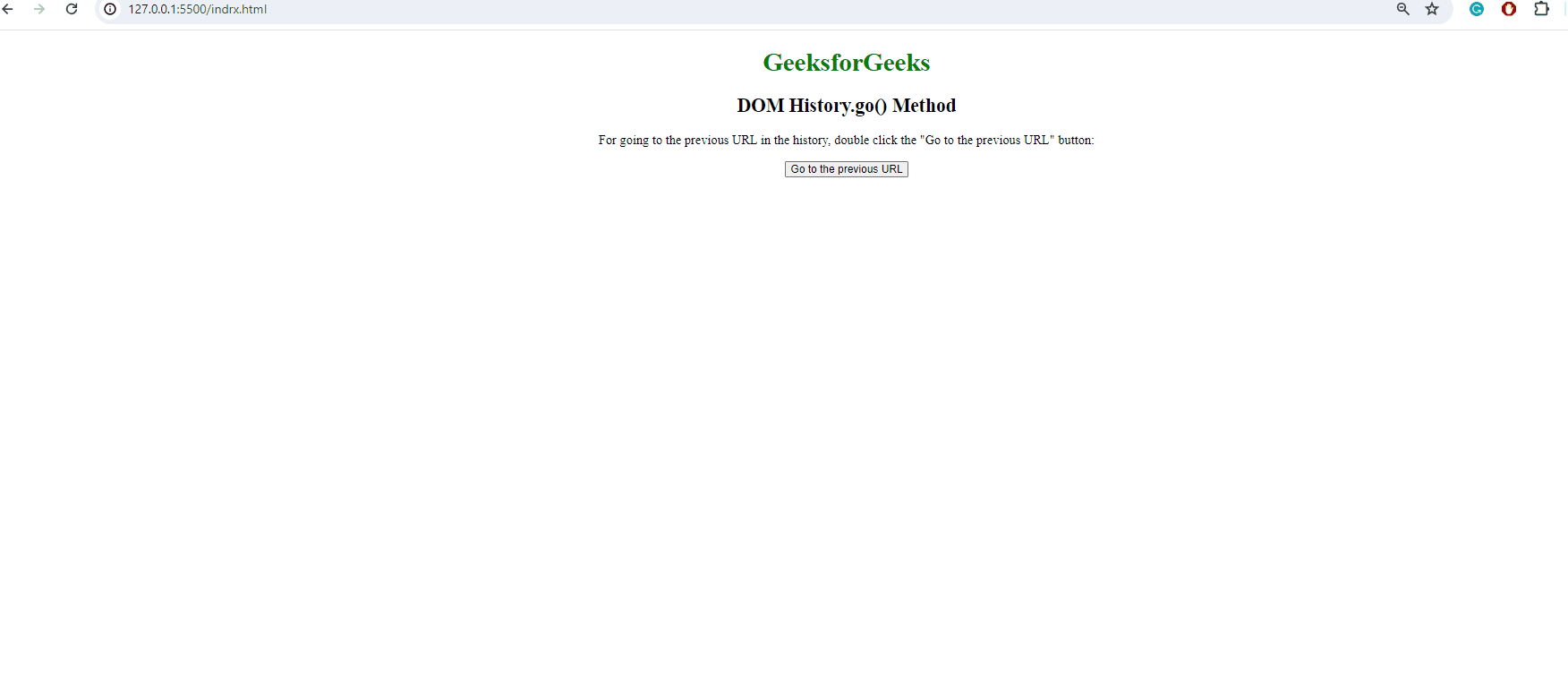
Note: This method will not work if the previous page does not exist in the history list.Â
Supported Browsers:Â
- Google Chrome
- Microsoft Edge
- Firefox
- Apple Safari
- Opera
Share your thoughts in the comments
Please Login to comment...