How to prevent the Common Vulnerabilities in JavaScript ?
Last Updated :
16 Oct, 2023
In this article, we will see the Preventing Common Vulnerabilities in JavaScript. Before we proceed, we will first understand the list of most common Vulnerability attacks, & then will understand the various approaches to resolve those Vulnerability attacks. Finally, we will understand the concept with the help of basic illustrations.
List of Common Vulnerabilities
The following is the list of the most common Vulnerabilities:
- Cross-Site Scripting (XSS): The XSS is a vulnerability that allows attackers to inject malicious scripts into the web applications which are then executed by other users.
- Cross-Site Request Forgery (CSRF): The CSRF is an attack where an attacker tricks a user into performing actions on a different site without their knowledge or consent.
- Input Validation and Sanitization: This involves validating and cleaning user inputs to prevent the execution of malicious code or unexpected behavior.
- SQL Injection: The Attackers manipulate input to execute malicious SQL queries on a database.
- Insecure Deserialization: The Attackers exploit weaknesses in the deserialization to execute malicious code.
- Authentication and Session Management Issues: The Weaknesses in authentication and session management can lead to unauthorized access.
List of Preventive Approaches
- Cross-Site Scripting (XSS) Prevention
- Cross-Site Request Forgery (CSRF)
- Input Validation and Sanitization
Cross-Site Scripting (XSS) Prevention
The XSS occurs when an attacker injects malicious scripts into a web application which are then executed in a user’s browser. This can lead to stealing sensitive data or compromising user sessions. The preventive approach is to Sanitize user inputs and escape output using the appropriate encoding functions. A detailed description of prevention is covered in Cross Site Scripting (XSS) Prevention Techniques article.
Example: This example illustrates the prevention from Cross-Site Scripting (XSS) in JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >The Prevention Example</ title >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Implementing the Cross-Site
Scripting (XSS) Prevention
</ h3 >
< label for = "userInput" >
Enter your input:
</ label >
< input type = "text" id = "userInput" >
< button onclick = "GFG()" >
Display
</ button >
< script >
function sanitizeInput(input) {
return input.replace(/</ g , '<')
.replace(/>/g, '>');
}
function GFG() {
const userInput = document
.getElementById('userInput').value;
const sanitizedInput = sanitizeInput(userInput);
alert('Sanitized Input: ' + sanitizedInput);
}
</ script >
</ body >
</ html >
|
Output:
Cross-Site Request Forgery (CSRF)
The CSRF involves tricking a user into performing unwanted actions without their consent and This happens when an attacker uses a user’s authenticated session to perform the actions on a different site. The preventive approach is to implement CSRF tokens in forms to validate the authenticity of the requests. Use SameSite cookies to restrict cookies from being sent in the cross-origin requests. Please refer to the Cross-Site Request Forgery (CSRF) Protection Methods and Bypasses Article for a detailed description.
Example: This example illustrates the basic implementation of the Cross-Site Request Forgery (CSRF).
HTML
<!DOCTYPE html>
< html >
< head >
< title >
The CSRF Prevention
</ title >
< script >
function GFG() {
const xhr = new XMLHttpRequest();
xhr.open("POST", "process.php", true);
const csrfToken = "your_csrf_token_here";
xhr.setRequestHeader("X-CSRF-Token", csrfToken);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
document.getElementById("output")
.textContent =
"Request processed successfully!";
} else {
document.getElementById("output")
.textContent =
"Error processing request.";
}
}
};
xhr.send();
}
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Implementing the Cross-Site Request Forgery (CSRF)
</ h3 >
< button onclick = "GFG()" >
Submit
</ button >
< div id = "output" ></ div >
</ body >
</ html >
|
Output:
In this approach, we’ll be using Regular Expressions to validate and sanitize input. Regular Expression has a sequence of characters that forms a search pattern. The search pattern can be used for text search and text to replace operations. In the case of Sanitization, it takes the help npm package that can be used to sanitize file names by removing all the unwanted characters or white spaces from the file name and making it a proper file name according to the specific file system or operating system.
Example: This example illustrates the basic implementation of Input Validation and Sanitization.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
The Input Validation and Sanitization
</ title >
< script >
function validate(input) {
return /^[a-zA-Z0-9]+$/.test(input);
}
function sanitize(input) {
return input.replace(/</ g , "<")
.replace(/>/g, ">");
}
function process() {
const userInput = document
.getElementById("userInput").value;
if (validate(userInput)) {
const sanitizedInput = sanitize(userInput);
document.getElementById("output")
.innerHTML = sanitizedInput;
} else {
alert("Invalid input! Only alphanumeric"+
" characters are allowed.");
}
}
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Implementing the Input
Validation and Sanitization
</ h3 >
< input type = "text"
id = "userInput"
placeholder = "Enter The alphanumeric input" >
< button onclick = "process()" >
Process
</ button >
< div id = "output" ></ div >
</ body >
</ html >
|
Output:
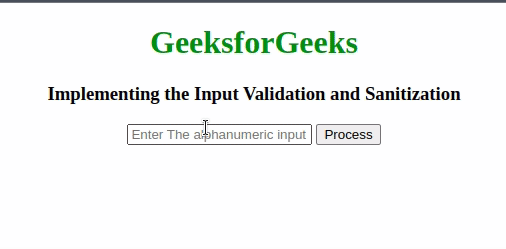
Conclusion
Securing JavaScript applications is paramount to prevent common vulnerabilities and Cross-site scripting (XSS) cross-site request forgery (CSRF) inadequate input validation and other vulnerabilities can have serious consequences. By implementing proper sanitization input validation using the security tokens and following secure coding practices developers can significantly reduce the risk of their applications being exploited.
Share your thoughts in the comments
Please Login to comment...