How to Write to a File Using Applet?
Last Updated :
24 Oct, 2023
In this article, we will learn how to write a file using Applet and grant access to write files using Applet.
Approach
The applet output window contains three fields. Those are the file name field, Text area field, and save button field.
- File name field: It asks the user to enter the file name to write content in the file.
- Text area: It is an input field where the content of the file can be entered.
- Save button: It is an event listener. On clicking the save button, it will call the function that contains the logic to write the file.
Important Note:
- Latest java version doesn’t support applet. so, it needs older version of java which supports applet to be installed.
- Latest browser will not permit applet application to write files. So, we need to add .policy file with permission granting instructions.
Steps to Run the Program
There are certain Steps to execute the program as mentioned below:
Step 1: Create a policy file with the file name “FileWriterApplet.policy” and add the below code to grant access for writing the file.
FileWriterApplet.policy
grant {
permission java.io.FilePermission "<<ALL FILES>>","write";
};
Step 2: Create an HTML file and replace it with the below code.
FileWriterApplet.html
HTML
< HTML >
< HEAD >
< TITLE >File Writer Applet</ TITLE >
< applet code = "FileWriterApplet.class" width = "400" height = "300" >
</ applet >
</ HEAD >
</ HTML >
|
Step 3: Create FileWriterApplet.java with below code which contains main logic to write the file.
Program for writing to a file using Applet.
Below is the implementaion of the above method:
Java
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class FileWriterApplet extends Applet {
private TextField fileNameField;
private TextArea textArea;
private Button saveButton;
public void init() {
setLayout( new BorderLayout());
fileNameField = new TextField( 20 );
textArea = new TextArea( 10 , 40 );
saveButton = new Button( "Save" );
Panel inputPanel = new Panel();
inputPanel.add( new Label( "File Name:" ));
inputPanel.add(fileNameField);
add(inputPanel, BorderLayout.NORTH);
add(textArea, BorderLayout.CENTER);
add(saveButton, BorderLayout.SOUTH);
saveButton.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e) {
saveToFile();
}
});
}
private void saveToFile() {
String fileName = fileNameField.getText();
String content = textArea.getText();
try {
FileWriter writer = new FileWriter(fileName);
writer.write(content);
writer.close();
System.out.println( "File saved successfully!" );
} catch (IOException e) {
System.out.println( "Error: " + e.getMessage());
}
}
}
|
Executing the Program

run commands to start applet
Output
Step 1: Enter the file name in the file name field.
Step 2: Write content in the text field.
Step 3. Click on save button to write the file.
Applet Screen
After exectuing the commands in the command line this applet screen will occur.
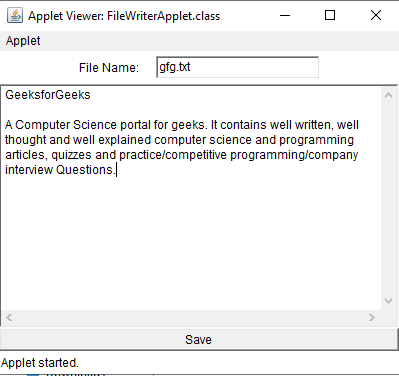
FIle Writer Applet output
Final Result
The below image shows the file written by the Applet File Writer.
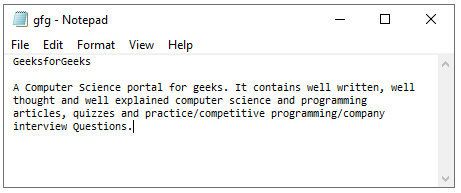
Written file
Share your thoughts in the comments
Please Login to comment...