How to move an array element from one array position to another in JavaScript?
Last Updated :
13 Dec, 2023
In JavaScript, we can access an array element as in other programming languages like C, C++, Java, etc. Also, there is a method called splice() in JavaScript, by which an array can be removed or replaced by another element for an index. So to move an array element from one array position to another we can splice() method or we can simply use array indexing ([]).Â
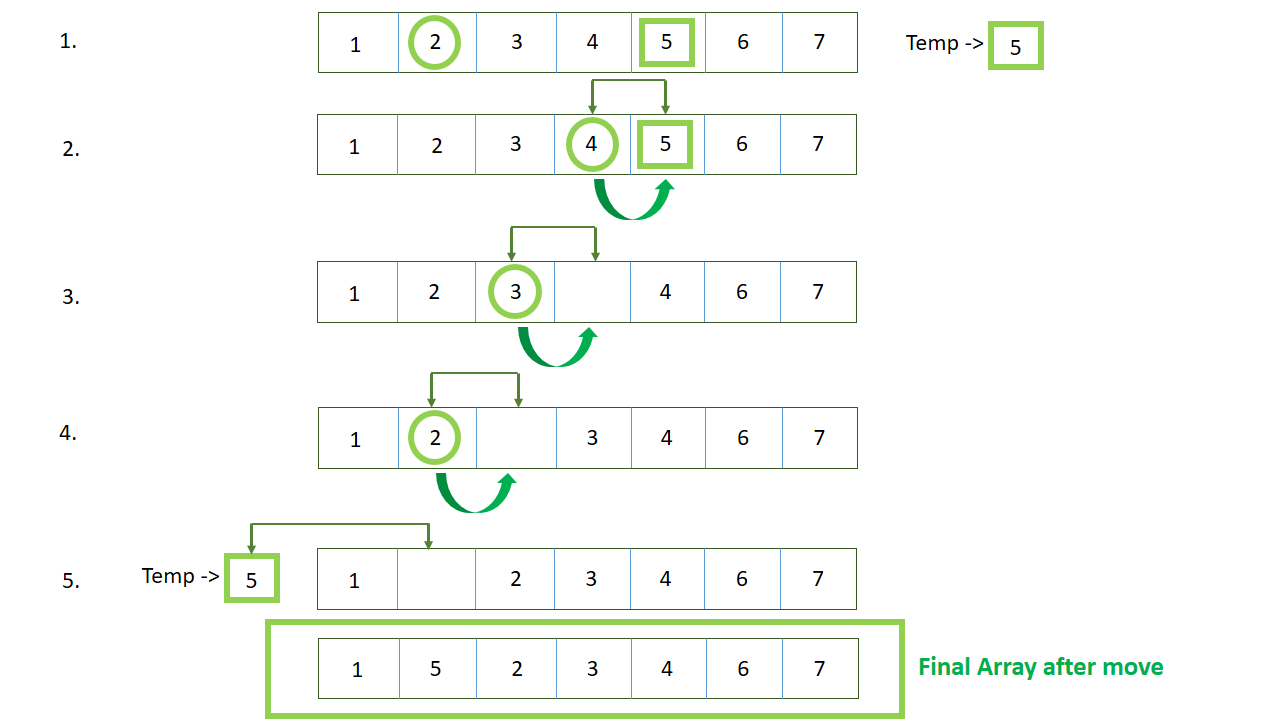
These are the following ways to solve this problem:
Using for loop
In this approach, we are using a for loop for moving an array element from one position to another one.
Example: This example shows the implementation of the above-explained approach.
javascript
let arr = [ "C++" , "Java" , "JS" , "Python" ];
console.log( "Original array: " + arr);
let x = 3;
let pos = 1;
let temp = arr[x];
let i;
for (i = x; i >= pos; i--) {
arr[i] = arr[i - 1];
}
arr[pos] = temp;
console.log( "After move: " + arr);
|
Output
Original array: C++,Java,JS,Python
After move: C++,Python,Java,JS
Using splice() function
In this approach, we are using splice() function. If the given posiiton is less then zero then we will move that element at the end of the array. If it is greater than 0 then we will move the element in between the array, that is how we move our element according to the index.
Example: This example shows the implementation of the above-explained approach.
javascript
let arr = [ "C++ " , "Java " , "JS " ,
"Ruby " , "Python " ];
console.log( "Original array: " + arr);
let moveEle = 3;
let moveToIndx = 1;
while (moveEle < 0) {
moveEle += arr.length;
}
while (moveToIndx < 0) {
moveToIndx = moveToIndx + arr.length;
}
if (moveToIndx >= arr.length) {
let un = moveToIndx - arr.length + 1;
while (un--) {
arr.push(undefined);
}
}
arr.splice(moveToIndx, 0, arr.splice(moveEle, 1));
console.log( "After move: " + arr);
|
Output
Original array: C++ ,Java ,JS ,Ruby ,Python
After move: C++ ,Ruby ,Java ,JS ,Python
Using slice(), concat(), and spread operator
In this approach, we are using slice(), concat(), and spread operator. we are craeting the array from the index 0 to the index where that element(that will be moved) is present and the inserted new element and the remaining elements.
Example: In this example, we will use slice(), concat(), and spread operator.
Javascript
const arr = [1, 2, 8, 4, 5];
const toMove = arr[2];
const newArr = [
...arr.slice(0, 2),
...arr.slice(3),
toMove
];
console.log(newArr);
|
Share your thoughts in the comments
Please Login to comment...