Add Elements to a JavaScript Array
Last Updated :
24 Jan, 2024
JavaScript Arrays are a fundamental data structure in JavaScript, providing a flexible way to store and manage collections of values. Add elements to a JavaScript Array is a common operation in programming. There are different methods to add elements to the JavaScript array, which are described below
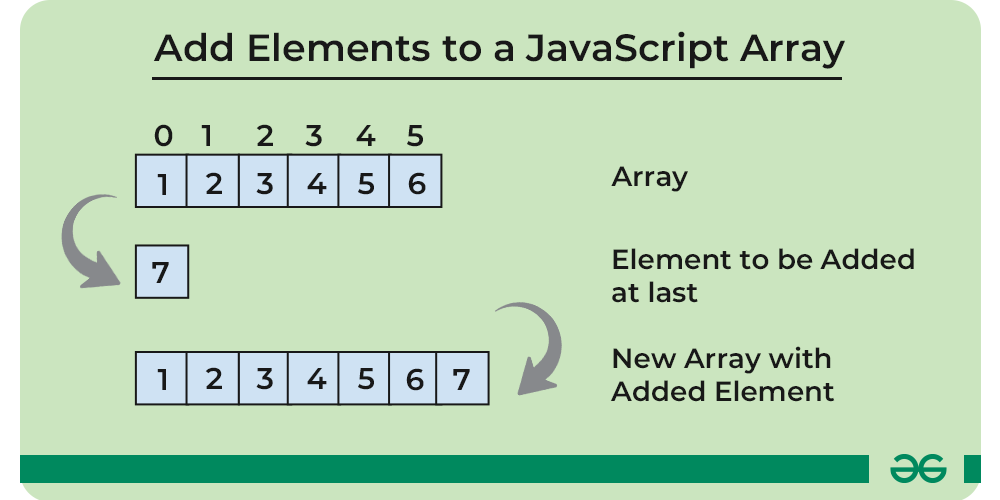
Methods to Add Elements to a JavaScript Array
Following are the methods that can be used to add elements to a JavaScript array:
Method 1: Using Push() Method
The push()
method is used to add one or more elements to the end of an array.
Example: The push()
method adds multiple elements at the end of the array.
Javascript
const myArray = [1, 2, 3];
myArray.push(4);
console.log(myArray);
myArray.push(5, 6);
console.log(myArray);
|
Output
[ 1, 2, 3, 4 ]
[ 1, 2, 3, 4, 5, 6 ]
Method 2: Using concat() Method
The concat() method
is used to create a new array by combining existing arrays with additional elements.
Example: The below code implements the concat method to add elements to an JavaScript array.
Javascript
const myArray1 = [ 'JavaScript' , 'GeeksforGeeks' ];
const myArray2 = [ 'TypeScript' , 'ReactJs' , 'Angular' ];
const newArray = myArray1.concat(myArray2);
console.log(newArray);
|
Output
[ 'JavaScript', 'GeeksforGeeks', 'TypeScript', 'ReactJs', 'Angular' ]
Method 3: Using Splice() Method
The
splice() method
is used to add or remove elements from a specific index in an array. We need to set the second argument of the splice method to 0 for inserting elements into an array using it.
Example: The below example shows the use of the splice method to add elements to an array.
Javascript
const myArray = [1, 2, 3];
myArray.splice(1, 0, 4);
console.log(myArray);
|
Method 4: Using Spread Operator
The Spread Operator is used to extend an array and create a new array with new elements.
Example: The below example illustrates the use of spread operator to add elements to an array.
Javascript
const myArray = [1, 2, 3];
const newArray = [...myArray, 4, 5];
console.log(newArray)
|
Method 5: Using Unshift() Method
The unshift()
method is used to add one or more elements to the beginning of an array.
Example: This code example uses unshift() method to add the elements into an array.
Javascript
const myArray = [4, 5, 6];
myArray.unshift(3);
console.log(myArray);
myArray.unshift(1, 2);
console.log(myArray);
|
Output
[ 3, 4, 5, 6 ]
[ 1, 2, 3, 4, 5, 6 ]
Method 6: Using Length Property
The array length property is used to set or return the number of elements in an array. This property can also be used to add elements at the end of the array.
Example: This example shows how to use the length property to add elements at the end of the array in JavaScript.
Javascript
const myArray = [1, 2, 3];
console.log(myArray.length);
myArray[myArray.length] = 4;
console.log(myArray);
console.log(myArray.length);
|
Output
3
[ 1, 2, 3, 4 ]
4
Method 7: Using Index Assignment
You can directly assign values to specific indices to add elements to the JavaScript array.
Example: This example describes how to use index number to add elements to the JavaScript Array.
Javascript
const myArray = [1, 2, 3];
myArray[3] = 4;
console.log(myArray);
|
Share your thoughts in the comments
Please Login to comment...