How to Manage startActivityForResult on Android?
Last Updated :
23 Feb, 2023
StartActivityForResult is a powerful method in Android that allows one activity to start another activity and receive a result back. This result can be used to perform an action in the original activity, such as updating its UI or making a network request. While it’s a useful tool, it can be difficult to manage StartActivityForResult properly, especially in complex applications with multiple activities. In this Geeks for Geeks article we will discuss the basics of StartActivityForResult and provide tips on how to effectively manage it.
When is startActivity called?
The StartActivityForResult method is called on an Activity instance and takes two parameters: the Intent that represents the activity to start, and a request code. The request code is an integer value that the original activity uses to identify the result from the started activity. When the started activity finishes, it sets a result using the setResult method and the original activity receives this result in its onActivityResult method. The onActivityResult method takes three parameters: the request code, a result code, and an Intent containing the data returned by the started activity.
Here’s an example of how StartActivityForResult can be used in an Android activity. Let’s say you have an activity named MainActivity that needs to start another activity, SecondActivity, to get a result. In MainActivity, you can call StartActivityForResult like this:
Java
Intent gfgIntent
= new Intent(SplashScreen. this , gfgLanding. class );
startActivityForResult(intent, "101" );
|
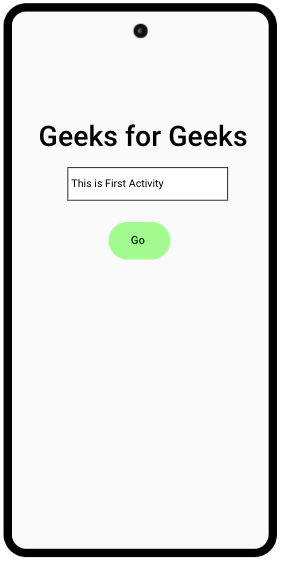
Image #1: The first activity.
Analyzing the Result in the Next Activity
In SecondActivity, you can set the result using setResult like this:
Java
Intent gfgResultAnalyze = new Intent();
result.putExtra( "activity_result" , "The outcome of the second activity" );
finish();
|
In this example, the requestCode and resultCode are checked to make sure that the result is from the correct activity and that the result is successful. Then, the data is retrieved from the Intent and used to update the MainActivity UI or perform another action.
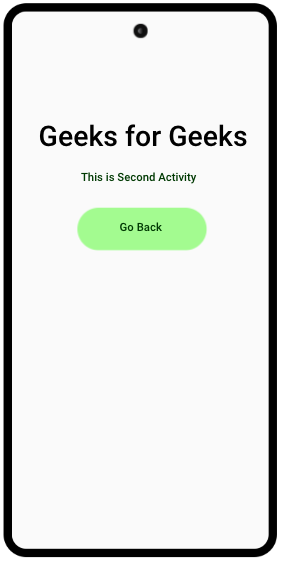
Image #2: The second activity.
Tips to manage startActivityForResult effectively
- Use a constant for the request code: It’s important to use a constant for the request code instead of a hardcoded value. This makes the code more readable and reduces the risk of using the same request code for different activities.
- Use unique request codes: Make sure to use unique request codes for each activity that you start using StartActivityForResult. This will prevent confusion and ensure that the correct result is received in the original activity.
- Check the request code: Always make sure to check the request code in the onActivityResult method to ensure that the result is from the correct activity. This can be done by comparing the request code passed to the StartActivityForResult method with the request code received in onActivityResult.
- Manage state: It’s important to manage the state of the original activity when it receives the result from the started activity. This may involve updating the UI, making a network request, or saving the data to a database.
- Use startActivityForResult for critical actions: StartActivityForResult is a powerful method that should be used for critical actions, such as taking a photo or choosing a file. This allows the original activity to receive the result and take appropriate action.
- Handle errors: It’s important to handle errors in the started activity and set an appropriate result code. This will allow the original activity to handle the error and take appropriate action.
- Use Intent extras: You can use the Intent extras to pass data to the started activity and receive data back in the original activity. This is a convenient way to pass data between activities and can be used to store data that is specific to the current session.
- Store data in the Application class: In complex applications, it can be useful to store data in the Application class instead of using Intent extras. This makes the data accessible from any activity and reduces the risk of data loss if the activity is destroyed.
Conclusion
In conclusion, StartActivityForResult is a powerful method in Android that allows one activity to start another activity and receive a result back. Hope this article helped you to learn how to manage your activities effectively, you can effectively manage StartActivityForResult and use it to create a more dynamic and flexible user experience in your Android applications.
Starting an activity is an expensive operation, and you should minimize the number of times you start an activity using StartActivityForResult. You can also use caching to improve the performance of your application by storing data that is frequently used.
Share your thoughts in the comments
Please Login to comment...