How to Manage Service Worker Cache in JavaScript?
Last Updated :
08 May, 2024
A service worker’s ability to cache resources is crucial for a performant and reliable web application. By storing frequently accessed files like HTML, JavaScript, and images, you can enable offline functionality and faster loading times.
Below are the approaches to manage service worker cache in JavaScript:
Caching Responses from Network Fetches
This approach uses the Cache.add(url) method to fetch a resource from the network and store the response in the cache and it is also simpler and ideal for frequently accessed static assets.
Steps:
- Open a Cache: Use caches.open(‘cacheName’) to open a named cache. This establishes a connection with a specific cache storage area.
- Add a URL to the Cache: Use .then(cache => cache.add(url)) within the caches.open promise chain. This fetches the resource at the specified URL and stores the response in the opened cache.
Example: To demonstrate caching ‘index.html’ with Service Worker in HTML
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style></style>
</head>
<body>
<h1 style="text-align: center;">Welcome to GFG</h1>
<script>
caches.open('my-static-cache')
.then(cache => {
cache.add('/index.html');
})
.catch(error => {
console.error('Cache opening error:', error);
});
</script>
</body>
</html>
Output: The browser console should ideally log no errors (Cache opening error:) if the cache is opened successfully. The requested resource (/index.html in this case) will be stored in the my-static-cache for future use.
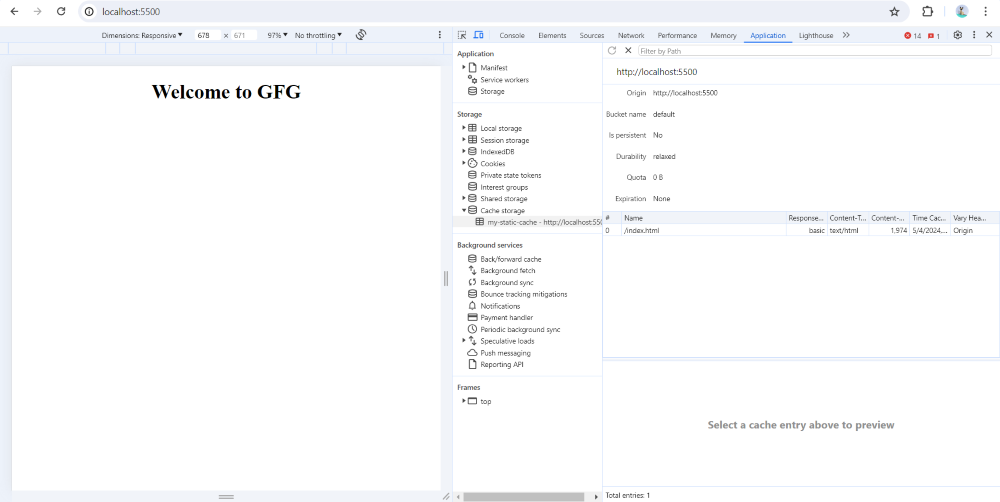
Output
Custom Caching with Request-Response Pairs
This approach provides finer control by letting you specify request-response pairs for caching and offers more control for caching specific interactions or dynamic content.
Steps:
- Create a Request Object: Use const request = new Request(url) to create a request object for the desired resource URL.
- Fetch the Resource: Use fetch(request) to fetch the resource from the network.
- Store the Request-Response Pair in Cache: Use .then(response => cache.put(request, response)) within the fetch promise chain. This stores the created request object (request) and the fetched response (response) together in the cache.
Example: To demonstrate caching ‘data.json’ with Service Worker in HTML.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style></style>
</head>
<body>
<h1 style="text-align: center;">Welcome to GFG</h1>
<script>
const request = new Request('/data.json');
fetch(request)
.then(response => {
return caches.open('my-data-cache').then(cache => {
return cache.put(request, response);
});
})
.catch(error => {
console.error('Fetching or caching error:', error);
});
</script>
</body>
</html>
Output: Similar to the previous approach, the browser console should ideally log no errors. The request (/data.json) and its corresponding response will be stored in the my-data-cache for future use.
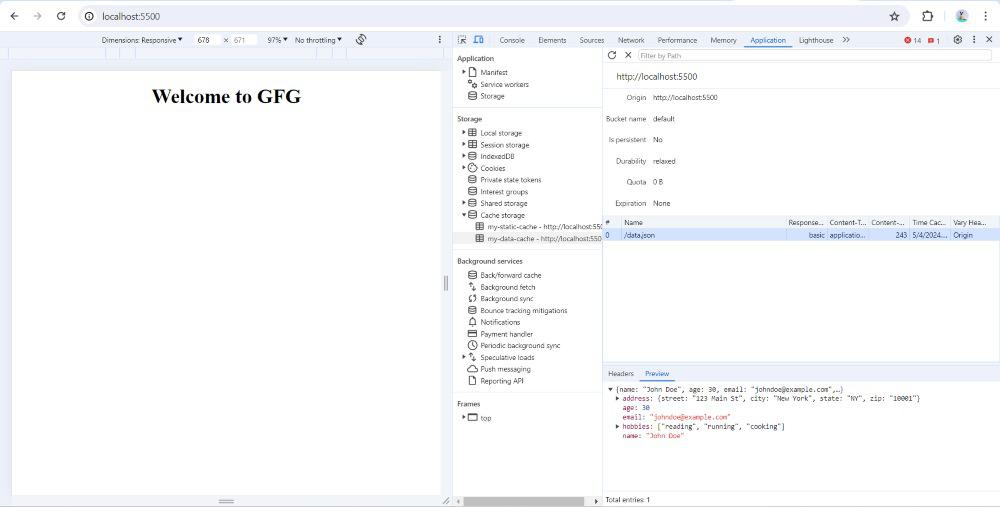
Output
Share your thoughts in the comments
Please Login to comment...