How to Make Scrollable Drop Down Lists in React-Bootstrap ?
Last Updated :
19 Oct, 2023
In this article, we will learn how to make Scrollable Dropdown lists in React-Bootstrap. Drop-down lists allow users to choose an option from a list of available options visible whenever the corresponding component is clicked or triggered. Using React-bootstrap, we can customize the drop-down list as needed.
Syntax:
<Dropdown>
<Dropdown.Toggle>...</Dropdown.Toggle>
<Dropdown.Menu>
<Dropdown.Item>
<Dropdown.Item>
</Dropdown.Item>
</Dropdown.menu>
</Dropdown>
Steps to create the application:
Step 1: Create the react app using the following CRA command.
npx create-react-app appdemo
Step 2: Now, navigate to your current project i.e. appdemo using the following command.
cd appdemo
Step 3: Install the required modules i.e. react-bootstrap and bootstrap using the following command
npm install react-bootstrap bootstrap
Step 4: Import the necessary components from react-bootstrap as per requirement. To demonstrate a scrollable dropdown list. I am importing Bootstrap CSS and Dropdown components from react-bootstrap.
import React from "react";
import Dropdown from "react-bootstrap/Dropdown";
Project Structure:
The project structure look like the following
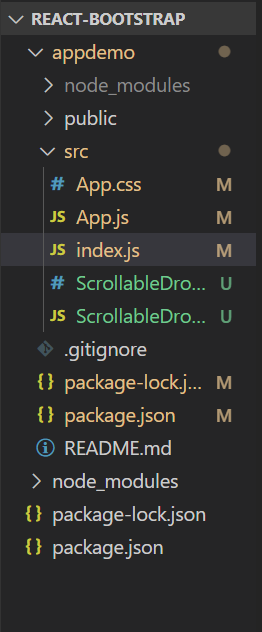
Project Structure
package.json:
{
"name": "appdemo",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.8.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
}
Approach
We will use react-bootstrap dropdown component to create the scrollable dropdown list as shown below.
Example: Let us create a dropdown list component using React-bootstrap
Javascript
import React from 'react' ;
import ScrollableDropdown from './ScrollableDropdown' ;
import './App.css' ;
const App = () => {
return (
<div class= "component" >
<ScrollableDropdown />
</div>
);
};
export default App;
|
Javascript
import React from "react" ;
import Dropdown from "react-bootstrap/Dropdown" ;
import "./ScrollableDropdown.css" ;
const ScrollableDropdown = () => {
return (
<Dropdown>
<h4 class= "subtitle" >
What is your specialization?
</h4>
<Dropdown.Toggle
variant= "success"
id= "dropdown-basic"
>
select from here
</Dropdown.Toggle>
<Dropdown.Menu
style={{
maxHeight: "200px" ,
overflowY: "auto" ,
}}>
<Dropdown.Item href= "#" >CSE</Dropdown.Item>
<Dropdown.Item href= "#" >IT</Dropdown.Item>
<Dropdown.Item href= "#" >
AI & DS
</Dropdown.Item>
<Dropdown.Item href= "#" >
AI & ML
</Dropdown.Item>
<Dropdown.Item href= "#" >EEE</Dropdown.Item>
<Dropdown.Item href= "#" >ECE</Dropdown.Item>
<Dropdown.Item href= "#" >
CIVIL
</Dropdown.Item>
<Dropdown.Item href= "#" >MECH</Dropdown.Item>
</Dropdown.Menu>
</Dropdown>
);
};
export default ScrollableDropdown;
|
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import 'bootstrap/dist/css/bootstrap.min.css' ;
import App from './App' ;
ReactDOM.render(<App />, document.getElementById( 'root' ));
|
CSS
.subtitle{
margin-bottom : 30px
}
|
CSS
.component {
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
height : 100 vh;
}
|
Explanation: In the above code, we created a functional component called ScrollableDropdown in which we are using the Dropdown component which is imported from React-bootstrap.
The Dropdown component comprises of various other components as follows:
- Dropdown.Toggle: This is used to trigger the element for dropdown containing the text “select from here”.
- Dropdowm.Menu: This is used to display the dropdown menu and styled to make the dropdown menu scrollable with
- Dropdown.Item: This is included in the Dropdown.Menu and used to represent the individual options/choices in the dropdown list to choose.
- We can add more customization to our component using CSS.
Steps to run the application:
To start the application. use the commands:
npm start
Output:
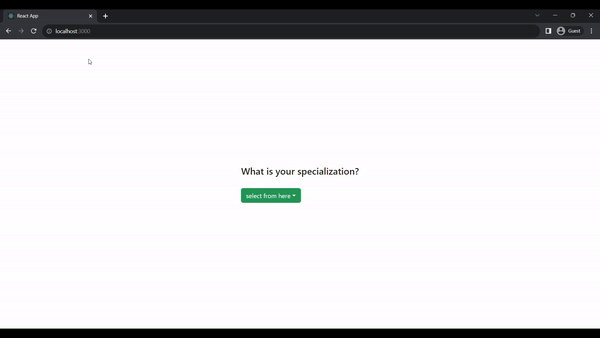
Output
Share your thoughts in the comments
Please Login to comment...