How to Load a File into the Python Console
Last Updated :
27 Feb, 2024
Loading files into the Python console is a fundamental skill for any Python programmer, enabling the manipulation and analysis of diverse data formats. In this article, we’ll explore how to load four common file types—text, JSON, CSV, and HTML—into the Python console. Whether you’re dealing with raw text, structured JSON data, tabular CSV files, or extracting information from HTML, understanding these techniques will enhance your ability to work with a wide range of data sources in Python.
Load a File into the Python Console
below, is the step-by-step guide to How To Load A File into the Python Console:
Create a Virtual Environment
First, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
File Structure
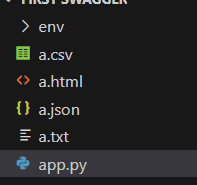
File Structure
Example 1: Load .txt File
In this example, below code opens a text file named ‘a.txt’ in read mode, reads its entire content, and stores it in the variable `text_content`. Finally, it prints the content to the console. The use of the ‘with’ statement ensures proper file closure after reading.
a.txt
GeeksforGeeks
Python3
with open ( 'a.txt' , 'r' ) as file :
text_content = file .read()
print (text_content)
|
Output:
GeeksforGeeks
Example 2: Load .CSV File
In this example, below code utilizes the `CSV’ Module to open a CSV file (‘a.csv’) in read mode. It creates a CSV reader object, `csv_reader`, and iterates through its rows, printing each row to the console. The ‘with’ statement ensures proper file closure after reading.
a.csv
['Name GeeksforGeeks ']
Python3
import csv
with open ( 'a.csv' , 'r' ) as file :
csv_reader = csv.reader( file )
for row in csv_reader:
print (row)
|
Output:
['Name GeeksforGeeks ']
Example 3: Load .json File
In this example, code uses the `json` module to open a JSON file (‘a.json’) in read mode. It loads the JSON content into a Python dictionary using `json.load()`. Finally, it prints the dictionary representing the JSON content to the console. The ‘with’ statement ensures proper file closure after reading.
a.json
{"GeeksforGeeks": "1st Rank" }
Python3
import json
with open ( 'a.json' , 'r' ) as file :
json_content = json.load( file )
print (json_content)
|
Output:
{ "GeeksforGeeks": "1st Rank" }
Example 4 : Load .html File
In this example, below code utilizes the `BeautifulSoup` library to parse an HTML file (‘a.html’) in read mode. It creates a BeautifulSoup object, `soup`, using the ‘html.parser‘. It then extracts and prints specific elements such as the title and body from the HTML file. The ‘with’ statement ensures proper file closure after reading.
a.html
<title>Document</title>
<body>
<h1>GeeksforGeeks</h1>
</body>
Python3
from bs4 import BeautifulSoup
with open ( 'a.html' , 'r' ) as file :
soup = BeautifulSoup( file , 'html.parser' )
print (soup.title)
print (soup.body)
|
Output:
<title>Document</title>
<body>
<h1>GeeksforGeeks</h1>
</body>
Complete Code
Python3
import json
import csv
from bs4 import BeautifulSoup
with open ( 'a.txt' , 'r' ) as file :
text_content = file .read()
print (text_content)
with open ( 'a.json' , 'r' ) as file :
json_content = json.load( file )
print (json_content)
with open ( 'a.csv' , 'r' ) as file :
csv_reader = csv.reader( file )
for row in csv_reader:
print (row)
with open ( 'a.html' , 'r' ) as file :
soup = BeautifulSoup( file , 'html.parser' )
print (soup.title)
print (soup.body)
|
Output:
GeeksforGeeks
{'GeeksforGeeks': '1st Rank'}
['name GeeksforGeeks']
<title>Document</title>
<body>
<h1>GeeksforGeeks</h1>
</body>
Conclusion
In conclusion, loading files into the Python console is a crucial skill for handling diverse data formats. By using techniques outlined in this guide, whether opening and reading text, parsing JSON or CSV, or navigating HTML with BeautifulSoup, Python developers gain the proficiency to seamlessly integrate file handling into their projects, enhancing data manipulation capabilities. Understanding these methods facilitates efficient interaction with different file types.
Share your thoughts in the comments
Please Login to comment...