How to List all Files in a Directory in Java?
Last Updated :
07 Feb, 2024
Java provides a feature to interact with the file system through the java.io.file package. This feature helps developers automate the things to operate with files and directories.
In this article, we will learn how to list all the files in a directory in Java.
Approaches to list all files in a directory
We have two ways to get the list of all the files in a directory in Java.
- Using listFiles()
- Using Java New I/O Package
Program to list all files in a directory in Java
Method 1: Using listFiles()
In the java.io.File class which provides an inbuilt method listFiles() that will return an array of File objects of the files in the directory.
Java
import java.io.File;
public class ListFilesExample
{
public static void main(String[] args)
{
String directoryPath = "C:/Users/GFG0354/Documents/JavaCode" ;
File directory = new File(directoryPath);
File[] files = directory.listFiles();
if (files != null ) {
for (File file : files) {
System.out.println(file.getName());
}
}
}
}
|
Output:
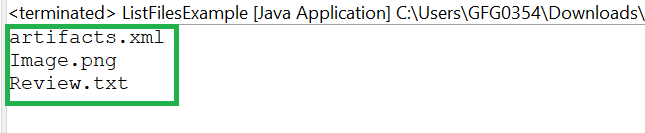
Explanation of the Program:
- In the above program, we have specified the path of the directory in the
directoryPath
variable.
- We have created a
File
object named directory
representing the specified directory path.
- We have used the
listFiles()
method of the File
class to retrieve an array of File
objects representing the files and directories contained within the specified directory.
- We iterate over the array of
File
objects and print the name of each file using the getName()
method.
Method 2: Using Java New I/O Package
New I/O provides a new easy way to interact with Files and apply file operations. The Files and Path classes are available in the java.nio.file package which we can use to list the files in a directory.
Java
import java.io.IOException;
import java.nio.file.DirectoryStream;
import java.nio.file.FileVisitOption;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.EnumSet;
public class NewIOExample {
public static void main(String[] args) throws IOException
{
String directoryPath = "C:/Users/GFG0354/Documents/JavaCode" ;
Path directory = Paths.get(directoryPath);
try (DirectoryStream<Path> stream = Files.newDirectoryStream(directory)) {
for (Path file : stream) {
System.out.println(file.getFileName());
}
}
}
}
|
Output:
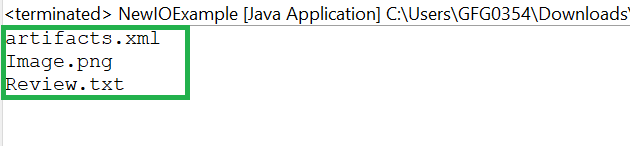
Explanation of the Program:
- In the above program, we have specified the path of the directory in the
directoryPath
variable.
- We have created a
Path
object named directory
representing the specified directory path using the Paths.get()
method.
- We have used the
Files.newDirectoryStream()
method to create a DirectoryStream
for the specified directory.
Share your thoughts in the comments
Please Login to comment...