How to include a template with parameters in EJS ?
Last Updated :
04 Mar, 2024
EJS (Embedded JavaScript) is a templating language that enables dynamic content generation in HTML files using JavaScript syntax. EJS templates consist of HTML code mixed with JavaScript expressions enclosed within <% %> tags.
We will discuss the following approaches to include template with parameters in EJS
Including Templates in EJS:
EJS provides an include function to include other EJS templates within a main template.
To include a template, use the syntax
<%- include('template_name.ejs') %>
where ‘template_name.ejs’ is the path to the template file. This allows you to reuse common sections of HTML across multiple pages, enhancing code modularity and maintainability.
Steps to Create Application:
Step 1: Create a project folder using the following command.
mkdir ejs-temp
cd ejs-temp
Step 2: Initialize the node application using the following command.
npm init -y
Step 3: Install the required libraries:
npm install express ejs
Folder Structure:

The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Step 4: Create the required folders and fies as seen in the folder structure and Add the following code
Javascript
<!-- included_template.ejs -->
<div>
<p>Parameter 1: <%= param1 %></p>
<p>Parameter 2: <%= param2 %></p>
</div>
|
Approach 1: Using Custom Functions
We define a custom function getParams in server.js to return an object with parameters. We pass this function as a parameter named getParams to the main.ejs template.In main.ejs, we call getParams() within the include statement to dynamically retrieve the parameters and pass them to the included template.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Main Template</ title >
</ head >
< body >
< h1 >Main Template</ h1 >
<%- include('included_template.ejs', getParams()) %>
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const ejs = require( 'ejs' );
const app = express();
function getParams() {
return { param1: 'Hello' , param2: 'World' };
}
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
res.render( 'main' , { getParams: getParams });
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
Approach 2: Using Global Variables
Using global variables, values are assigned to param1 and param2 directly in the server.js file, making them accessible across all EJS templates without needing to pass them explicitly, simplifying the template rendering process.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Main Template</ title >
</ head >
< body >
< h1 >Main Template</ h1 >
<%- include('included_template.ejs') %>
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const ejs = require( 'ejs' );
const app = express();
global.param1 = 'Geeks' ;
global.param2 = 'ForGeeks' ;
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
res.render( 'main' );
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
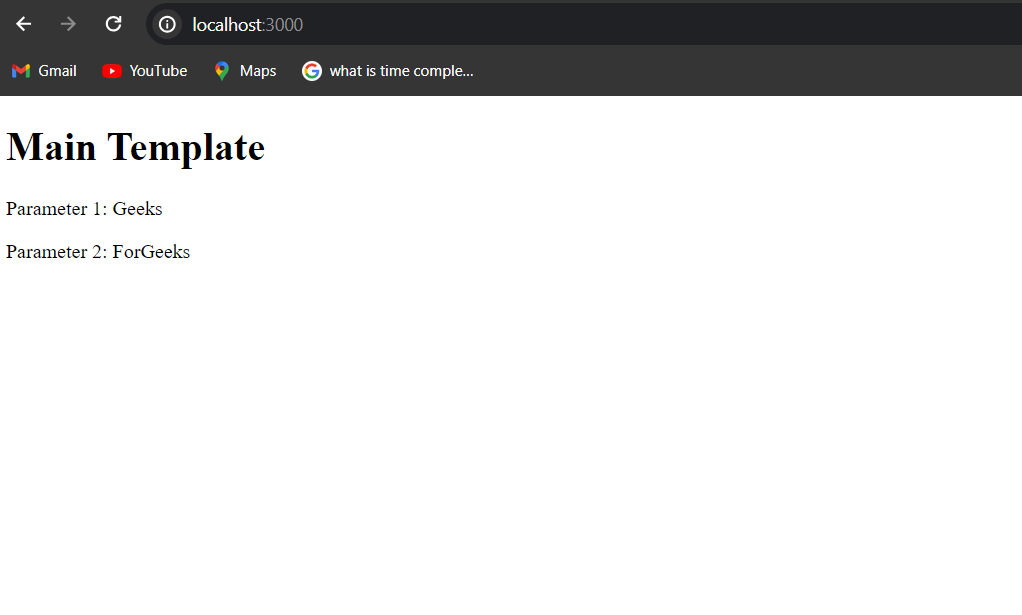
Approach 3: By Passing Data Object
Passing parameters via data object involves creating an object containing the parameters and passing it to the included template’s render function. In the main EJS template, you use <%- include(‘included_template.ejs’, data) %> to include the template and pass the data object. Then, in the included template, you can access the parameters using EJS syntax, such as <%= param1 %>.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Main Template</ title >
</ head >
< body >
< h1 >Main Template</ h1 >
<%- include('included_template.ejs', data) %>
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const ejs = require( 'ejs' );
const app = express();
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
const data = { param1: 'hello' , param2: 'geek' };
res.render( 'main' , { data: data });
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
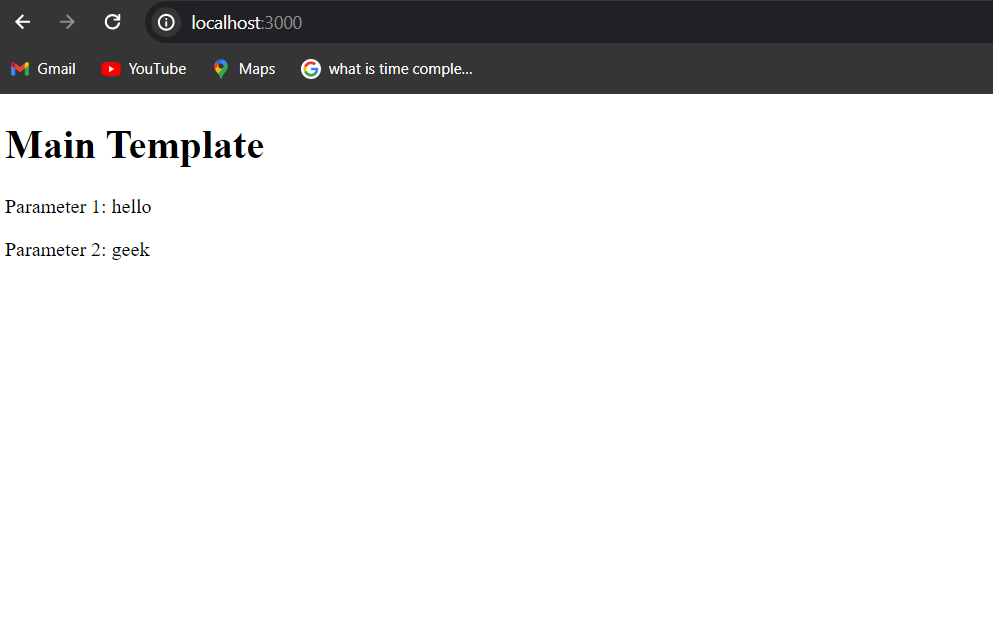
Approach 4: By Passing Parameters Directly
Parameters are passed directly to the included template within the include statement in the main EJS template. This method allows for inline specification of parameter values without the need for intermediate variables. It’s a straightforward approach suitable for passing static or predefined values to the included template.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Main Template</ title >
</ head >
< body >
< h1 >Main Template</ h1 >
<%- include('included_template.ejs', {param1: param1, param2: param2}) %>
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const ejs = require( 'ejs' );
const app = express();
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
res.render( 'main' , { param1: 'Good' , param2: 'Evening' });
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output: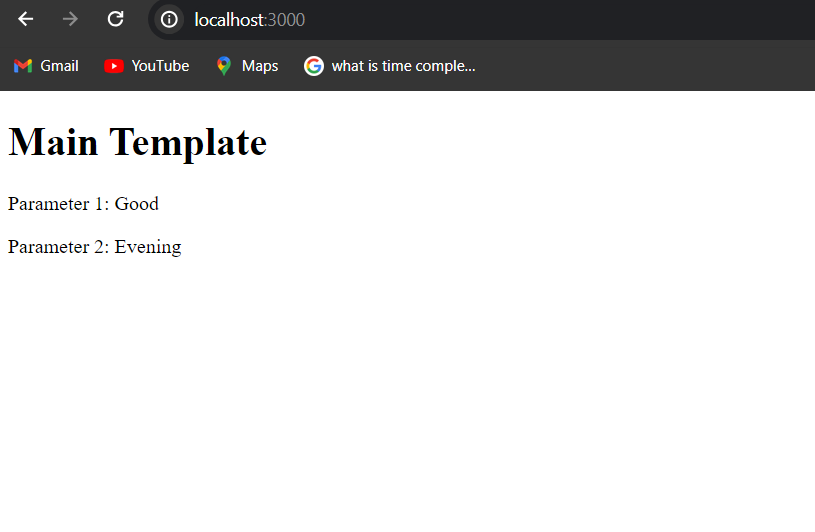
Share your thoughts in the comments
Please Login to comment...