How to import dbutils in Scala?
Last Updated :
01 Apr, 2024
In Scala, dbutils typically refers to a utility library provided by Databricks for interacting with databases and performing data-related tasks within Databricks environments. To import dbutils in Scala, follow these steps:
Steps:
- Open a Databricks Notebook: First, ensure you’re working within a Databricks Notebook environment.
- Create a Scala Notebook: Create a new Scala notebook or use an existing one.
- Import dbutils: In a Scala notebook, dbutils is already available by default, so you don’t need to explicitly import it. You can directly use it in your Scala code.
Example 1:
Here’s an example of how you might use dbutils within a Databricks Scala notebook to read data from a CSV file and display the first few rows:
Scala
// Assuming you have a CSV file uploaded to Databricks File System (DBFS)
val filePath = "dbfs:/FileStore/sample.csv"
// Use dbutils to read the CSV file
val df = spark.read.option("header", "true").csv(filePath)
// Print a message indicating that the file is read successfully
println(s"File '$filePath' is read successfully.")
In this code:
- dbutils is used implicitly to access the file system.
- spark is the SparkSession object, which is also available by default in Databricks notebooks.
Output:
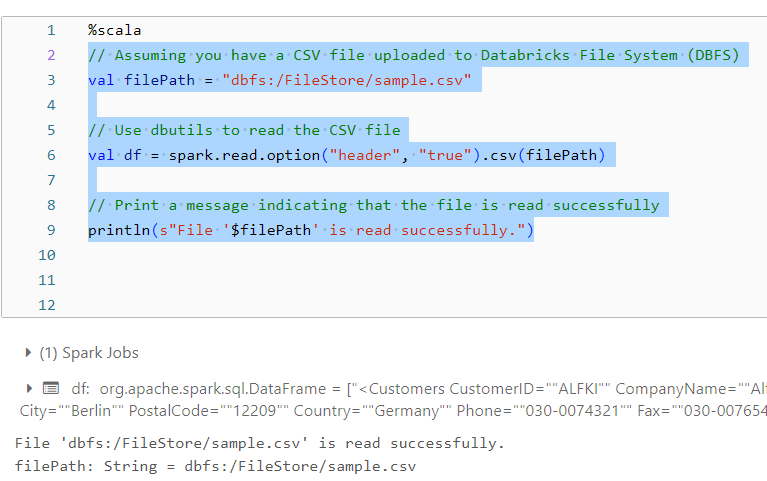
Example 2:
Another example demonstrating how to use dbutils in a Databricks Scala notebook to perform file operations like moving a file from one location to another within the Databricks File System (DBFS):
Scala
// Define source and destination file paths
val sourceFilePath = "/path/to/source/file.txt"
val destinationFilePath = "/path/to/destination/file.txt"
// Use dbutils to move the file from source to destination
dbutils.fs.mv(sourceFilePath, destinationFilePath)
// Check if the file has been moved successfully
val fileExistsAtDestination = dbutils.fs.ls(destinationFilePath).nonEmpty
if (fileExistsAtDestination) {
println(s"File moved successfully from $sourceFilePath to $destinationFilePath")
} else {
println(s"Failed to move file from $sourceFilePath to $destinationFilePath")
}
In this code:
- We define the source and destination file paths within the Databricks File System (DBFS).
- We use dbutils.fs.mv() to move the file from the source to the destination.
- Then, we check if the file exists at the destination using dbutils.fs.ls().
- Finally, we print a success or failure message based on whether the file exists at the destination.
Share your thoughts in the comments
Please Login to comment...