How to Force SSL/https in Express ?
Last Updated :
14 Jan, 2024
Securing web applications is crucial in today’s digital world. One fundamental security practice is ensuring that your application communicates over a secure connection. For Express applications, this involves configuring the server to serve content over HTTPS instead of HTTP.
In this article, we will learn how we can secure our application with the help of SSL certification and run the application on HTTPS instead of HTTP.
Prerequisites:
Steps to download SSL and use https:
Step 1: Install Chocolatey and mkcert
Open PowerShell as Administrator and install Chocolateyby running the following command.
Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString(‘https://chocolatey.org/install.ps1’))
Now Install the mkcert Using Chocolatey, run the following command in the powershell window.
choco install mkcert
To verify the installation of the mkcert, check the version of the mkcert using the following command.
mkcert -version
After installing mkcert, run the following command to create and install a local CA in the system trust store. A local CA (Certificate Authority) is a privately established entity that functions like a publicly trusted CA.
mkcert -install
Now we will create the SSL Certificate, for this make sure to navigate to the directory where you want to save your certificates and run, note that it will create two files: localhost.pem (the certificate) and localhost-key.pem (the private key).
mkcert localhost
Step 2: Create an Express.js Application and install required dependencies.
npm init -y
npm install express https
Folder Structure:
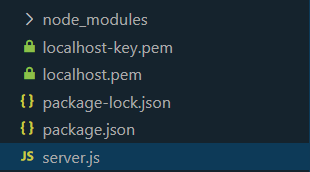
Folder Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"https": "^1.0.0"
}
Example: Add the following code in your server.js file.
Javascript
const express = require( 'express' );
const https = require( 'https' );
const fs = require( 'fs' );
const app = express();
const privateKey = fs.readFileSync( 'localhost-key.pem' , 'utf8' );
const certificate = fs.readFileSync( 'localhost.pem' , 'utf8' );
const passphrase = 'gaurav' ;
const credentials = { key: privateKey, passphrase, cert: certificate };
const httpsServer = https.createServer(credentials, app);
function ensureSecure(req, res, next) {
if (req.secure) {
return next();
}
res.redirect( 'https://' + req.hostname + req.originalUrl);
}
app.use(ensureSecure);
app.get( '/' , (req, res) => {
res.send( 'Hello, welcome to the secure server!' );
});
httpsServer.listen(443, () => {
console.log( 'HTTPS server running on port 443' );
});
|
Run Your Express.js Application by running the following command in the terminal.
node index.js
Step 4: Access the Application
Open the browser and go to the https://localhost:443 and it will show the message that is mentioned in the code. Here we can see in the image below that output is currect and the certificate is also valid and the connection is secure that means it is using the https protocol.
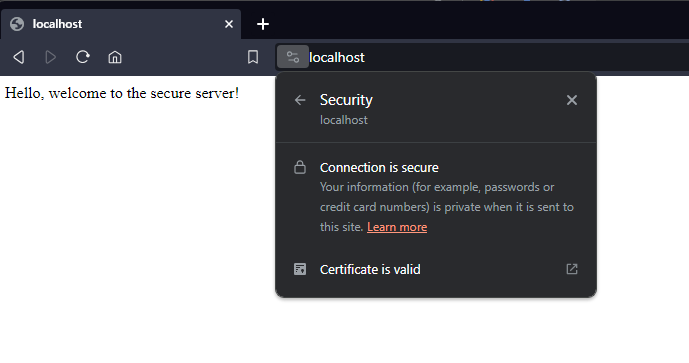
Share your thoughts in the comments
Please Login to comment...