How to fix: “fatal error: Python.h: No such file or directory”
Last Updated :
21 Aug, 2023
The “fatal error: Python.h: No such file or directory” error is a common issue encountered when compiling C/C++ code that interacts with Python. This error occurs when the C/C++ compiler is unable to locate the Python.h header file, which is part of the Python development package required for compiling code that interacts with Python.In this article, we will discuss in detail how to fix the “fatal error: Python.h: No such file or directory” error, providing step-by-step instructions and guidelines to successfully compile C/C++ code that interacts with Python.
Problem Statement :
When attempting to compile C/C++ code that interacts with Python, developers often encounter the error message “fatal error: Python.h: No such file or directory”. This error occurs when the C/C++ compiler is unable to locate the Python.h header file, which is essential for compiling code that interacts with Python.
This error can pose a significant hurdle in building and running C/C++ programs that depend on Python, preventing developers from successfully integrating Python functionalities into their code. It can be caused by various factors, such as the Python development package not being installed, incorrect header file location, improper compiler settings, or missing environment variables related to Python.
Problem Screenshot:
The error message “fatal error: Python.h: No such file or directory” typically occurs when the C/C++ compiler is unable to find the Python.h header file, which is part of the Python development package required for compiling C/C++ code that interacts with Python.
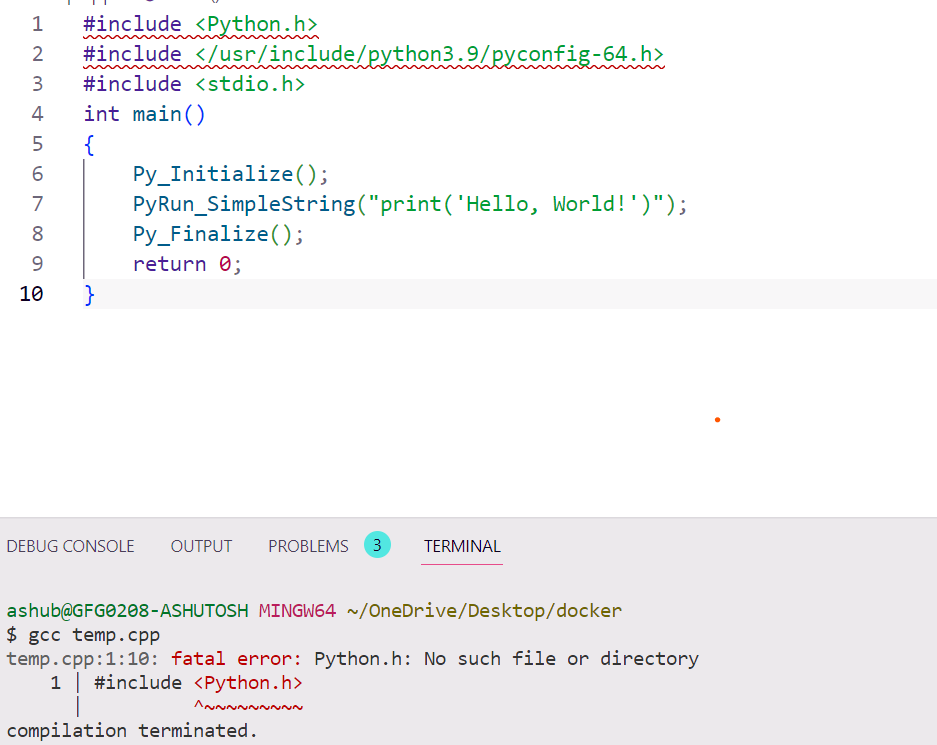
Error
Steps required to solve the problem :
Step 1: Install the Python development package.
Installing the Python development package is an essential step when working with Python, especially when compiling C/C++ code that interacts with Python. The development package provides the header files and libraries necessary for compiling Python extension modules or embedding Python in other applications. In this article, we will guide you through the installation process for different Linux-based systems.
For Ubuntu/Debian-based systems:
Ubuntu and Debian-based systems, such as Ubuntu, Debian, and Linux Mint, use the apt-get package manager. To install the Python development package, open a terminal window and run the following command with administrative privileges:
sudo apt-get update
sudo apt-get install python-dev
For Fedora/RHEL-based systems:
Fedora and Red Hat Enterprise Linux (RHEL) use the yum package manager. To install the Python development package, open a terminal window and run the following command with administrative privileges:
sudo yum install python-devel
For Arch Linux:
Arch Linux uses the pacman package manager. To install the Python development package, open a terminal window and run the following command with administrative privileges:
sudo pacman -S python
Step 2 : Set up in the include path.
Including Python.h is typically required when embedding Python in other applications, such as creating Python extension modules, embedding Python interpreter in a C/C++ program, or calling Python functions from C/C++ code. This header file defines the Python C API, which is a set of C functions and macros that allow C/C++ code to interact with the Python interpreter and Python objects.
Including the pyconfig-64.h header file is a crucial step when compiling C/C++ code that interacts with Python x.y. It provides platform-specific configuration settings and macros that are necessary for correctly building Python extension modules or embedding Python in other applications. It’s important to verify the correct path of the pyconfig-64.h header file on your system and ensure that the Python development headers are installed and configured correctly to avoid compilation errors.
C++
#include </usr/include/python3.9/pyconfig-64.h>
|
Step 3 : Complie and run
After setting the inclusion path and installing the Python development package, you should be able to successfully build and run your application.
For Example :
Py_Initialize(): This function initializes the Python interpreter in the C/C++ program. It must be called before any Python code can be executed.
PyRun_SimpleString(“print(‘Hello, World!’)”): This function runs a simple Python code string within the C/C++ program. In this case, it prints the string “Hello, World!” to the standard output using the Python interpreter embedded within the C/C++ program.
Py_Finalize(): This function finalizes the Python interpreter in the C/C++ program. It must be called after all Python code has been executed.
C++
#include <Python.h>
#include </usr/include/python3.9/pyconfig-64.h>
#include <stdio.h>
int main()
{
Py_Initialize();
PyRun_SimpleString( "print('Hello, World!')" );
Py_Finalize();
return 0;
}
|
.png)
Code Image
To create a output file we need to run the command
gcc -I/usr/include/python3.9 test.c -o test -l/usr/lib -lpython3.9
Let’s break down the command and understand its components:
- gcc: This is the command to invoke the GCC compiler.
- -I/usr/include/python3.9: This option specifies the directory where the Python 3.9 header files are located (/usr/include/python3.9). The -I option is used to add the directory to the compiler’s include path, allowing it to find the necessary header files during compilation.
- test.c: This is the name of the C file that you want to compile.
- -o test: This option specifies the name of the output file that will be generated after successful compilation. In this case, the output file will be named test.
- -L/usr/lib: This option specifies the directory where the Python 3.9 library files are located (/usr/lib). The -L option is used to add the directory to the compiler’s library search path, allowing it to find the necessary Python library during linking.
- -lpython3.9: This option specifies the name of the Python 3.9 library that should be linked with the compiled code. The -l option is used to specify a library to be linked, and the library name follows the -l option without the lib prefix or file extension. In this case, it specifies the python3.9 library.
Once the compilation command is executed successfully without any errors, it should generate an executable file named test (or the name specified with the -o option), which can be executed to run your program that interacts with Python 3.9.
./test
Output :
Hello World
.png)
Terminal Output
Share your thoughts in the comments
Please Login to comment...