How to Execute a SQL Query with Named Parameters in JDBC?
Last Updated :
09 Apr, 2024
Executing the SQL (Structured Query Language) query with named parameters in JDBC (Java Database Connectivity) is the fundamental feature of the database interaction in Java. Named parameters provide convenient and it provides a secure way to pass the values into the SQL queries without using concatenating the strings against attacks of SQL injection. While JDBC does not support the named parameters, we can achieve the same functionalities using the prepared statements.
Note: The Named parameters are emulated using placeholders like “?"
in prepared statements.
Prerequisites:
The following are the prerequisites to execute an SQL query with named parameters in JDBC.
- Java Development Kit (JDK)
- JDBC Driver (Java Database Connectivity)
- Database Connection
- Prepared Statement
Step-by-step implementation to execute a SQL query with named parameters in JDBC
Below is the step-by-step implementation to execute SQL query with Named Parameters in JDBC.
Step 1: Create a table in the Database.
Create a table in the database and name it as “login” and column names as “uname” and “password”. Insert the rows into the table. Here is the example for “login” table:
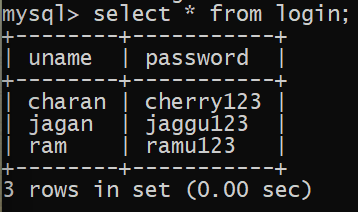
Step 2: Create Java Project in Eclipse.
Open Eclipse IDE and create a Java project, name it as “JDBCExample”.
Step 3: Add MYSQL JDBC Driver to the project.
- First download the MYSQL JDBC driver (jar file) from the MYSQL website.
- Open Eclipse, right click on the name of the project in the Package Explorer.
- After that, select the Build Path and then Configure Build Path.
- There is Libraries tab and then click Add External JARs and then select the downloaded MYSQL JDBC driver JAR file.
- After that, click Apply and then Apply and close button.
Here is the path for MYSQL JDBC driver jar file:
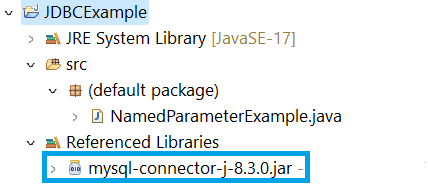
Step 4: Create a java class in Java project.
Create a class in the src folder in java project, and name it as “NamedParameterExample“. Here is the path for java class file:
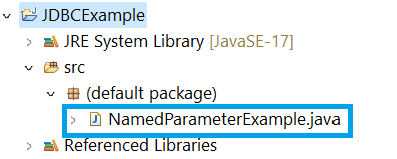
Step 5: Implement the code
Open the java class file and write the below code to execute a SQL query with named parameters in JDBC.
Java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class NamedParameterExample
{
public static void main(String[] args)
{
// Database connection parameters
String url = "jdbc:mysql://localhost:3307/work"; //url for the database
String username = "root"; // username of database
String password = "tiger"; // password of database
// SQL query with named parameters
String sql = "SELECT * FROM login WHERE uname = ? AND password = ?";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement stmt = conn.prepareStatement(sql)) {
// Set parameter values using placeholder
stmt.setString(1, "jagan");
stmt.setString(2, "jaggu123");
// Execute the query
try (ResultSet rs = stmt.executeQuery()) {
while (rs.next()) {
// Process the result set
// For example:
String column1Value = rs.getString("uname");
String column2Value = rs.getString("password");
System.out.println( " uname : " + column1Value + ", password: " + column2Value);
// ...
}
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation of the above Program:
- In the above project, we established the connection to the database with the help of “DriverManager.getConnection ()”.
- We have created the “PreparedStatement” using the SQL query.
- Then, we set the parameter values with the help of “setString()” method with using the parameter name as the first argument.
- Now, we will execute the query using the “executeQuery()” method.
Note: Ensure that you should replace the connection parameters such as URL, username, password with your details of the database connections. And replace the SQL query and parameters names with the your query and parameter names.
Step 6: Run the code
- Right click on the java class file and select Run As > Java Application.
- After running the code, the output will be shown in the console window in eclipse as shown below.
Output:
After running the java application, we can see the below output in console.
Share your thoughts in the comments
Please Login to comment...