How to End setTimeout After User Input ?
Last Updated :
04 Jan, 2024
To end setTimeout after user input, you can use the clearTimeout
function. The clearTimeout() function in JavaScript clears the timeout which has been set by the setTimeout() function before that.
Syntax:
clearTimeout(name_of_setTimeout);
Approach
- Set an initial countdown time (e.g., 5 seconds) and a variable for the timeout ID.
- Use
setTimeout
to decrement the countdown and update the display every second. Set a new timeout for the next second until the countdown reaches 0.
- Listen for a button click event (e.g., with the ID
myButton
).
- On button click(or on input or keypress etc), use
clearTimeout
to stop the countdown by clearing the timeout using the stored timeout ID.
Example 1: In this example, we have followed the above-explained approach.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Timeout Example</ title >
< style >
#myButton {
cursor: pointer;
}
</ style >
</ head >
< body >
< button id = "myButton" >Click me to clear timeout</ button >
< div id = "output" ></ div >
< script >
document.addEventListener('DOMContentLoaded', function () {
let countdown = 5;
let timestamp;
const updateCountdown = () => {
document.getElementById('output')
.innerHTML = `Timeout in ${countdown} seconds`;
if (countdown === 0) {
document.getElementById('output')
.innerHTML = "Timeout completed!";
} else {
timestamp = setTimeout(updateCountdown, 1000);
}
countdown--;
};
updateCountdown();
document.getElementById('myButton')
.addEventListener('click', () => {
clearTimeout(timestamp);
document.getElementById('output')
.innerHTML = "Timeout cleared!";
});
});
</ script >
</ body >
</ html >
|
Output:
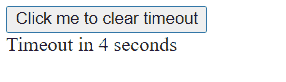
Example 2: In this example, if we type something in the input box, Timer stops.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Timeout Example with Text Input</ title >
< style >
#textInput {
margin-bottom: 10px;
}
</ style >
</ head >
< body >
< input type = "text"
id = "textInput"
placeholder = "Type to stop the timeout" >
< div id = "output" ></ div >
< script >
document.addEventListener('DOMContentLoaded', function () {
let countdown = 5;
let timestamp;
const updateCountdown = () => {
document.getElementById('output').innerHTML =
`Timeout in ${countdown} seconds`;
if (countdown === 0) {
document.getElementById('output').innerHTML =
"Timeout completed!";
} else {
timestamp = setTimeout(updateCountdown, 1000);
}
countdown--;
};
updateCountdown();
document.getElementById('textInput').addEventListener('input',
(event) => {
if (event.target.value.toLowerCase() !== '') {
clearTimeout(timestamp);
document.getElementById('output').innerHTML =
"Timeout cleared!";
}
});
});
</ script >
</ body >
</ html >
|
Output:
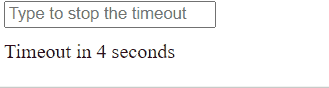
Example 3: In this example, if we press(keydown event) the spacebar, timer stops.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Timeout Example with Keydown Event</ title >
</ head >
< body >
< div id = "output" ></ div >
< script >
document.addEventListener('DOMContentLoaded', function () {
let countdown = 5;
let timestamp;
const updateCountdown = () => {
document.getElementById('output').innerHTML =
`Timeout in ${countdown} seconds`;
if (countdown === 0) {
document.getElementById('output').innerHTML =
"Timeout completed!";
} else {
timestamp = setTimeout(updateCountdown, 1000);
}
countdown--;
};
updateCountdown();
document.addEventListener('keydown', (event) => {
if (event.code === 'Space') {
clearTimeout(timestamp);
document.getElementById('output').innerHTML =
"Space bar is pressed and Timeout cleared!";
}
});
});
</ script >
</ body >
</ html >
|
Output:
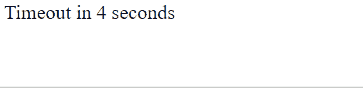
Share your thoughts in the comments
Please Login to comment...