How to draw with mouse in HTML 5 canvas ?
Last Updated :
25 Jan, 2023
In this article, we shall explore a few ways to draw with the mouse pointer on the HTML 5 canvas. The HTML canvas is essentially a container for various graphics elements such as squares, rectangles, arcs, images, etc. It gives us flexible control over animating the graphics elements inside the canvas. However, functionality to the canvas has to be added through JavaScript.
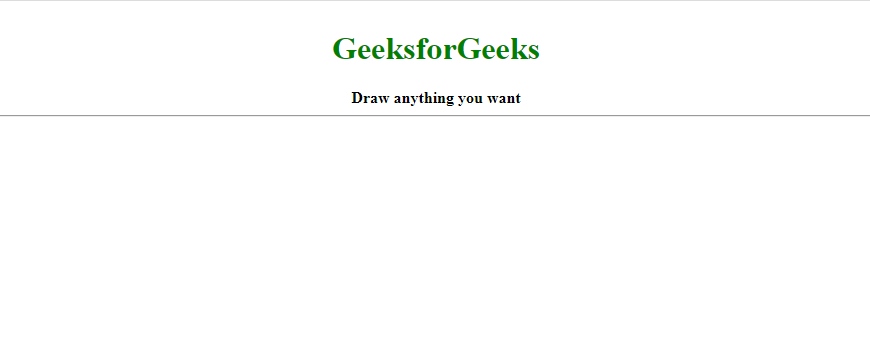
In the following procedure, we will use a flag variable to toggle the drawing on and off in relation to the mouse events. The events we will be listening to the mousedown, mouseup and the mousemove events in JavaScript.
The canvas element by default has some properties such as padding etc.(can be changed styles). Hence, properties offsetTop and offsetLeft are used to retrieve the position of the canvas, relative to its offsetParent (closest ancestor element of the canvas in the DOM). By subtracting these values from event.clientX and event.clientY, we can reposition the starting point of the drawing to the tip of the cursor. In the function sketch(), we use the following in-built methods to add functionality.
- beginPath(): Starts a new path, every time left mouse button is clicked.
- lineWidth: Sets the width of the line that will be drawn.
- strokeStyle: In this regard, we use it to set the color of the line to black. This attribute can be changed to produce lines of different colors.
- moveTo(): The Starting position of the path moves to the specified coordinates on the canvas.
- lineTo(): Creates a line to from the said position to the coordinates specified.
- stroke(): Adds stroke to the line created. Without this, the line will not be visible.
Example: Creating a Canvas Element and adding the JavaScript functionality.
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
Draw with the mouse in a HTML5 canvas
</ title >
< style >
* {
overflow: hidden;
}
body {
text-align: center;
}
h1 {
color: green;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< b >Draw anything you want</ b >
< hr >
< canvas id = "canvas" ></ canvas >
< script src = "index.js" ></ script >
</ body >
</ html >
|
Javascript
window.addEventListener( 'load' , ()=>{
resize();
document.addEventListener( 'mousedown' , startPainting);
document.addEventListener( 'mouseup' , stopPainting);
document.addEventListener( 'mousemove' , sketch);
window.addEventListener( 'resize' , resize);
});
const canvas = document.querySelector( '#canvas' );
const ctx = canvas.getContext( '2d' );
function resize(){
ctx.canvas.width = window.innerWidth;
ctx.canvas.height = window.innerHeight;
}
let coord = {x:0 , y:0};
let paint = false ;
function getPosition(event){
coord.x = event.clientX - canvas.offsetLeft;
coord.y = event.clientY - canvas.offsetTop;
}
function startPainting(event){
paint = true ;
getPosition(event);
}
function stopPainting(){
paint = false ;
}
function sketch(event){
if (!paint) return ;
ctx.beginPath();
ctx.lineWidth = 5;
ctx.lineCap = 'round' ;
ctx.strokeStyle = 'green' ;
ctx.moveTo(coord.x, coord.y);
getPosition(event);
ctx.lineTo(coord.x , coord.y);
ctx.stroke();
}
|
Output: The function sketch() will only execute if the value of the flag is true.It is important to update the coordinates stored in the object coord after beginPath(), hence getPosition(event) is called. After linking the JavaScript file to the HTML file, the following code will be obtained.
Click here to see live output
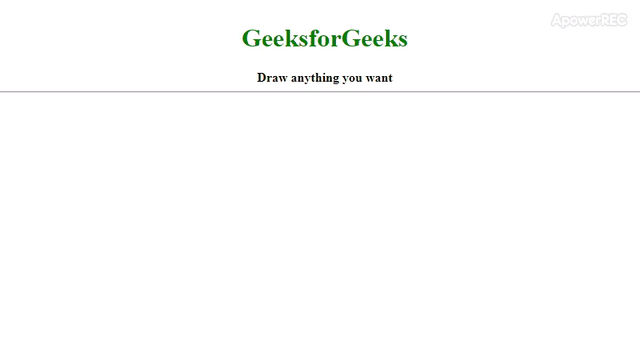
Share your thoughts in the comments
Please Login to comment...