How to Display Images in JavaScript ?
Last Updated :
29 Dec, 2023
To display images in JavaScript, we have different approaches. In this article, we are going to learn how to display images in JavaScript.
Below are the approaches to display images in JavaScript:
In an HTML document, the document.createElement() is a method used to create the HTML element. The element specified using elementName is created or an unknown HTML element is created if the specified elementName is not recognized.Â
Syntax:
let element = document.createElement("elementName");
Example: In this example, we will display an image using CreateElement.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Display Image</ title >
</ head >
< body >
< script >
function show_image(src, width, height,alt) {
// Create a new image element
let img = document.createElement("img");
// Set the source, width,
// height, and alt attributes
img.src = src;
img.width = width;
img.height = height;
img.alt = alt;
// Append the image element
// to the body of the document
document.body.appendChild(img);
}
// Example usage:
show_image(
300, 200,"gfg logo");
</ script >
</ body >
</ html >
|
Output:
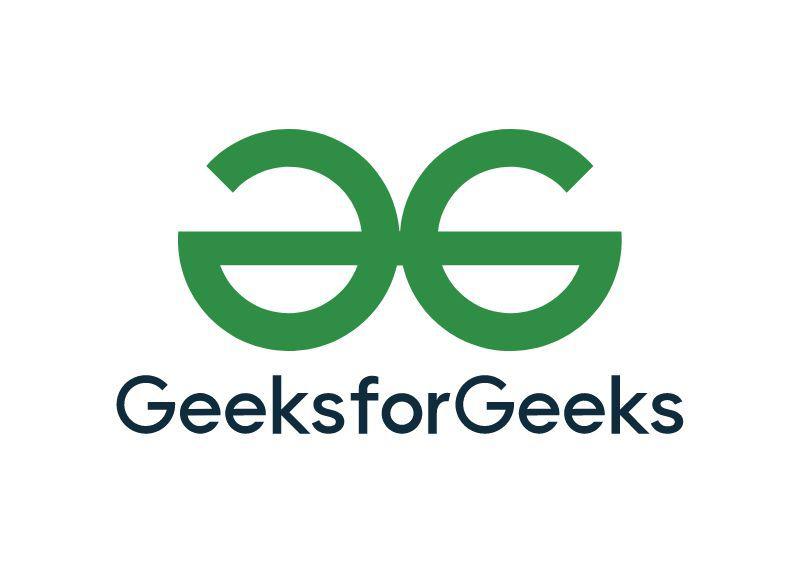
The DOMÂ innerHTMLÂ property is used to set or return the HTML content of an element.
Syntax:
It returns the innerHTML Property.
Object.innerHTML
Example: In this example, we are using InnerHTML.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Display Image</ title >
</ head >
< body >
< div id = "imageContainer" ></ div >
< script >
// You can dynamically set the innerHTML
// of a container to include an image element
const imageContainer = document
.getElementById("imageContainer");
imageContainer.innerHTML =
`< img src =
</ script >
</ body >
</ html >
|
Output:
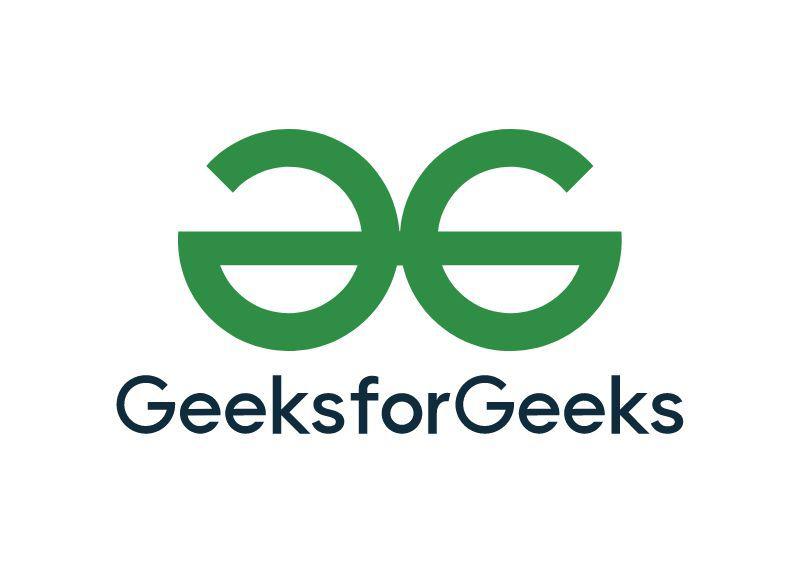
Share your thoughts in the comments
Please Login to comment...