DataTables are a modern jQuery plugin for adding interactive and advanced controls to HTML tables for a webpage. It is a very simple-to-use plug-in with a variety of options for the developer’s custom changes as per the application need. The plugin’s features include pagination, sorting, searching, and multiple-column ordering.
In this article, we will demonstrate the ajax loading of the data object using the DataTables plugin.
Approach: In the following example, DataTables uses data objects from a plain file as the main source. Each row in the table shows details for one employee’s information.
- This can be implemented by using the columns.data option of DataTables plugin API.
- The source returns an array of objects which is used to display the data in the HTML table.
The example for the structure of the data row from the data file is as follows. It simply represents the data in key and value pair as shown below.
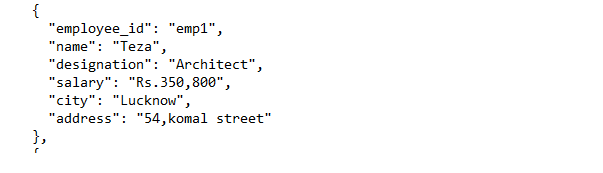
employee data object with key and value pair
The pre-compiled files which are needed to implement are
CSS:
https://cdn.datatables.net/1.10.22/css/jquery.dataTables.min.css
JavaScript:
https://code.jquery.com/jquery-3.5.1.js
https://cdn.datatables.net/1.10.22/js/jquery.dataTables.min.js
Example: The following code demonstrates the fetch of the employee data object from a file “nestedData.txt” and displays all rows in the HTML table using the plugin.
HTML
<!DOCTYPE html>
< html >
< head >
< meta content=" initial-scale = 1 , maximum-scale = 1 ,
user-scalable = 0 " name = "viewport" />
< meta name = "viewport" content = "width=device-width" />
< link rel = "stylesheet" href =
< script type = "text/javascript"
</ script >
< script type = "text/javascript" src =
</ script >
</ head >
< body >
< h2 >
How to demonstrate the use of Ajax
loading data in DataTables
</ h2 >
< table id = "tableID" class = "display" style = "width:100%" >
< thead >
< tr >
< th >EmployeeID</ th >
< th >Name</ th >
< th >Designation</ th >
< th >Salary</ th >
< th >Address</ th >
< th >City</ th >
</ tr >
</ thead >
</ table >
< script >
/* Initialization of datatable */
$(document).ready(function () {
/* Datatable access by using table ID */
$('#tableID').DataTable({
"info": false,
/* Set pagination as false or
true as per need */
"paging": false,
/* Name of the file source
for data retrieval */
"ajax": 'nestedData.txt',
"columns": [
/* Name of the keys from
data file source */
{ "data": "employee_id" },
{ "data": "name" },
{ "data": "designation" },
{ "data": "salary" },
{ "data": "address" },
{ "data": "city" }
]
});
});
</ script >
</ body >
</ html >
|
nestedData.txt: The following is the content for the file “nestedData.txt” used in the above HTML code.
{
"data": [
{
"employee_id": "emp1",
"name": "Teza",
"designation": "Architect",
"salary": "Rs.350,800",
"city": "Lucknow",
"address": "54,komal street"
},
{
"employee_id": "emp2",
"name": "Garima",
"designation": "Accountant",
"salary": "Rs.180,050",
"city": "Hyderabad",
"address": "Hitech city,kodapur"
},
{
"employee_id": "emp3",
"name": "Ashmita",
"designation": "Technical Author",
"salary": "Rs.186,000",
"city": "Kolkatta",
"address": "156, new park,chowk"
},
{
"employee_id": "emp4",
"name": "Celina",
"designation": "Javascript Developer",
"salary": "Rs.450,000",
"city": "Itanagar",
"address": "chandni chowk,new lane"
},
{
"employee_id": "emp5",
"name": "Asha",
"designation": "Accountant",
"salary": "Rs.542,700",
"city": "Tokyo",
"address": "54,Japanese colony"
},
{
"employee_id": "emp6",
"name": "Baren Samal",
"designation": "Integration Specialist",
"salary": "Rs.370,000",
"city": "New Delhi",
"address": "48,RK puram"
},
{
"employee_id": "emp7",
"name": "Hari Om",
"designation": "Sales Manager",
"salary": "Rs.437,500",
"city": "Raipur",
"address": "Sector 6,bhilai"
},
{
"employee_id": "emp8",
"name": "Ranu",
"designation": "Integration Specialist",
"salary": "Rs.330,900",
"city": "Tokyo",
"address": "64,indian colony"
},
{
"employee_id": "emp9",
"name": "Celly",
"designation": "PHP Developer",
"salary": "Rs.275,500",
"city": "Secunderabad",
"address": "23,Sainikpuri"
},
{
"employee_id": "emp55",
"name": "Ama",
"designation": "Director",
"salary": "Rs.985,000",
"city": "kanpur",
"address": "63,Narangi lane"
},
{
"employee_id": "emp56",
"name": "Michael Jackson",
"designation": "C++ Developer",
"salary": "Rs.783,000",
"city": "Singapore",
"address": "53,Sweetpark"
},
{
"employee_id": "emp57",
"name": "Danny",
"designation": "Customer Support",
"salary": "Rs.345,000",
"city": "Ludhiana",
"address": "456,Punjab"
}
]
}
Output:
Before executing the code:
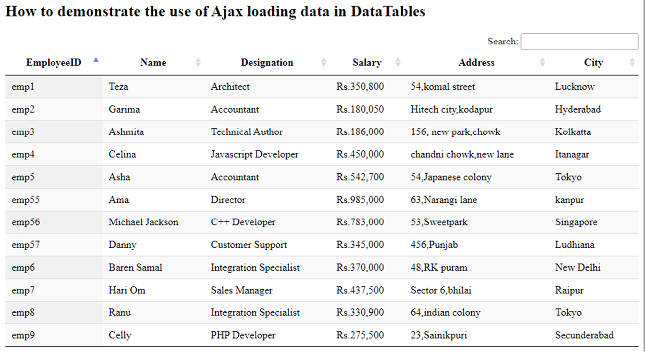
employee data
After executing the code: The following output shows that the table showing the “searching” operation for employees having designation as “Accountant” or string with suffix “Developer”. Another search is performed for City “Hyderabad” and so on.
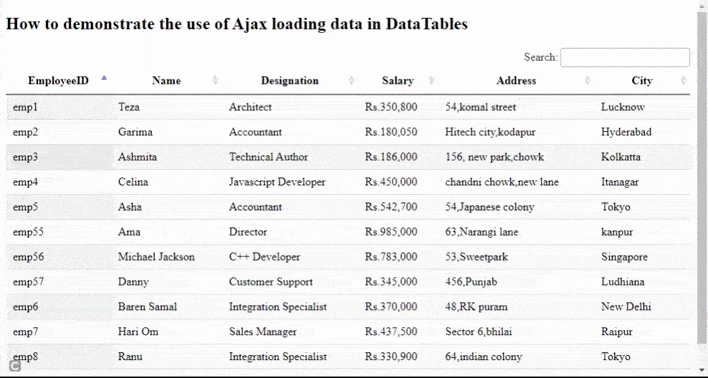
Share your thoughts in the comments
Please Login to comment...