How to Delay a Function Call in JavaScript ?
Last Updated :
26 Mar, 2024
Delaying a JavaScript function call involves executing a function after a certain amount of time has passed. This is commonly used in scenarios where you want to postpone the execution of a function, such as in animations, event handling, or asynchronous operations.
Below are the methods to delay a JavaScript function call:
Using setTimeout()
The setTimeout() method schedules a function to be executed after a specified delay in milliseconds.
Syntax:
setTimeout(function, delay, arg1, arg2, ...);
Example: Printing the output after 2 seconds using setTimeout().
JavaScript
setTimeout(() => {
console.log('Function called after 2000 milliseconds');
}, 2000);
Output:
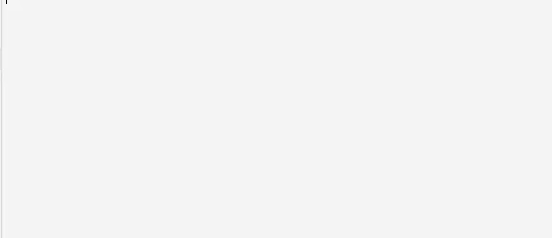
Output
setInterval()
Similar to setTimeout(), setInterval() repeatedly calls a function with a fixed time delay between each call.
Syntax:
setInterval(function, delay, arg1, arg2, ...);
Example: Printing the each output after interval of 1 second using setTimeout().
JavaScript
let count = 0;
const intervalID = setInterval(() => {
console.log('Function called every 1000 milliseconds');
count++;
if (count === 3) {
clearInterval(intervalID);
}
}, 1000);
Output:
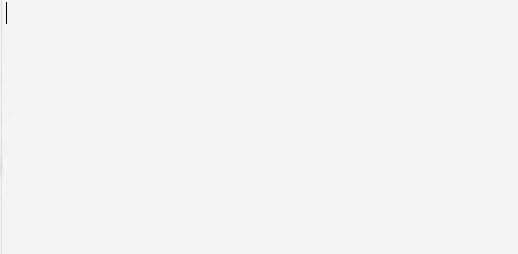
Output
Promises with setTimeout()
We can create a Promise and resolve it after a certain delay using setTimeout(). This allows for better handling of asynchronous operations.
Syntax:
const delayedFunction = (delay) => new Promise(resolve => setTimeout(resolve, delay));
Example: Printing the output at a delay of 3 seconds using Promises with setTimeout().
JavaScript
const delayedFunction = (delay) => new Promise(resolve => setTimeout(() => {
console.log('Function called after ' + delay + ' milliseconds');
resolve();
}, delay));
delayedFunction(3000);
Output:
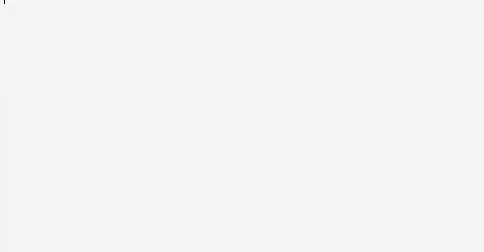
Output
Async/Await with setTimeout()
Using async/await syntax with setTimeout() allows for writing asynchronous code in a synchronous-like manner, improving code readability and maintainability.
Syntax:
async function delayedFunction(delay) {
await new Promise(resolve => setTimeout(resolve, delay));
// Your code to be executed after delay
}
Example: Printing the output at a delay of 4 seconds using Promises with setTimeout().
JavaScript
async function delayedFunction(delay) {
await new Promise(resolve => setTimeout(resolve, delay));
console.log('Function called after ' + delay + ' milliseconds');
}
delayedFunction(4000);
Output:
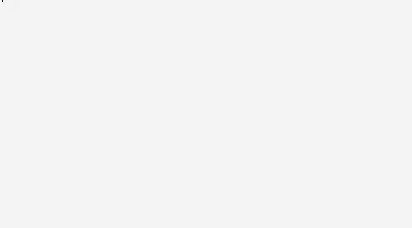
delayed function call using async/await along with setTimeout function
Share your thoughts in the comments
Please Login to comment...