How to create Selenium test cases
Last Updated :
10 Apr, 2024
Selenium IDE is an open-source tool that is widely used in conducting automated web testing and browser automation. This tool is intended mainly for Web Application testers and developers to develop, edit, and run automated test cases for Web Applications.
Selenium IDE lets you easily playback and record interactively web application activities during test automation on the browser-based interface.
What are Selenium test cases?
Selenium is an open-source/license-free web automation framework. It is a quick and easy way to test functional scenarios as it minimizes manual efforts thereby reducing human errors. This article is going to describe how to set up your local systems to run a selenium test case in Chrome Browser using Python.
Create Selenium test cases
Step 1: Install Selenium: Open Command Prompt and type command > pip install selenium
Note: It is important to have Python installed in the system.
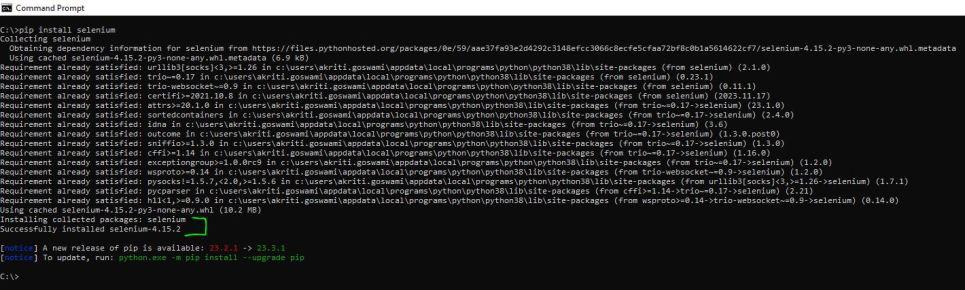
pip install selenium
Step 2: Download chrome binary and chrome driver.
Make sure both are compatible i.e., have the same version. For example, if you are using windows platform then, download the latest driver and binary for win64 or win32 based on your system’s settings.
Note: if you have Chrome browser already installed in your system, you can check the version by going to Chrome browser settings >> About Chrome or simply type in chrome://settings/help in the Chrome Browser, then in this case you will only need to download chrome driver – make sure that the chrome driver which you will be downloading should be of the same version as that of Chrome browser/binary. In cases where chrome driver of the same version is not available for Chrome binary/browser version like below – 119.0.6045.200, then please download the required version of Chrome binary/browser as well.
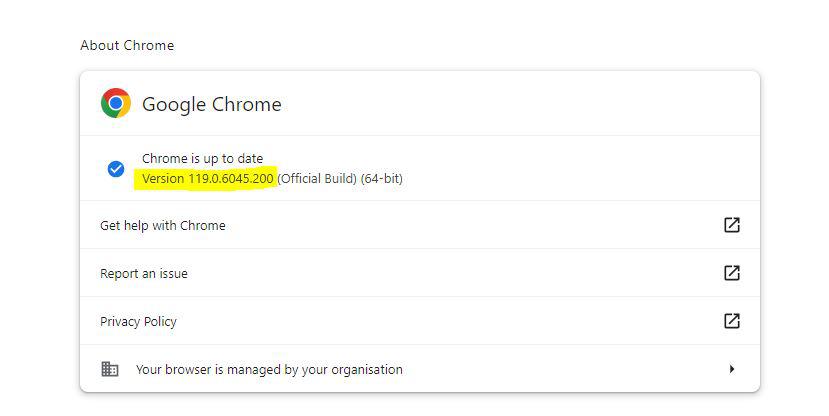
Chrome binary/browser Version
Step 3: Import the modules of selenium for web automation and sys for managing command line arguments.
Step 4: A search function is defined which will be called using 1 argument ( keyword to search in Google ). This search function contains the main implementation of the “Search Google” task and is called once when the script is run.
Step 5: webdriver_path variable should be configured to match the location of the Chrome WebDriver folder. Ensure that this variable points precisely to the location where the folder has been downloaded and extracted in alignment with the automation script. For example, both “SearchGoogle.py” script and extracted “chromedriver-win64” folder are in the same location , so webdriver_path is defined with value = “/chromedriver-win64“
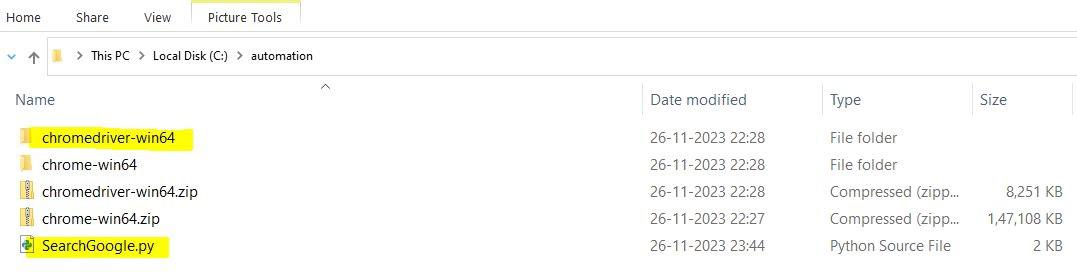
webdriver_path
Step 6: An instance of “ChromeOptions” Class is created with name chrome_options. The executable_path argument inside formatted string literal (f”…”) is used to direct Chrome for finding the WebDriver executable using webdriver_path variable.
Step 7: Chrome WebDriver initialized as “driver” variable uses WebDriver module ( which is part of the Selenium library ) with specific configuration options provided through the chrome_options variable
Step 8: Using the above driver, first the Chrome window is maximized using maximize_window() method and get() method is used to navigate the browser to the specified URL which is Google HomePage. The browser window will then display the content of the Google webpage in the Chrome Browser.
Step 9: find_element() method of the WebDriver instance (driver) is used to locate an HTML element having “name” attribute with value = “q” on the webpage which is used for Search Box input and this element is assigned to variable “search_box”.
Step 10: Next, send_keys() method simulates typing on the keyboard into a specific element, in this case, “search_box” variable defined in above step. Here, “keyword” argument is the command line argument which we will pass while calling the script. This “keyword” variable will hold the search term that needs to be entered into the search box. Keys.RETURN is a constant provided by the selenium.WebDriver.common.keys module which is used to simulate pressing the “Enter” key after typing the search term which triggers the search action.
Step 11: save_screenshot() method is used to save a screen snippet of the result displayed from the above actions at the same location as that of the python script.
The browser is closed at the end using quit() method.
Python
# python program for Selenium First Test Case
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import sys
def search(keyword):
# Set the path to your ChromeDriver directory, in my case the windows 64 chromedriver folder and python script were in the same folder, so below path is used
webdriver_path = "/chromedriver-win64"
# Set up Chrome options
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument(f"executable_path={webdriver_path}")
# Initialize the WebDriver with Chrome options
driver = webdriver.Chrome(options=chrome_options)
try:
# Before performing any operation, maximizing the Chrome browser for better display
driver.maximize_window()
# Open the Google page using the url
driver.get("https://www.google.com")
# Find the search box by its name attribute value using find_element method
search_box = driver.find_element("name", "q")
# Type the search keyword and press Enter
search_box.send_keys(keyword)
search_box.send_keys(Keys.RETURN)
# Capture a screenshot for the result generated from above operations, the screenshot name is configurable as per the search keyword
driver.save_screenshot(f"result_{keyword.replace(' ', '_')}.png")
finally:
# It is important to close the browser once all the desired operations are done
driver.quit()
# if number of arguments passed is less than or more than 2 , the exception will be handled by below code
if __name__ == "__main__":
if len(sys.argv) != 2:
print("Usage: python script.py <search_keyword>")
sys.exit(1)
search_keyword = sys.argv[1]
search(search_keyword)
Save the above code as fileName.py and run the script using below command in the command prompt:
python fileName.py “Keyword to search”
Example: if file name is saved as SearchGoogle.py
Then, below command is run:
python SearchGoogle.py “Sun”
Output
Conclusion
To summarize the above procedure, install selenium and download the chrome binary and driver in your local system. Create an object of ChromeOptions class and use this configurable object to create a Chrome webdriver for the web automation. There are various web tasks that can be automated such as navigating to URLs, interacting with webpage elements, extracting information, waiting for elements to load and executing actions like a Google search. Finally, it’s crucial to close the WebDriver after completing the automation tasks to release system resources and close the browser window.
Share your thoughts in the comments
Please Login to comment...