How to create Cookie Consent Box using HTML CSS and JavaScript ?
Last Updated :
15 Nov, 2023
In this article, we will see how cookies are stored using the cookie consent box using HTML, CSS, & JavaScript.
Cookies are small pieces of data that are stored on a user’s device while browsing the website. Cookies are used to store information for faster access to data and provide a better user experience. Also, it is used for session management and helps users log in as they go to different web pages of the same website.
To see where the cookies are stored, follow the below steps:
- Right-click on the browser
- click on the inspect option,
- Go to the Application tab, under the storage section there are ‘Cookies’
- Expand the ‘Cookies’ and now see all cookies stored by the current website.
- Also, you can manage the cookies from that section like editing, Deleting, storing, etc.
Prerequisite
Preview
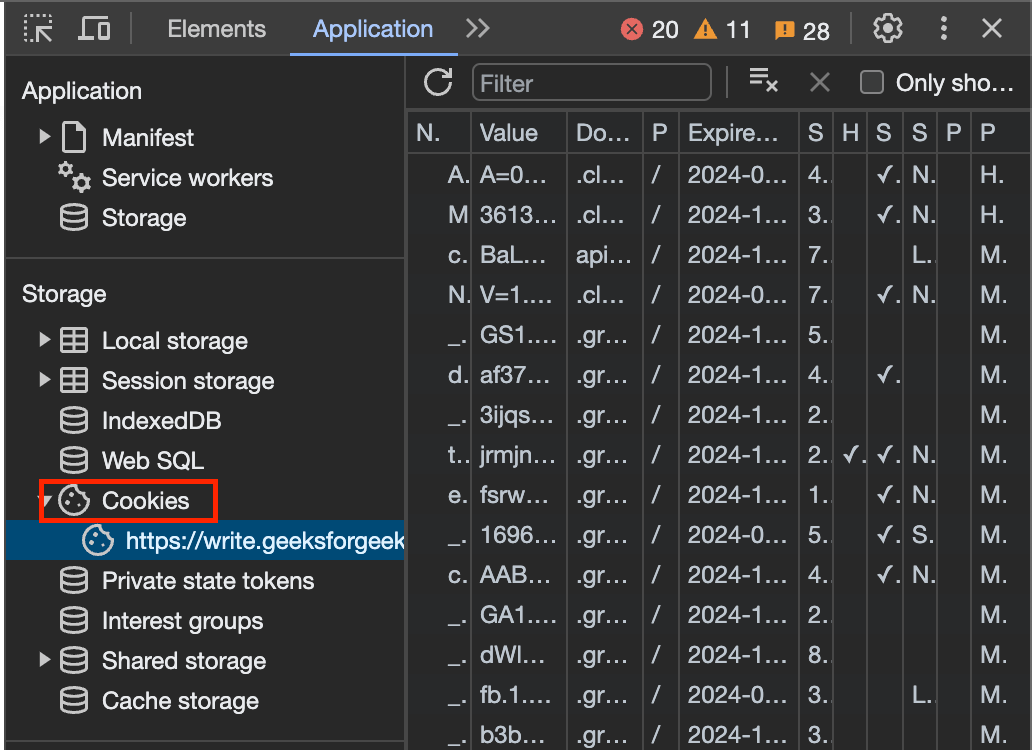
Approach
- Create an HTML file, defining a div for the cookie consent box. Inside, add an image, a header, text explaining cookie usage, and Accept/Reject buttons.
- Style the consent box using CSS, applying specific designs for elements like buttons, backgrounds, and positioning.
- Use JavaScript to handle button clicks. When the ‘Accept’ button is clicked, set a cookie and hide the consent box. On ‘Reject’, display an alert and hide the box.
- Check if a specific cookie exists. If the cookie is found, hide the consent box to reflect the user’s choice. If not, show the consent box for the user to decide.
Example: This example describes the basic creation of the Cookie Consent Box using HTML CSS & JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< title >
Cookie Consent Box using HTML CSS & JavaScript
</ title >
< link rel = "stylesheet"
href = "style.css" >
</ head >
< body >
< div id = "consentBox" >
< img src =
alt = "Logo" >
< div id = "consentContent" >
< header id = "consentHeader" >
Cookies: The choice is yours
</ header >
< p >
We use cookies to make our site work well
for you and so we can continually improve it.
< br >The cookies that are necessary to
keep the site functioning are always on
</ p >
< div class = "buttons" >
< button class = "consentButton" >
Accept Cookies
</ button >
< button class = "rejectButton" >
Reject
</ button >
</ div >
</ div >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
display : flex;
justify- content : center ;
align-items: center ;
height : 100 vh;
margin : 0 ;
background : #f3f3f3 ;
}
#consentBox {
background : #fff ;
padding : 20px ;
border-radius: 15px ;
box-shadow: 0 8px 16px rgba( 0 , 0 , 0 , 0.2 );
text-align : center ;
}
#consentBox. hide {
opacity: 0 ;
pointer-events: none ;
transform: scale( 0.8 );
transition: all 0.3 s ease;
}
::selection {
color : #fff ;
background : #229a0f ;
}
#consentContent p {
color : #858585 ;
margin : 10px 0 20px 0 ;
}
#consentContent .buttons {
display : flex;
align-items: center ;
justify- content : center ;
margin-top : 20px ;
}
.consentButton,
.rejectButton {
padding : 12px 30px ;
border : none ;
outline : none ;
color : #fff ;
font-size : 16px ;
font-weight : 500 ;
border-radius: 5px ;
cursor : pointer ;
transition: all 0.3 s ease;
}
.consentButton {
background : #2a910b ;
margin-right : 10px ;
}
.rejectButton {
color : #111211 ;
background : transparent ;
border : 2px solid #099c2c ;
text-decoration : none ;
}
#consentBox img {
max-width : 90px ;
}
#consentHeader {
font-size : 25px ;
font-weight : 600 ;
margin-top : 10px ;
}
|
Javascript
const consentBox =
document.getElementById( "consentBox" );
const acceptBtn =
document.querySelector( ".consentButton" );
const rejectBtn =
document.querySelector( ".rejectButton" );
acceptBtn.onclick = () => {
document.cookie = "CookieBy=GeeksForGeeks; max-age="
+ 60 * 60 * 24;
if (document.cookie) {
consentBox.classList.add( "hide" );
} else {
alert
( "Cookie can't be set! Please" +
" unblock this site from the cookie" +
" setting of your browser." );
}
};
rejectBtn.onclick = () => {
alert(
"Cookies rejected. Some functionality may be limited." );
consentBox.classList.add( "hide" );
};
let checkCookie =
document.cookie.indexOf( "CookieBy=GeeksForGeeks" );
checkCookie !== -1 ? consentBox.classList.add( "hide" ) :
consentBox.classList.remove( "hide" );
|
Explanation
- The HTML file includes a section for cookie consent with visual elements and buttons for user choice.
- CSS styles are applied to enhance the appearance of the consent box, customizing its visual attributes.
- JavaScript functions manage user actions, setting cookies upon ‘Accept’ and hiding the box.
- On clicking on the ‘Accept cookies’ button, a cookie named “GeeksForGeeks” is stored in the cookies storage with a maximum age of 24hrs, which means that after 24hrs the cookies are automatically deleted from the storage.
- Upon ‘Reject’, an alert is shown, and the consent box is hidden accordingly.
- The script verifies the existence of a specific cookie and adjusts the display of the consent box based on its presence or absence.
Output:
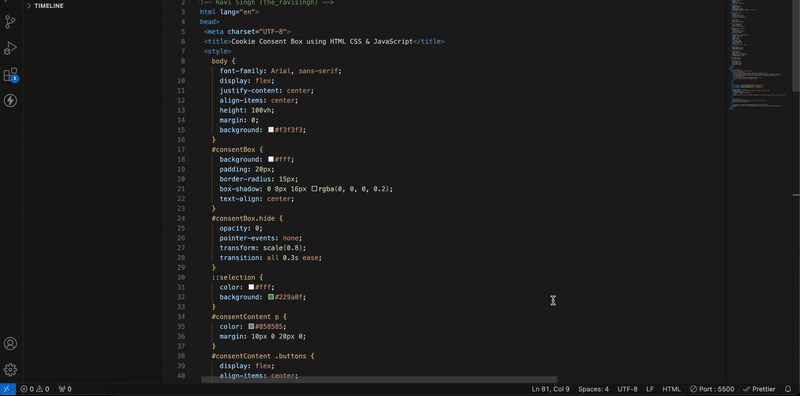
Share your thoughts in the comments
Please Login to comment...