What is Snippet and How to Create Java Snippets in VSCode for Competitive Programming?
Last Updated :
19 Feb, 2021
The snippet refers to a small piece of re-usable source code, machine code, or text that with the help of the snippet we can use long lines code again and again in our programs. Snippets are a great tool to write programs faster. Typing speed is very important for competitive programming. Generally, there are two classes used in java for Performing Input/ Output Operations.
- Scanner Class
- BufferedReader Class
Scanner Class is a class in java.util package used for obtaining the input of the primitive types like int, double, etc., and strings. It is the easiest way to read input in a Java program, though not very efficient if you want an input method for scenarios where time is a constraint like in competitive programming. Scanner class used inbuilt parsing operation which makes it slow for competitive programming.
BufferedReader Class: Reads text from a character-input stream, buffering characters to provide for the efficient reading of characters, arrays, and lines. This class is much faster than the Scanner class for performing Input/ Output Operations but requires a lot of typing)
BufferedReader is very much efficient and faster than Scanner class but the syntax for initialization of this class and performing operations is very more as compared to Scanner Class Operations.
Components of the snippet: Each snippet contains Four components
- Name of the snippet: snippet name that makes unique from different snippets
- prefix: The keyword which generates current snippets in the program
- body: The Actual code which we bind to snippets contains in the body.
- description: Information about the snippet contains in a snippet.
Format of the snippet: Snippet of particular code implemented in the java.json file which uses JSON format.
Javascript
"Name_of_the_snippet " :
{
"prefix" : "prefix_of_the_snippet" ,
"body" : [
],
"description" : "description_about_the_snippet"
}
|
Procedure: Steps Involved are as follows:
- Creating and implementing a user-defined class in a new java file.
- Now, creating a snippet of this class
Implementation: For a user-defined class. Implement all the Input/Output methods of the BufferedReader as a user-defined by implementing them as a class member in our class (which uses BufferedReader and StringTokenizer): This method uses the time advantage of BufferedReader and StringTokenizer and the advantage of user-defined methods for less typing and therefore a faster input altogether. This gets accepted with a time of 1.23 s and this method is very much recommended as it is easy to remember and is fast enough to meet the needs of most of the questions in competitive coding.
Java
import java.util.*;
import java.util.Map.Entry;
import java.io.*;
import java.util.regex.Pattern;
public class GFG {
static class FastReader {
BufferedReader br;
StringTokenizer st;
public FastReader()
{
br = new BufferedReader(
new InputStreamReader(System.in));
}
String next()
{
while (st == null || !st.hasMoreElements()) {
try {
st = new StringTokenizer(br.readLine());
}
catch (IOException e) {
e.printStackTrace();
}
}
return st.nextToken();
}
int nextInt() { return Integer.parseInt(next()); }
long nextLong() { return Long.parseLong(next()); }
double nextDouble()
{
return Double.parseDouble(next());
}
String nextLine()
{
String str = "" ;
try {
str = br.readLine();
}
catch (IOException e) {
e.printStackTrace();
}
return str;
}
}
public static void main(String[] args)
{
FastReader scan= new FastReader();
}
}
|
Output:

Step 2: Creating snippets of User-defined class in VSCode
VSCode is a Text editor that provides support for development operations and version control systems. It provides tools for a user to build hassle-free codes. It can be downloaded and installed from visualstudio.com. Now jumping onto step 2 of the procedure.
- Open VS in a folder to be created.
- Search in settings for java.js after the user snippet.
- Search SnippetGenerator.
- Paste the snippet in java.json file and check for it.
Steps are shown in detail with the visual representation for better understanding below:
2.1: Open VScode in the folder where we want to create Snippets.
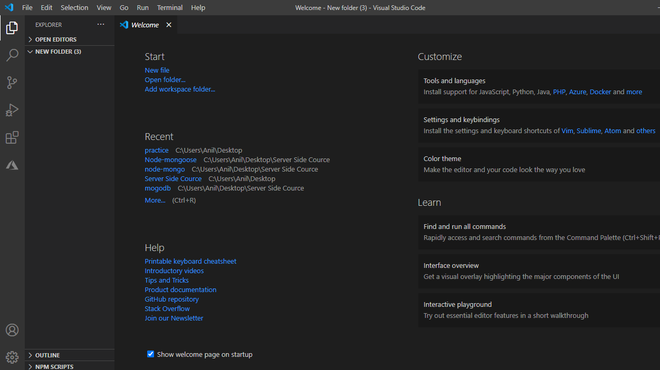
2.2: Click on the Setting button and after user snippets and search java.js in the text box. The file looks like below.
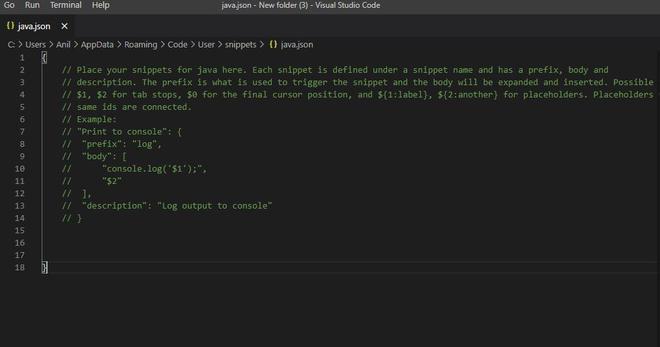
2.3: Now Search Snippet Generator this tool converts the java code into snippets. Copy the snippet of JSON from this tool.
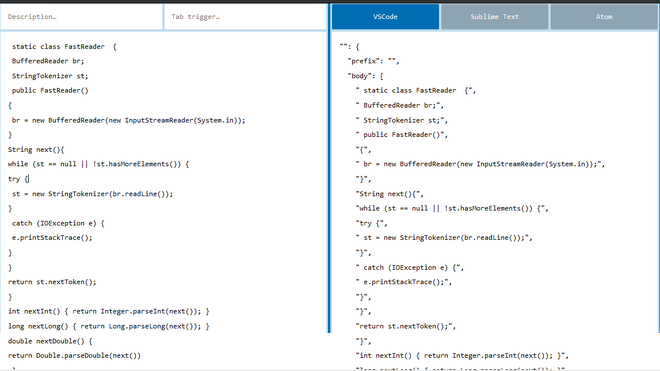
2.4: Paste the snippet in the java.json file in the program directory.
Javascript
{
"User-Defined_SnippetGFG" : {
"prefix" : "FastClass" ,
"body" : [
" static class FastReader {" ,
" BufferedReader br;" ,
" StringTokenizer st;" ,
" public FastReader()" ,
"{" ,
" br = new BufferedReader(new InputStreamReader(System.in));" ,
"}" ,
"String next(){" ,
"while (st == null || !st.hasMoreElements()) {" ,
"try {" ,
" st = new StringTokenizer(br.readLine());" ,
"}" ,
" catch (IOException e) {" ,
" e.printStackTrace();" ,
"}" ,
"}" ,
"return st.nextToken();" ,
"}" ,
"int nextInt() { return Integer.parseInt(next()); }" ,
"long nextLong() { return Long.parseLong(next()); }" ,
"double nextDouble() {" ,
"return Double.parseDouble(next())" ,
" }" ,
"String nextLine(){" ,
"String str = \"\";" ,
"try {" ,
"str = br.readLine();" ,
"}" ,
"catch (IOException e) {" ,
"e.printStackTrace();" ,
"}" ,
"return str;" ,
"}" ,
"}" ,
"" ,
" "
],
"description" : ""
}
}
|
Output: java.json file
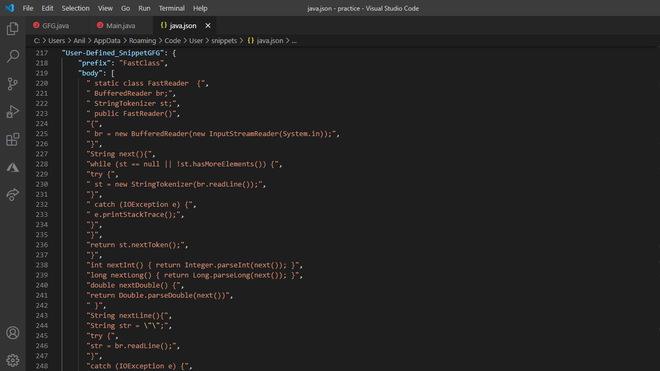
2.5: Checking the snippet: FastClass is the keyword to launch the snippet.
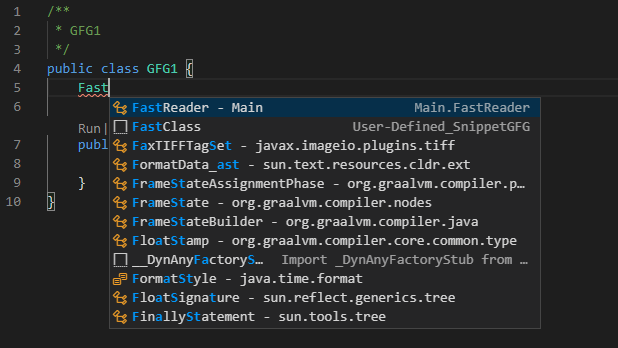
2.6. GFG1.java file
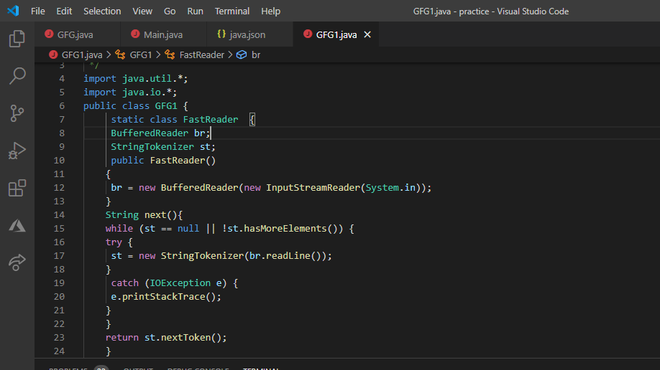
Share your thoughts in the comments
Please Login to comment...