How to create dark mode using prefer-color-scheme media query ?
Last Updated :
08 Jun, 2023
In this article, we are creating a dark mode interface using the prefer-color-scheme media query.
The prefer-color-scheme is a new media query that is used to detect the current theme of your system and provide a feature that helps in creating your web page theme according to your system preference. If your phone (or any device) is currently in dark mode then this media query will detect it, and you can use the custom CSS of your choice.
Syntax:
@media (prefer-color-scheme : your_color_scheme)
{
...
}
At the place of your_color_scheme, you can use the dark or light option. The dark indicates that the user has notified the system that they prefer an interface that has a dark theme and the light indicates that the user has notified the system that they prefer an interface that has a light theme, or has not expressed an active preference.
@media (prefer-color-scheme : dark) { ... }
@media (prefer-color-scheme : light) { ... }
Example: We have created a dummy webpage using HTML and CSS, and we want to create it in dark mode.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Creating dark mode</ title >
< style >
.main {
border: 1px solid black;
padding: 20px;
}
</ style >
</ head >
< body >
< center >
< div class = "main" >
< h1 >Geeksforgeeks</ h1 >
< p >< i >A computer science portal for geeks</ i ></ p >
< br />< br />
< p >Creating dark mode using prefers-color-scheme</ p >
< br />
< img src =
</ div >
</ center >
</ body >
</ html >
|
Output:
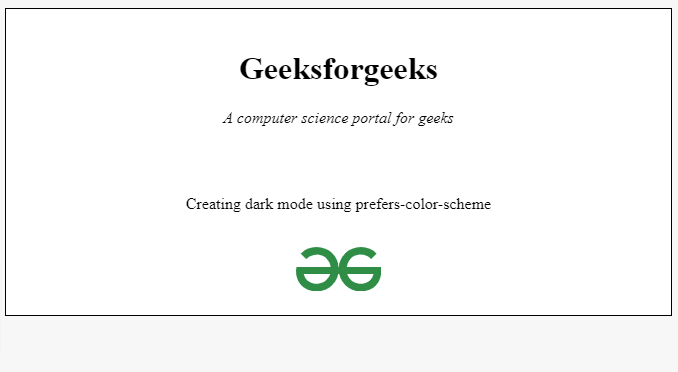
Creating the dark mode UI: Now create a prefers-color-scheme media query and set it to dark. We are creating all the black texts to white and all the white backgrounds to black if the system is using dark mode.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Creating dark mode</ title >
< style >
.main {
border: 1px solid black;
padding: 20px;
}
@media (prefers-color-scheme: dark) {
h1,
p,
div {
color: white;
}
div {
background-color: black;
}
}
@media (prefers-color-scheme: light) {
h1,
p,
div {
color: black;
}
div {
background-color: white;
}
}
</ style >
</ head >
< body >
< center >
< div class = "main" >
< h1 >Geeksforgeeks</ h1 >
< p >< i >A computer science portal for geeks</ i ></ p >
< br />< br />
< p >Creating dark mode using prefers-color-scheme</ p >
< br />
< img src =
</ div >
</ center >
</ body >
</ html >
|
Output:
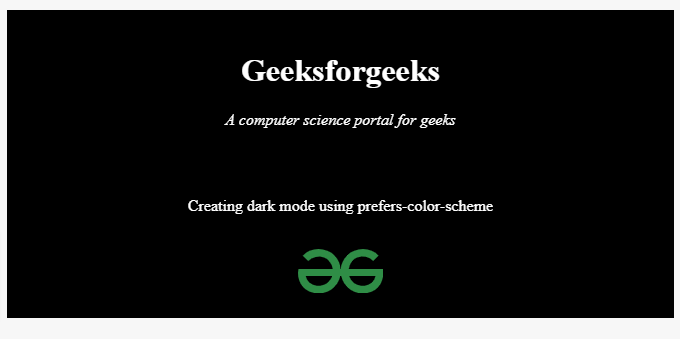
Detect the current mode of the system using JavaScript: Sometimes we need to detect the current mode of the theme. For example, if we want to use two separate images for light mode and dark mode then you need to detect the current theme mode. To detect the mode, we use the matchMedia( ) method. This method takes a string (the media query you want to search) and returns the result of that media query.
Example: In the following code snippet, we are searching for the result of the prefers-color-scheme media query.
Javascript
const a = window.matchMedia( "(prefers-color-scheme: dark)" );
console.log(a.matches);
|
Output:
- If the system theme is dark, it returns:
true
- If the system theme is light, it returns:
false
Share your thoughts in the comments
Please Login to comment...