How to create a table in ReactJS ?
Last Updated :
02 Nov, 2023
When displaying data in an organized style, Table in React JS is a popular component. Using HTML tables and JavaScript, you can quickly construct dynamic and interactive tables in ReactJS.
Prerequisites
The pre-requisites for creating a Table in React:
Steps to create React application
Step 1: Create a react application by typing the following command in the terminal.
npx create-react-app react-table
Step 2: Now, go to the project folder i.e. react-table by running the following command.
cd react-table
Project Structure:
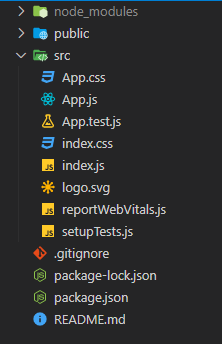
Approach 1: Table with Hardcoded values
Using HTML tables along with the hardcoded data we will simply used the HTML table elements which are <table>, <tr>, <th>, and <td> elements.
Example: We will see how to create a table using the hardcoded values using HTML tables.
Javascript
import './App.css' ;
function App() {
return (
<div className= "App" >
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Gender</th>
</tr>
<tr>
<td>Anom</td>
<td>19</td>
<td>Male</td>
</tr>
<tr>
<td>Megha</td>
<td>19</td>
<td>Female</td>
</tr>
<tr>
<td>Subham</td>
<td>25</td>
<td>Male</td>
</tr>
</table>
</div>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
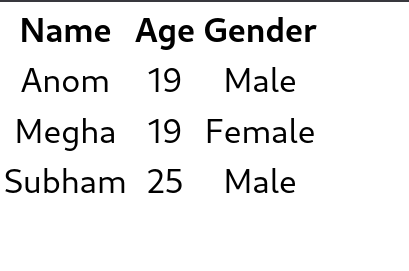
Approah 2: Create a dynamic Table from array of objects
Now let us see how we can dynamically render data from an array. Instead of manually iterating over the array using a loop, we can simply use the inbuilt Array.map() method. The Array.map() method allows you to iterate over an array and modify its elements using a callback function. The callback function will then be executed on each of the array’s elements. In this case, we will just return a table row on each iteration.
Example: Creating a Table from an array of objects using array map and html table elements.
Javascript
import './App.css' ;
const data = [
{ name: "Anom" , age: 19, gender: "Male" },
{ name: "Megha" , age: 19, gender: "Female" },
{ name: "Subham" , age: 25, gender: "Male" },
]
function App() {
return (
<div className= "App" >
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Gender</th>
</tr>
{data.map((val, key) => {
return (
<tr key={key}>
<td>{val.name}</td>
<td>{val.age}</td>
<td>{val.gender}</td>
</tr>
)
})}
</table>
</div>
);
}
export default App;
|
CSS
.App {
width : 100% ;
height : 100 vh;
display : flex;
justify- content : center ;
align-items: center ;
}
table {
border : 2px solid forestgreen;
width : 800px ;
height : 200px ;
}
th {
border-bottom : 1px solid black ;
}
td {
text-align : center ;
}
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
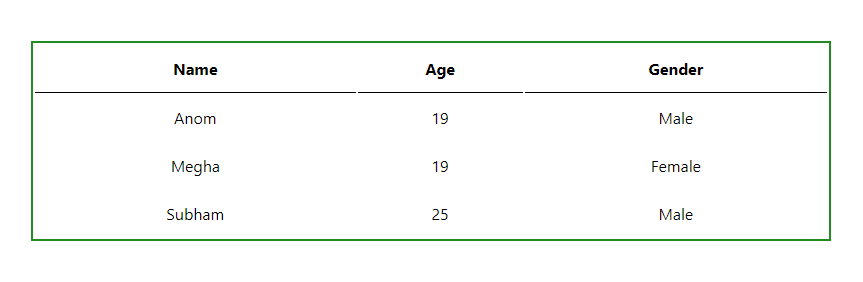
Share your thoughts in the comments
Please Login to comment...