How to Create a WebSocket Connection in JavaScript ?
Last Updated :
21 Mar, 2024
WebSocket is a powerful communication protocol enabling real-time data exchange between clients and servers. In this guide, we’ll explore how to establish a WebSocket connection using JavaScript. Below are the steps outlined for implementation:
Approach
“To establish a WebSocket connection in JavaScript, we need to configure a WebSocket server on the backend and WebSocket clients on the front end. The server listens for incoming WebSocket connections, while the client initiates a WebSocket handshake and communicates with the server using JavaScript’s WebSocket API.
For the backend, we’ll use Node.js and the ws library to create a WebSocket server. The ws library facilitates WebSocket connection management, message handling, and broadcasting to connected clients.
On the front end, we’ll instantiate a WebSocket object using the WebSocket constructor. We’ll utilize the onopen, onmessage, and onclose events to manage various operations, and employ the send() method to transmit messages to the server.”
Syntax (Client side):
const socket = new WebSocket('ws://localhost:8080');
socket.onopen = function(event) {
// Handle connection open
};
socket.onmessage = function(event) {
// Handle received message
};
socket.onclose = function(event) {
// Handle connection close
};
function sendMessage(message) {
socket.send(message);
}
Syntax (Server-side Node.js):
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
ws.on('message', function incoming(message) {
// Handle incoming message
});
ws.on('close', function() {
// Handle connection close
});
});
Steps to create Application
Step 1: Create a NodeJS application using the following command:
npm init
Step 2: Install required Dependencies:
npm i ws
The updated dependencies in package.json file will look like:
"dependencies": {
"ws": "^8.16.0"
}
Example: The Below example is demonstrating the use of WebSocket.
index.js (project root folder)
JavaScript
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', function connection(ws) {
console.log('Client connected');
ws.on('message', function incoming(message) {
console.log('Received: %s', message);
ws.send(`${message}`);
});
ws.on('close', function () {
console.log('Client disconnected');
});
});
index.html
HTML
<!-- File path: index.html (project root folder) -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>WebSocket Client</title>
<style>
h1 {
color: green;
}
.container {
margin: 10px;
}
</style>
</head>
<body>
<h1>WebSocket Example</h1>
<div class="container">
<label>Send Message to Server:</label> <br><br>
<input type="text" id="messageInput">
<button onclick="sendMessage()">Send</button>
<div id="output"></div>
</div>
<script>
// Create a WebSocket instance
// and connect to the server
const socket = new WebSocket('ws://localhost:8080');
// Event listener for when
//the WebSocket connection is opened
socket.onopen = function (event) {
// Alert the user that they are
// connected to the WebSocket server
alert('You are Connected to WebSocket Server');
};
// Event listener for when a message
// is received from the server
socket.onmessage = function (event) {
// Get the output div element
const outputDiv = document
.getElementById('output');
// Append a paragraph with the
// received message to the output div
outputDiv
.innerHTML += `<p>Received <b>"${event.data}"</b> from server.</p>`;
};
// Event listener for when the
// WebSocket connection is closed
socket.onclose = function (event) {
// Log a message when disconnected
// from the WebSocket server
console.log('Disconnected from WebSocket server');
};
// Function to send a message
// to the WebSocket server
function sendMessage() {
// Get the message input element
const messageInput = document
.getElementById('messageInput');
// Get the value of
// the message input
const message = messageInput.value;
// Send the message to
// the WebSocket server
socket.send(message);
// Clear the message input
messageInput.value = '';
}
</script>
</body>
</html>
To launch the application, execute the following command in your terminal:
node index.js
Then, open the `index.html` file in your web browser.”
Output:
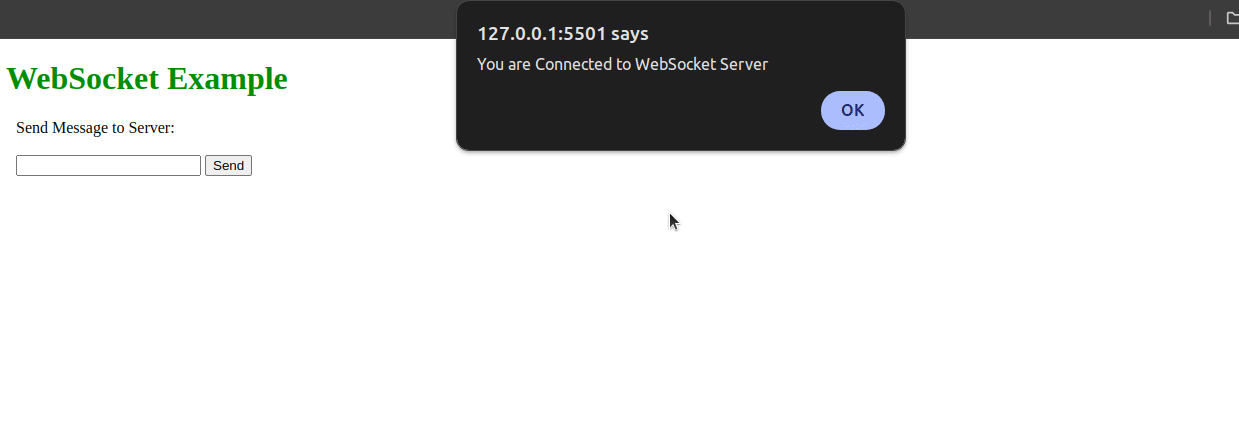
Server side output:
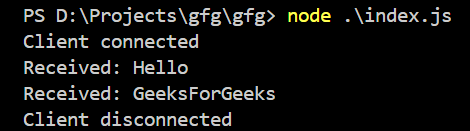
Share your thoughts in the comments
Please Login to comment...