How to create a simple server using Express JS?
Last Updated :
01 Dec, 2023
In web application development, Express.js is one of the simplified frameworks for the creation of scalable web servers with proper features for building web and mobile applications. Express.js has list of middleware which makes developers easy to quickly set up servers, define routes, and handle HTTP requests.
Prerequisites
In this article, we will create a simple server using Express.js and also handle the client’s request for testing the created server using Express.js.
Steps to create a simple server using Express.js
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
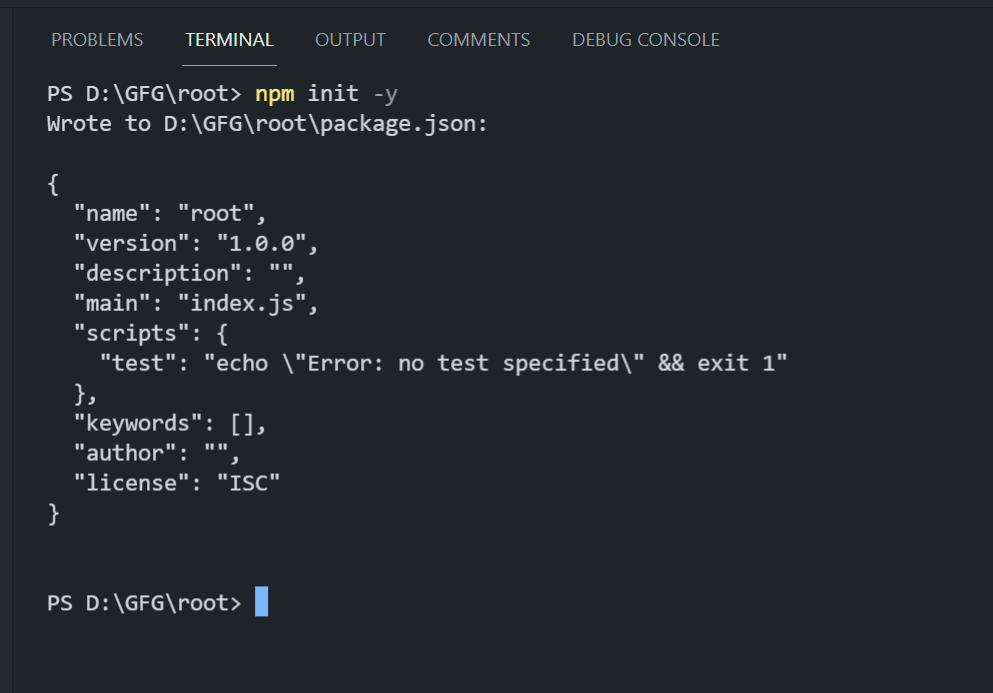
Step 3: Once the project is been initialized, we need to install Express dependencies in our project by using the below installation command of NPM.
npm i express
Dependencies:
"dependencies": {
"express": "^4.18.2",
}
Step 4: Now create the below Project Structure in your project which includes public/index.html and server.js file.
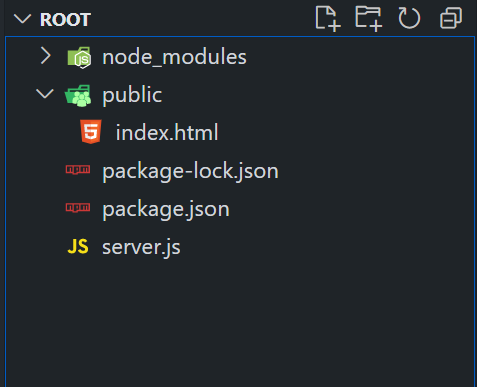
Step 5: Now, we need to write code for our server.js file and in index.html.
server.js
const express = require( 'express' );
const app = express();
const port = 3000;
app.use(express.static( 'public' ));
app.get( '/api/message' , (req, res) => {
const message = 'Hello Geek. This Message is From Server' ;
res.json({ message });
});
app.listen(port, () => {
console.log(`Server is listening at http:
});
|
index.html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >Express.js Server</ title >
</ head >
< body >
< h1 >Client-Server Communication</ h1 >
< button onclick = "getMessage()" >Get Message from Server</ button >
< p id = "message" ></ p >
< script >
async function getMessage() {
const response = await fetch('/api/message');
const data = await response.json();
document.getElementById('message').innerText = data.message;
}
</ script >
</ body >
</ html >
|
To run the application, we need to start the server by using the below command.
node server.js
Output:
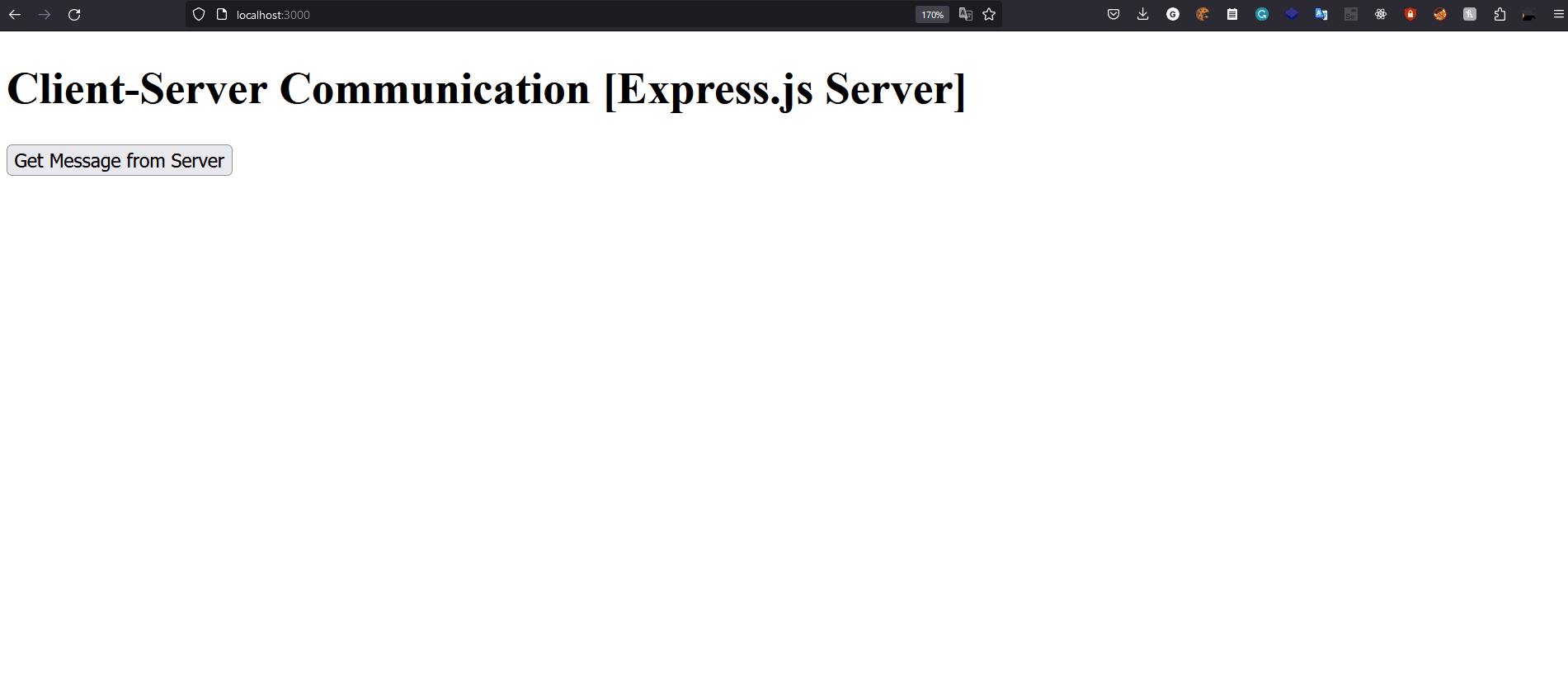
Share your thoughts in the comments
Please Login to comment...