How to create a Custom Hook in React ?
Last Updated :
26 Feb, 2024
Custom Hooks are special functions that we create in our application to extract certain functionality and increase reusability. These hooks are basically just regular JavaScript functions that begin with the prefix “use“. If you’re reading this, chances are you’re already familiar with built-in hooks such as useState and useEffect. After going through this article, you’ll gain a better understanding of custom hooks.
What is a Custom Hook?
Custom hooks are hooks that we create ourselves. We often have to perform repetitive tasks in many components, so instead of repeating the same code over and over again, we can create a custom hook and use it in all components wherever needed. This makes it easier to maintain our code as if we ever need to change the logic, we just have to modify the code of that hook. We don’t have to change anything in each component individually.
How to Create a Custom Hook?
- Define a Function: Create a JavaScript function. Its name should start with
use
to indicate it’s a custom hook.
- Use React Hooks: Within the custom hook function, you can use built-in React hooks like
useState
, useEffect
, useContext
, etc., or even other custom hooks.
- Encapsulate Logic: Implement the desired logic within the custom hook. This can include state management, side effects (like data fetching), or any other functionality needed by your components.
- Return Values: Ensure the custom hook returns the necessary values or functions that your components will use. Typically, this involves returning state variables and any functions required to manipulate that state.
- Export the Hook: Export the custom hook function so it can be imported and used in other components.
- Usage: In your functional components, import and use the custom hook by calling it. Then, destructure the values returned by the hook and use them in your component as needed.
File name can be anything but try to use it same as the hook name and also remember that function name should always start with the prefix “use”, this is not mandatory but recommended. So let’s make a custom hook.
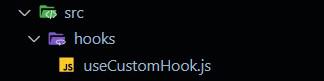
Folder Structure
Steps to Create Custom Hook in React Application:
Step 1:Â Create a react project folder, open the terminal, and write the following command.
npm create-react-app foldername
Step 2: Navigate to the root directory of your project using the following command.
cd foldername
Examples of Creating Custom Hooks in React
Example 1: Suppose we have to fetch data from GitHub API and use it in multiple components, instead of fetching data in every component, we will create a custom hook, and separate the logic of fetching the data easily.
- Always add the “use” prefix in name of hook.
- Keep in mind that one hook should perform a single task only, not more than one, as this increases the modularity of the code and makes the code easier to understand and test.
- While testing, test these hooks with different scenarios and testcases to ensure they are working properly.
Javascript
import { useState, useEffect } from 'react' ;
const useGitHubData = (username) => {
const [userData, setUserData] = useState( null );
const [loading, setLoading] = useState( false );
const [error, setError] = useState( '' );
useEffect(() => {
const fetchData = async () => {
if (!username) return ;
setLoading( true );
try {
const response = await
fetch(`https:
if (!response.ok) {
throw new Error
( 'User not found or failed to fetch data' );
}
const data = await response.json();
setUserData(data);
setError( '' );
} catch (error) {
setError( 'User not found or failed to fetch data' );
setUserData( null );
}
setLoading( false );
};
fetchData();
}, [username]);
return { userData, loading, error };
};
export default useGitHubData;
|
Javascript
import useGitHubData from './useGitHubData' ;
const GitHubDataComponent = () => {
const { userData, loading, error } = useGitHubData( "Tapesh-1308" );
console.log( "UserData:" , userData);
console.log( "Loading:" , loading);
console.log( "Error:" , error);
return <></>;
};
export default GitHubDataComponent;
|
Output:
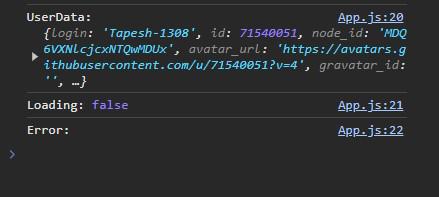
Output
Example 2: Let’s see another example to get more clarity, why not build a feature that will tell if the user is online or not? Companies use this same feature to show if the user is online or not.
Javascript
import { useState, useEffect } from 'react' ;
const useOnlineStatus = () => {
const [isOnline, setIsOnline] = useState(navigator.onLine);
useEffect(() => {
const handleOnline = () => {
setIsOnline( true );
};
const handleOffline = () => {
setIsOnline( false );
};
window.addEventListener( 'online' , handleOnline);
window.addEventListener( 'offline' , handleOffline);
return () => {
window.removeEventListener( 'online' , handleOnline);
window.removeEventListener( 'offline' , handleOffline);
};
}, []);
return isOnline;
};
export default useOnlineStatus;
|
Javascript
import useOnlineStatus from './useOnlineStatus' ;
const OnlineStatusComponent = () => {
const isOnline = useOnlineStatus();
console.log( "User is online:" , isOnline);
return <></>;
};
export default OnlineStatusComponent;
|
Output:
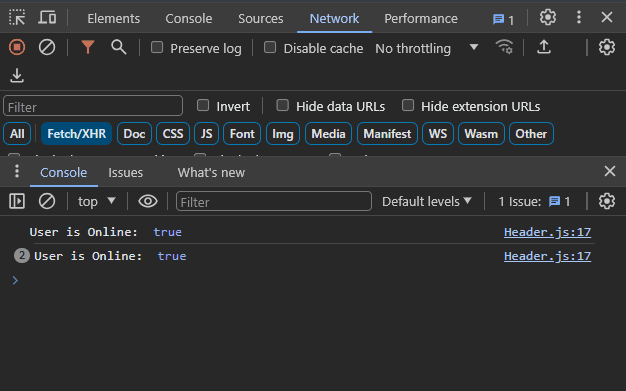
Output
Conclusion:
- Hooks are nothing but normal javascript functions made to achieve some functionality.
- Custom Hooks are those hooks that we made to extract reusable logic from UI.
- Using custom hooks makes our code readable, modular, and easy to understand and test.
- Things to make sure is to add the “use” prefix, and a hook must have a single responsibility.
Share your thoughts in the comments
Please Login to comment...