How to Convert Scala Map into JSON String?
Last Updated :
28 Mar, 2024
In this article, we will learn to convert a Scala Map into JSON String. We can convert Scala Map to JSON String using various libraries and custom conversion functions.
What is Scala Map?
In Scala, a Map is a collection of key-value pairs where each key is unique and maps to a corresponding value. It is similar to a dictionary in other programming languages. The Scala uses the immutable Map by default. If we want to use a mutable Map, then we need to import the import scala.collection.mutable.Map class explicitly.
Syntax:
val mapName: Map[KeyType, ValueType] = Map(key1 -> value1, key2 -> value2, …)
What is JSON String?
A JSON string is a textual representation of data in JSON (JavaScript Object Notation) format. It is commonly used for data interchange between a client and a server in web development and for storing structured data in a human-readable and machine-readable format.
Syntax:
val jsonString: String = “{\”key1\”: \”value1\”, \”key2\”: 2, \”key3\”: true}”
Converting Scala Map into JSON String
Below are the possible approaches to convert the scala map into JSON string.
Approach 1: Using Play JSON Library
- In this approach, we are using the Play JSON library in Scala to convert a Scala Map into a JSON string.
- First, we create a Scala Map with key-value pairs, and then we use Json.toJson and Json. stringify functions from Play JSON to convert the map into a JSON string.
Example:
The below example uses Play JSON Library to convert Scala Map into JSON String.
Scala
// Scala program to convert Scala Map into JSON String
import play.api.libs.json.Json
// Creating object
object GFG {
// Main method
def main(args: Array[String]): Unit = {
// Creating a map
val map = Map(
"name" -> "GeeksforGeeks",
"founder" -> "Sandeep Jain",
"Place" -> "Noida"
)
// Converting Map into JSON String
val res = Json.stringify(Json.toJson(map))
// Printing Output
println(res)
}
}
Output:
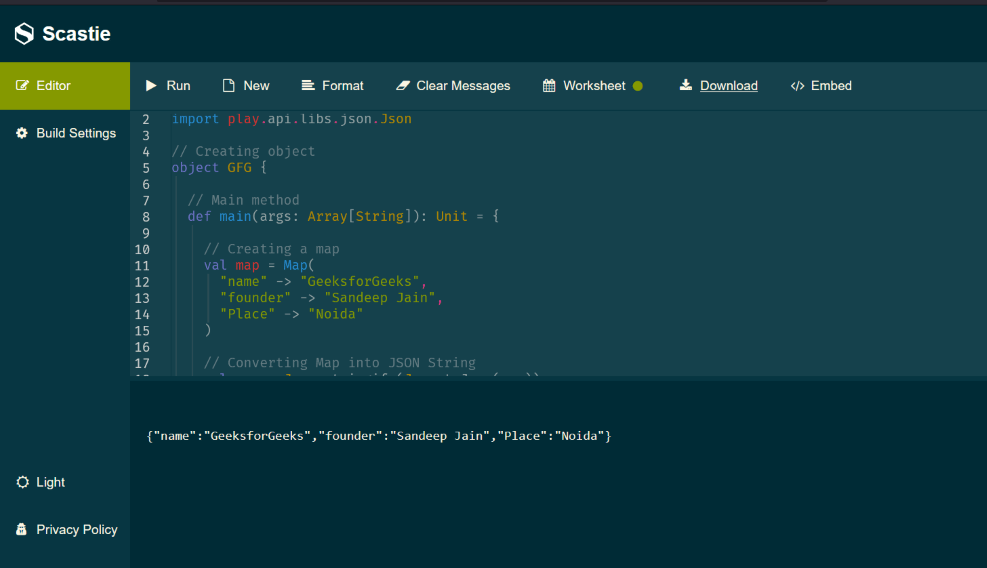
Approach 2: Using Scala Function
- In this approach, we define a custom Scala function conversionFn that takes a Scala Map as input and converts it into a JSON string using string interpolation and map operations.
- The function iterates over the map’s key-value pairs, formats them as JSON key-value pairs, and then joins them together to form a valid JSON string.
Example:
In this example, we are using a Scala function to convert a Map into a JSON String.
Scala
// Scala program to convert Scala Map into JSON String
// Creating object
object GFG {
// Function to convert Map into JSON String
def conversionFn(map: Map[String, String]): String = {
map
.map { case (key, value) => s""""$key":"$value"""" }
.mkString("{", ",", "}")
}
// Main Method
def main(args: Array[String]): Unit = {
// Creating a map
val map = Map(
"name" -> "GeeksforGeeks",
"founder" -> "Sandeep Jain",
"Place" -> "Noida"
)
// JSON String
val res = conversionFn(map)
// Printing Output
println(res)
}
}
Output:
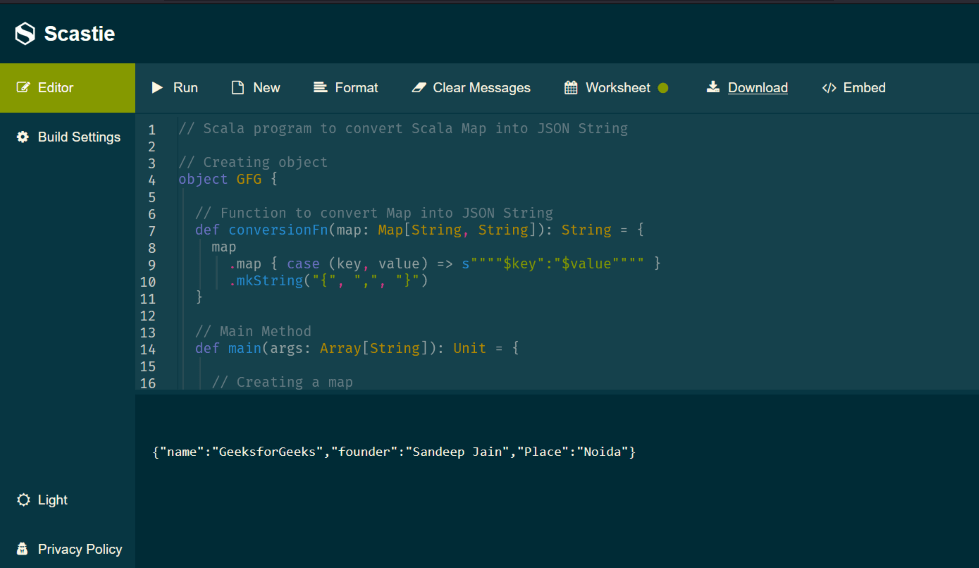
Approach 3: Using Circle Library
- In this approach, we use the circle library to convert a Scala Map into a JSON string.
- The conversionFn function takes a Map[String, String] as input, uses asJson to convert it into a JSON structure, and then noSpaces to format it as a string without any additional spaces.
Example 3:
In this example, we use the Circe library’s asJson and noSpaces methods to convert a Scala Map into a JSON string.
Scala
// Scala program to convert Scala Map into JSON String
import io.circe._
import io.circe.syntax._
// Creating object
object MapToJson {
// Map to JSON String Conversion
def conversionFn(map: Map[String, String]): String = {
map.asJson.noSpaces
}
// Main Method
def main(args: Array[String]): Unit = {
// Creating a map
val map = Map(
"name" -> "GeeksforGeeks",
"founder" -> "Sandeep Jain",
"Place" -> "Noida"
)
// JSON String
val res = conversionFn(map)
// Printing Output
println(res)
}
}
Output:
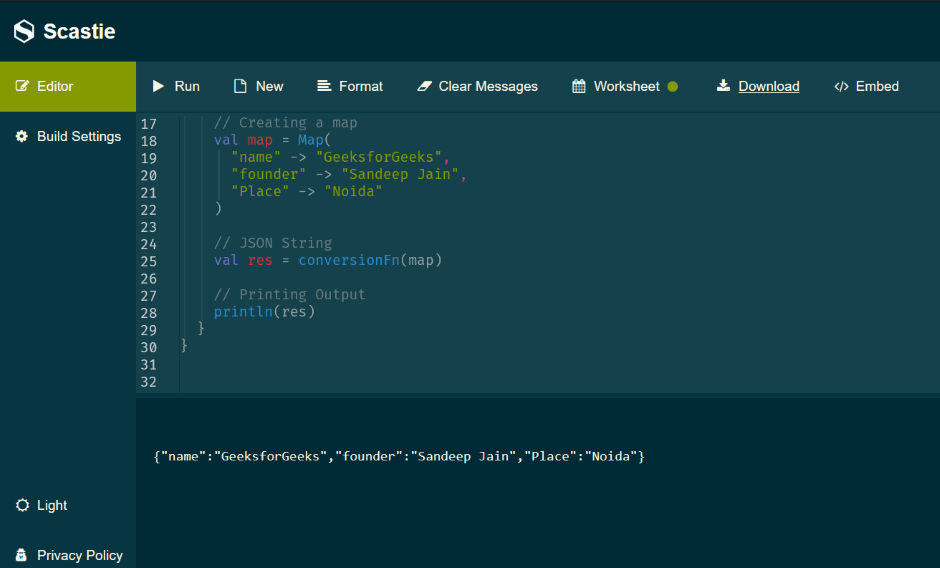
Share your thoughts in the comments
Please Login to comment...