How to convert date and time with different timezones in Python?
Last Updated :
22 Apr, 2021
In this article, we will discuss how to convert date and time with different timezones in Python. To do this we will use one of the modules of python pytz . This module brings the Olson tz database into Python and this library allows accurate and cross-platform timezone calculations using Python. The method pytz.timezone() generates the current timezone of a particular region.
Syntax:
pytz.timezone(“name of the time zone”)
Ex: pytz.timezone(“Asia/Kolkata”)
Example 1:
In the below program the current UTC time is converted according to Asia/Kolkata timezone.
Python3
from datetime import datetime
import pytz
UTC = pytz.utc
IST = pytz.timezone( 'Asia/Kolkata' )
print ( "UTC in Default Format : " ,
datetime.now(UTC))
print ( "IST in Default Format : " ,
datetime.now(IST))
datetime_utc = datetime.now(UTC)
print ( "Date & Time in UTC : " ,
datetime_utc.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
datetime_ist = datetime.now(IST)
print ( "Date & Time in IST : " ,
datetime_ist.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
|
Output:

Example 2:
Here is another program where the current Asia/Kolkata timezone is converted to US/Eastern timezone.
Python3
from datetime import datetime
import pytz
original = pytz.timezone( 'Asia/Kolkata' )
converted = pytz.timezone( 'US/Eastern' )
dateTimeObj = datetime.now(original)
print ( "Original Date & Time: " ,
dateTimeObj.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
dateTimeObj = datetime.now(converted )
print ( "Converted Date & Time: " ,
dateTimeObj.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
|
Output:

One can get all timezone values present in the pytz by executing the below code:
for timezone in pytz.all_timezones:
print(timezone)
Below is a program to convert a particular timezone to multiple timezones of the Indian region:
Python3
from datetime import datetime
import pytz
original = pytz.utc
dateTimeObj = datetime.now(original)
print ( "Original Date & Time: " ,
dateTimeObj.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
for timeZone in pytz.all_timezones:
if 'Indian/' in timeZone:
dateTimeObj = datetime.now(pytz.timezone(timeZone))
print (timeZone, ":" ,dateTimeObj.strftime( '%Y:%m:%d %H:%M:%S %Z %z' ))
|
Output:
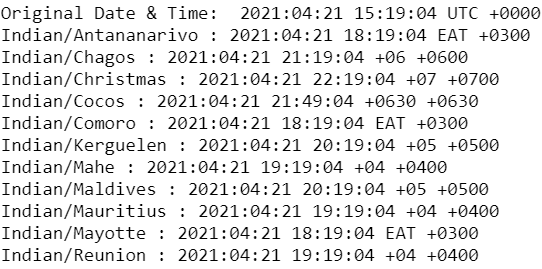
Share your thoughts in the comments
Please Login to comment...