How to convert camel case to snake case in JSON response implicitly using Node.js ?
Last Updated :
02 Feb, 2023
Camel case and snake case are two common conventions for naming variables and properties in programming languages. In camel case, compound words are written with the first word in lowercase and the subsequent words capitalized, with no spaces or underscore between them. For example, firstName is written in camel case. In snake case, compound words are written with all words in lowercase, separated by underscores. For example, first_name is written in snake case.
In this tutorial, we will learn how to convert camel case to snake case in a JSON response using Node.js. We will do this by creating a custom middleware function that will intercept the JSON response and convert it to snake case before it is sent to the client.
Setting Up a Node.js Project: To get started, you will need to have Node.js and npm (the Node.js package manager) installed on your system. If you don’t already have them installed, you can download and install them from the official website (https://nodejs.org/).
Step 1: Once you have Node.js and npm installed, you can create a new Node.js project by creating a new directory and initializing a package.json file. To do this, open a terminal window and navigate to the directory where you want to create your project. Then, run the following commands:
mkdir my-project
cd my-project
npm init -y
This will create a node.js project.
Step 2: Creating a Custom Middleware Function. Next, we will create a custom middleware function that will intercept the JSON response and convert it to a snake case before it is sent to the client. To do this we can use the lodash package, which provides utility functions for working with strings and objects in JavaScript. The lodash package does have a snakeCase function that can be used to convert strings to snake case.
To install the lodash package, run the following command in your terminal:
npm install lodash
Step 3: Once the lodash package is installed, you can create a new file called camel-case-to-snake-case.js in your project directory and add the following code.
camel-case-to-snake-case.js
Javascript
const _ = require( 'lodash' );
function camelCaseToSnakeCase(obj) {
if (obj === null || typeof obj !== 'object' ) {
return obj;
}
const result = {};
for (const key in obj) {
if (obj.hasOwnProperty(key)) {
const snakeCaseKey = _.snakeCase(key);
result[snakeCaseKey] = camelCaseToSnakeCase(obj[key]);
}
}
return result;
}
module.exports = camelCaseToSnakeCase;
|
In this code, we are using the lodash package’s snakeCase function to convert camel case strings to snake case. We are also using recursion to traverse the object and convert all keys to snake case.
Step 4: Using the Custom Middleware Function. To use the custom middleware function, you will need to create a server that sends a JSON response to the client. You can do this using the express package, which is a popular web application framework for Node.js.
To install the express package, run the following command in your terminal:
npm install express
Once the express package is installed, you can create a new file called server.js in your project directory and add the following code.
server.js
Javascript
const express = require( 'express' );
const camelCaseToSnakeCase = require( './camel-case-to-snake-case' );
const app = express();
app.get( '/' , (req, res) => {
const data = {
firstName: 'John' ,
lastName: 'Doe' ,
email: 'john.doe@example.com' ,
};
res.json(camelCaseToSnakeCase(data));
});
app.get( '/second' , (req, res) => {
const data = {
firstName: 'John' ,
jobDesignation: 'Junior Salesman' ,
email_id: 'john.doe@example.com' ,
};
res.json(camelCaseToSnakeCase(data));
});
app.listen(3000, () => {
console.log( 'Server listening on port 3000' );
});
|
Step 5: In this code, we are creating an HTTP server using the express package and listening for GET requests on the root path. When a GET request is received, we are sending a JSON response to the client that contains the data object, which has keys that are written in camel case. We are using the res.json method to send the response, which automatically sets the content type to application/json and sends the data as a JSON string.
The project structure is as follows:
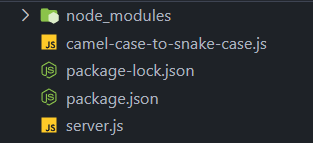
project structure inside ‘my-project’
Step 6: Before sending the response, we are using the camelCaseToSnakeCase function to convert the camel case keys in the data object to snake case. This ensures that the JSON response sent to the client will have keys written in snake case. To start the server, open a terminal window and navigate to the root directory of your project. Then, run the following command:
node server.js
This will start the server, and you can test it by opening a web browser and navigating to http://localhost:3000. You should see a JSON response with keys written in snake case.
We get the following response with the keys converted to snake case:
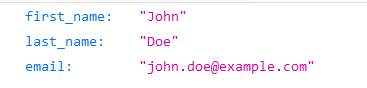
object with snake case keys
Output: You can go to http://localhost:3000/second to check the response for the second endpoint too.
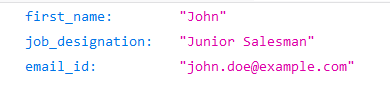
keys are in snake case
In this tutorial, we learned how to convert camel case to snake case in a JSON response using Node.js. We did this by creating a custom middleware function that used the lodash package’s snakeCase function. We then used the custom middleware function to intercept the JSON response before it was sent to the client, ensuring that the keys were written in snake case.
Share your thoughts in the comments
Please Login to comment...