How to Write Postman Test to Compare the Response JSON against another JSON?
Last Updated :
03 Apr, 2024
In the era of API-driven development, testing the API responses to ensure their correctness is of paramount importance. One such test includes comparing the JSON response of an API against a predefined JSON. In this article, we will delve into how to write a Postman test for this purpose.
What is Postman?
Postman is a popular API client that offers features for designing, debugging, testing, documenting, and monitoring APIs. With its user-friendly interface, developers can send HTTP requests to a server and view the responses.
JSON and its Role in APIs:
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is primarily used to transmit data between a server and a web application, serving as an alternative to XML.
Why Compare JSON Responses?
When developing or testing APIs, it’s often necessary to compare the actual JSON response of an API to a predefined or expected JSON. This comparison helps in validating the correctness and consistency of the API’s response.
Approach 1: Using JavaScript Code in the Tests Tab
Postman allows users to write JavaScript code in the ‘Tests’ section of a request. Here’s a simple way to compare two JSON objects:
JavaScript
var serverData = JSON.parse(responseBody);
var JSONtoCompare = {}; // Insert your predefined JSON here.
tests["Body is correct"] = _.isEqual(serverData, JSONtoCompare);
Approach 2: Using Postman’s pm.expect Method
Postman provides a built-in library, pm, for writing test assertions. Here’s an example of how to use pm.expect to compare JSON responses:
JavaScript
pm.test("Your test name", function () {
var jsonData = pm.response.json();
pm.expect(jsonData).to.eql({
"key1": "value1",
"key2": 100
});
});
Steps to Set Up a Postman Test:
Now that we’ve explored the different approaches to comparing JSON responses, let’s walk through the steps of setting up a Postman test.
Step 1. Open Postman and create a new request or select an existing one.
- Choose the request type (e.g., GET, POST, etc.) from the dropdown menu.
- Enter the request URL in the address bar and any necessary headers.
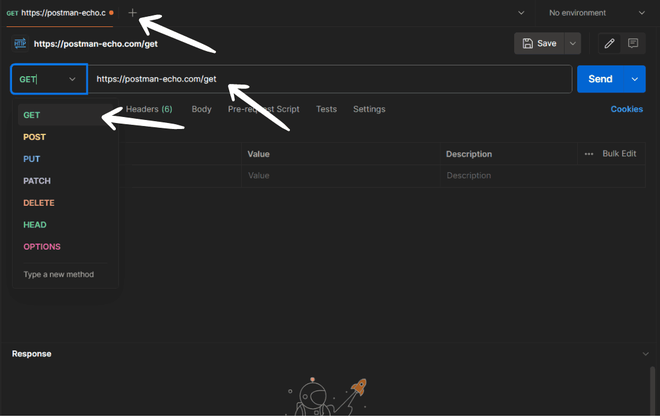
Step 2. Enter Request Details in the ‘Body’ Tab:
- If the request requires a body (e.g., for POST or PUT requests), navigate to the ‘Body’ tab within the request editor.
- Enter the necessary details such as JSON, form data, or raw text according to your API’s requirements.
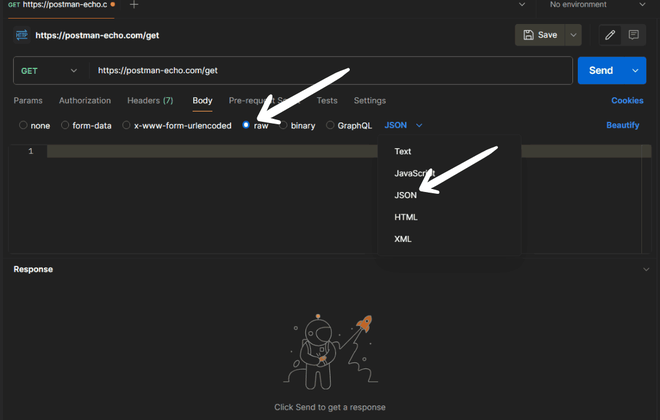
Step 3. Go to the ‘Tests’ tab and write your test script using one of the methods described above.
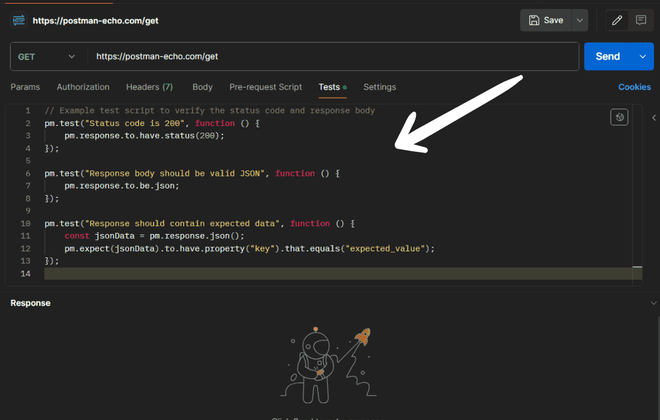
Step 4. Click ‘Send’ to execute the request and run the test.
- Once you’ve written your test script, click the blue ‘Send’ button located at the top-right corner to execute the request and run the tests.
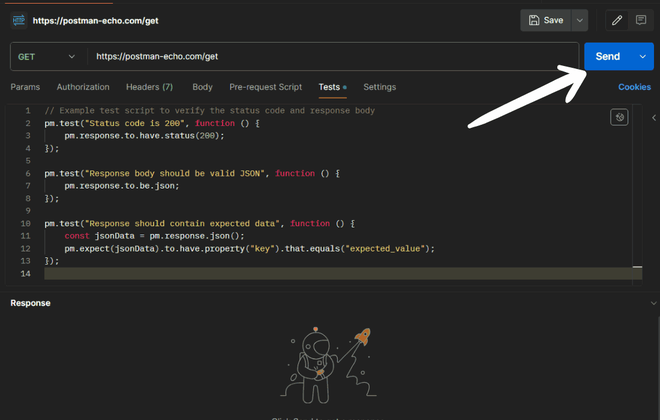
Step 5. The test results will appear in the ‘Test Results’ section.
- After the request has been sent and the tests have run, the test results will appear in the ‘Test Results’ section at the bottom of the Postman window.
- Review the test results to see if each test passed or failed.
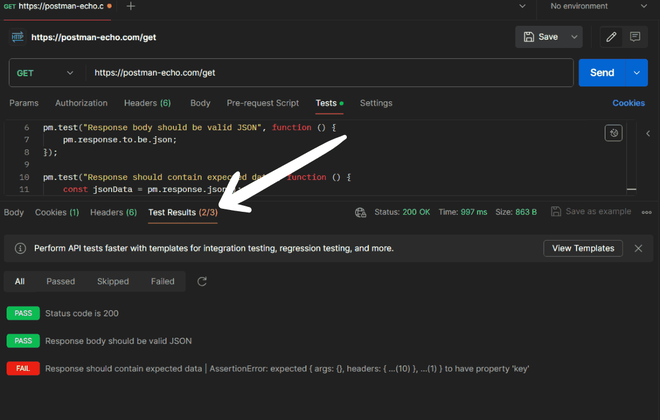
Example: To illustrate, let’s create a Postman test using the pm.expect method. Suppose we have an API that returns user details, and we want to validate that the username and email are as expected.
Our test script in Postman would look something like this:
pm.test("Check user details", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.username).to.eql("michael");
pm.expect(jsonData.email).to.eql("michael@example.com");
});
After running the request, the test results will confirm whether the username and email in the response match our expectations.
Conclusion:
By using Postman’s powerful features and a bit of JavaScript, we can effectively compare the JSON response of an API against a predefined JSON. This practice is a key part of API testing, helping to ensure the accuracy and consistency of the API’s responses. Whether you’re comparing simple key-value pairs or complex JSON objects with nested arrays, Postman offers flexible solutions for your testing needs. By understanding and implementing these methods, you can elevate your API testing to a new level.
Share your thoughts in the comments
Please Login to comment...