How to Connect Node to a MongoDB Database ?
Last Updated :
14 Jan, 2024
MongoDB is a NoSQL database used to store large amounts of data without any traditional relational database table. Instead of rows & columns, MongoDB used collections & documents to store data. A collections consist of a set of documents & a document consists of key-value pairs which are the basic unit of data in MongoDB.
Make sure that MongoDB installs on your PC.
To connect a Node.js application to MongoDB, we have to use a library called Mongoose. Â
const mongoose = require("mongoose");
After that, we have to call the connect method of Mongoose
mongoose.connect("mongodb://localhost:27017/collectionName", {
useNewUrlParser: true,
useUnifiedTopology: true
});
Then we have to define a schema. A schema is a structure, that gives information about how the data is being stored in a collection.
Example: Suppose we want to store information from a contact form of a website.
const contactSchema = {
email: String,
query: String,
};
Then we have to create a model using that schema which is then used to store data in a document as objects.
const Contact = mongoose.model("Contact", contactSchema);
Then, finally, we are able to store data in our document.
app.post("/contact", function (req, res) {
const contact = new Contact({
email: req.body.email,
query: req.body.query,
});
contact.save(function (err) {
if (err) {
res.redirect("/error");
} else {
res.redirect("/thank-you");
}
});
});
Example: Below is the code example of how to connect node.js to a mongodb database.
Javascript
const express = require( "express" );
const ejs = require( "ejs" );
const mongoose = require( "mongoose" );
const bodyParser = require( "body-parser" );
mongoose.connect(
{
useNewUrlParser: true ,
useUnifiedTopology: true
});
const contactSchema = {
email: String,
query: String,
};
const Contact =
mongoose.model( "Contact" , contactSchema);
const app = express();
app.set( "view engine" , "ejs" );
app.use(bodyParser.urlencoded({
extended: true
}));
app.use(express.static(__dirname + '/public' ));
app.get( "/contact" ,
function (req, res) {
res.render( "contact" );
});
app.post( "/contact" ,
function (req, res) {
console.log(req.body.email);
const contact = new Contact({
email: req.body.email,
query: req.body.query,
});
contact.save( function (err) {
if (err) {
throw err;
} else {
res.render( "contact" );
}
});
});
app.listen(3000,
function () {
console.log( "App is running on Port 3000" );
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible"
content = "IE=edge" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Document</ title >
</ head >
< body >
< form action = "/contact" method = "post" >
< input type = "text"
placeholder = "Email"
name = "email" >
< input type = "text"
placeholder = "Query"
name = "query" >
< button type = "submit" >
Submit
</ button >
</ form >
</ body >
</ html >
|
Output:
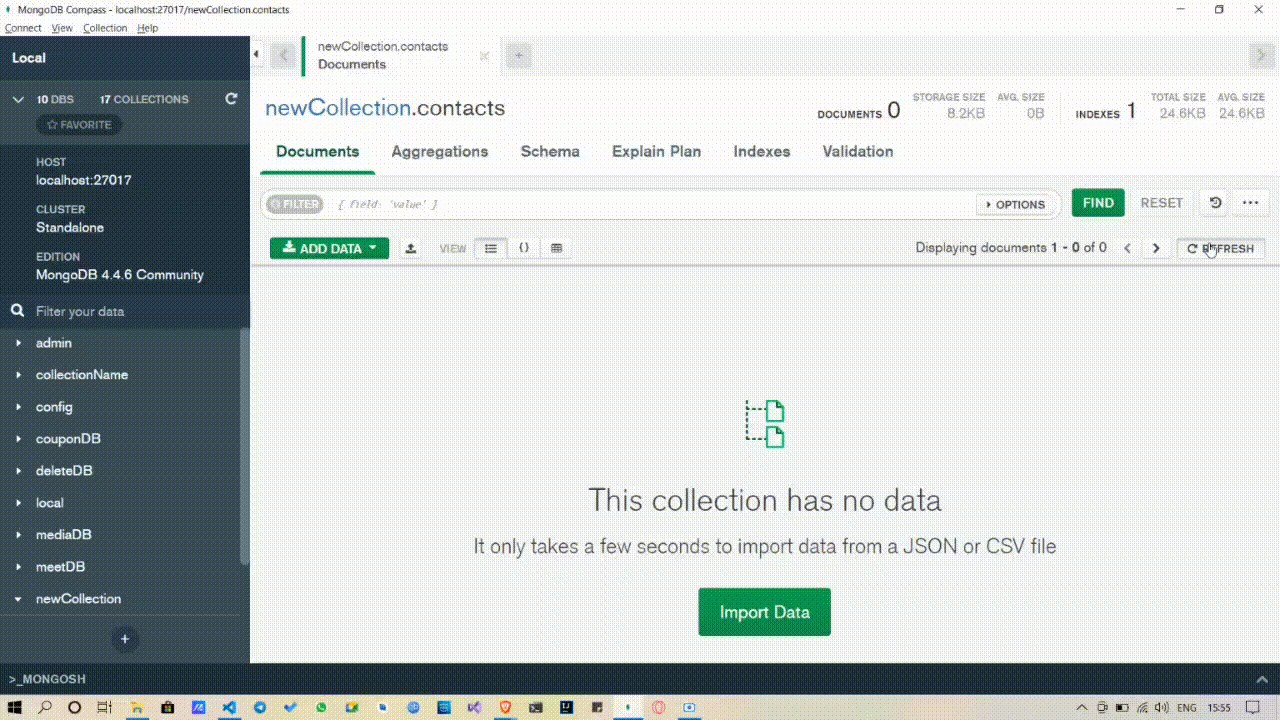
Output
Share your thoughts in the comments
Please Login to comment...