How to check the current runtime environment is a browser in JavaScript ?
Last Updated :
18 Apr, 2021
In this article, we will see how to detect the runtime environment in which our JavaScript code is running. Suppose you are building an application in Node.js and need to include that code in a webpage that would be run on a browser. This would cause some functions of a Node application not to work on browsers and vice-versa.
Approach: There is no function, method, or property to detect if the runtime environment is a browser, however, we can make some checks to identify whether a script is running in the browser. Some environments in which the JavaScript code can run are Node.js, Service Workers, or in a web browser.
There are different conditions for each type of environment. We will create a function that will return a boolean value indicating whether the environment is a browser or not. In this function,
- We first check if the process is of the type ‘object’ and the type of require is a function using the typeof operator. When both the conditions are true, then the environment is Node.js, hence we return false.
- We similarly check if the environment is a service worker by checking if the type of importScripts is a function. We again return false if the condition matches.
- In the end, we check the type of window to be equal to an ‘object’. A true condition indicates that the environment is a browser, and we return true from the function.
Syntax:
function isBrowser() {
// Check if the environment is Node.js
if (typeof process === "object" &&
typeof require === "function") {
return false;
}
// Check if the environment is a
// Service worker
if (typeof importScripts === "function") {
return false;
}
// Check if the environment is a Browser
if (typeof window === "object") {
return true;
}
}
Example:
HTML
<!DOCTYPE html>
< html >
< body >
< h1 >Hello Geeks</ h1 >
< script >
function isBrowser() {
// Check if the environment is Node.js
if (typeof process === "object" &&
typeof require === "function") {
return false;
}
// Check if the environment is
// a Service worker
if (typeof importScripts === "function") {
return false;
}
// Check if the environment is a Browser
if (typeof window === "object") {
return true;
}
}
// Calling a alert if the environment is Browser
if (isBrowser()) {
alert("The Environment is Browser....")
}
</ script >
</ body >
</ html >
|
Output:
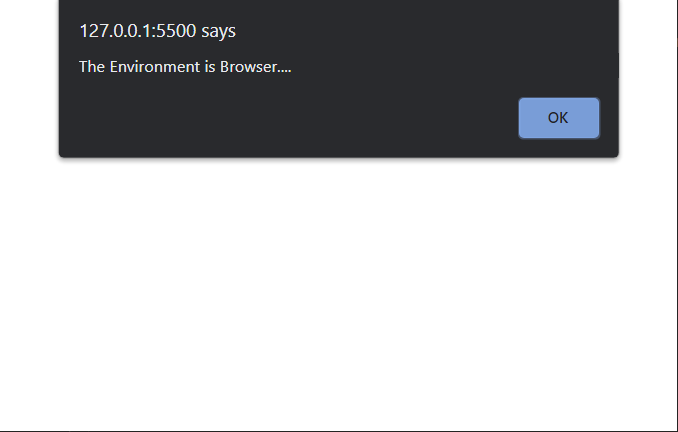
Share your thoughts in the comments
Please Login to comment...