How to change language in react-day-picker DayPickerInput component ?
Last Updated :
04 Dec, 2023
React, a declarative and efficient JavaScript library for UI development, focuses on the ‘V’ (View) in MVC, serving as an open-source, component-based front-end tool maintained by Facebook. The react-day-picker enhances date picking in web apps, featuring the React DayPickerInput component for an input field linked to an overlay displaying the DayPicker.
Prerequisites:
Syntax:
<DayPicker locale="*" weekdaysLong="*" weekdaysShort="*" months="*" />
Steps to Create the React Application And Installing Module:
Step 1: Create a react application using the following command.
npx create-react-app foldername
Step 2: Once it is done, change your directory to the newly created application using the following command.
cd foldername
Step 3: Install the module using the following command:
npm install react-day-picker
npm install moment
Project Structure:
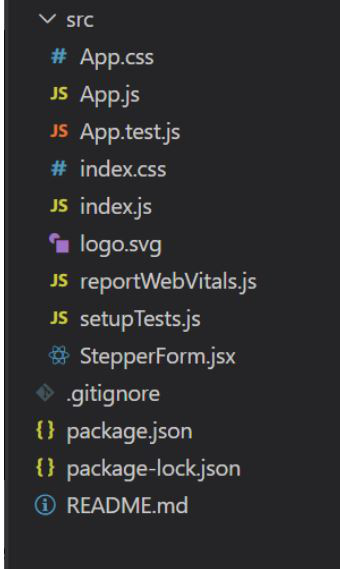
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-day-picker": "^8.9.1",
"react-dom": "^18.2.0",
"react-icons": "^4.12.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Approach:
In order to change the language of DatePickerInput, we need to use the dayPickerProp i.e locale. This is known as localization.
- Localization: The react-day-picker can be localized into any language (English being the default). This can be done by:
- Using dayPickerProp (locale)
- Using moment.js
- Using Locale
- Locale is used to set up language in DateInputPicker. It can be any language. We need to define some other props also based upon the language like weekdaysLong, weekdaysShort, months.
Example 1: In this example, we will preDefine Months, weekdays for the language. Then we will render them accordingly.
Javascript
import React from "react" ;
import DayPicker from "react-day-picker" ;
import "react-day-picker/lib/style.css" ;
class LocalizedExample extends React.Component {
constructor(props) {
super (props);
this .handleLanguage = this .handleLanguage.bind( this );
this .state = {
locale: "en" ,
};
}
WEEKDAYS_LONG = {
en: [
"Sunday" ,
"Monday" ,
"Tuesday" ,
"Wednesday" ,
"Thursday" ,
"Friday" ,
"Saturday" ,
],
it: [
"Domenica" ,
"Lunedì" ,
"Martedì" ,
"Mercoledì" ,
"Giovedì" ,
"Venerdì" ,
"Sabato" ,
],
};
WEEKDAYS_SHORT = {
en: [ "Su" , "Mo" , "Tu" , "We" , "Th" , "Fr" , "Sa" ],
it: [ "D" , "L" , "M" , "M" , "G" , "V" , "S" ],
};
MONTHS = {
en: [
"January" ,
"February" ,
"March" ,
"April" ,
"May" ,
"June" ,
"July" ,
"August" ,
"September" ,
"October" ,
"November" ,
"December" ,
],
it: [
"Gennaio" ,
"Febbraio" ,
"Marzo" ,
"Aprile" ,
"Maggio" ,
"Giugno" ,
"Luglio" ,
"Agosto" ,
"Settembre" ,
"Ottobre" ,
"Novembre" ,
"Dicembre" ,
],
};
handleLanguage(e) {
this .setState({
locale: e.target.value,
});
}
render() {
return (
<div>
<p>
<select onChange={ this .handleLanguage}>
<option value= "en" selected>
English
</option>
<option value= "it" >Italian</option>
</select>
</p>
<DayPicker
locale={ this .state.locale}
months={ this .MONTHS[ this .state.locale]}
weekdaysLong={ this .WEEKDAYS_LONG[ this .state.locale]}
weekdaysShort={ this .WEEKDAYS_SHORT[ this .state.locale]}
/>
</div>
);
}
}
function App() {
return (
<div className= "App" >
<div>
<h1 style={{ color: "green" }}>GeeksforGeeks</h1>
<h3>Changing Language in React DayInputPicker using Locale</h3>
<br></br>
<LocalizedExample />
</div>
</div>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:
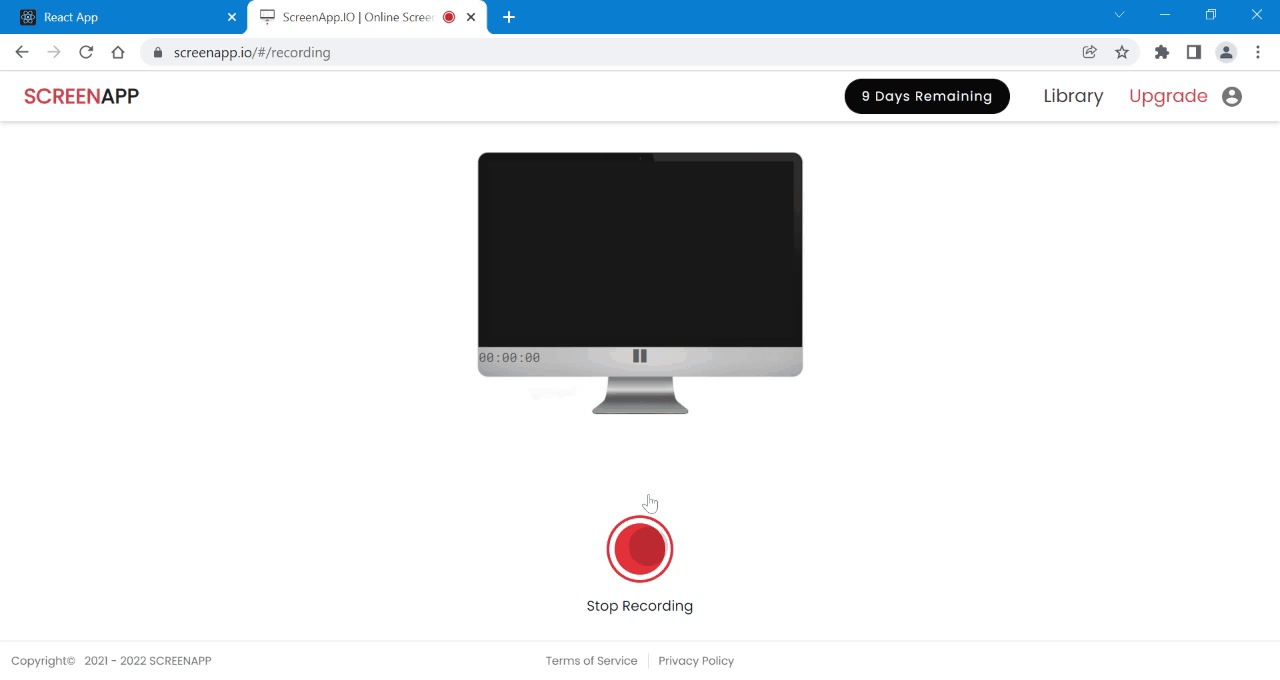
Example 2: In this example, we will again have a dropdown menu for selecting Language, and the calendar will render accordingly. This time we don’t need to specifically mention weekdays and other props. These are imported using moment, for example, import ‘moment/locale/hi’ for Hindi language weeks, days, etc.
Javascript
import React from "react" ;
import { useState } from "react" ;
import DayPicker from "react-day-picker" ;
import "react-toastify/dist/ReactToastify.css" ;
import "react-day-picker/lib/style.css" ;
import MomentLocaleUtils from "react-day-picker/moment" ;
import "moment/locale/ta" ;
import "moment/locale/ar" ;
import "moment/locale/hi" ;
import "moment/locale/it" ;
import "moment/locale/ur" ;
class MomentLocalizedExample extends React.Component {
constructor(props) {
super (props);
this .handleLanguage = this .handleLanguage.bind( this );
this .state = {
locale: "en" ,
};
}
handleLanguage(e) {
this .setState({
locale: e.target.value,
});
}
render() {
return (
<div>
<p>
<select onChange={ this .handleLanguage}>
<option value= "en" selected>
English
</option>
<option value= "hi" >Hindi</option>
<option value= "ur" >Urdu</option>
<option value= "ta" >Tamil</option>
<option value= "ar" >Arabic</option>
<option value= "it" >Italian</option>
</select>
</p>
<DayPicker
localeUtils={MomentLocaleUtils}
locale={ this .state.locale}
/>
</div>
);
}
}
function App() {
return (
<div className= "App" >
<div>
<h1 style={{ color: "green" }}>GeeksforGeeks</h1>
<h3>
Changing Language in React DayInputPicker using Moment.JS
</h3>
<br></br>
<MomentLocalizedExample />
</div>
</div>
);
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:
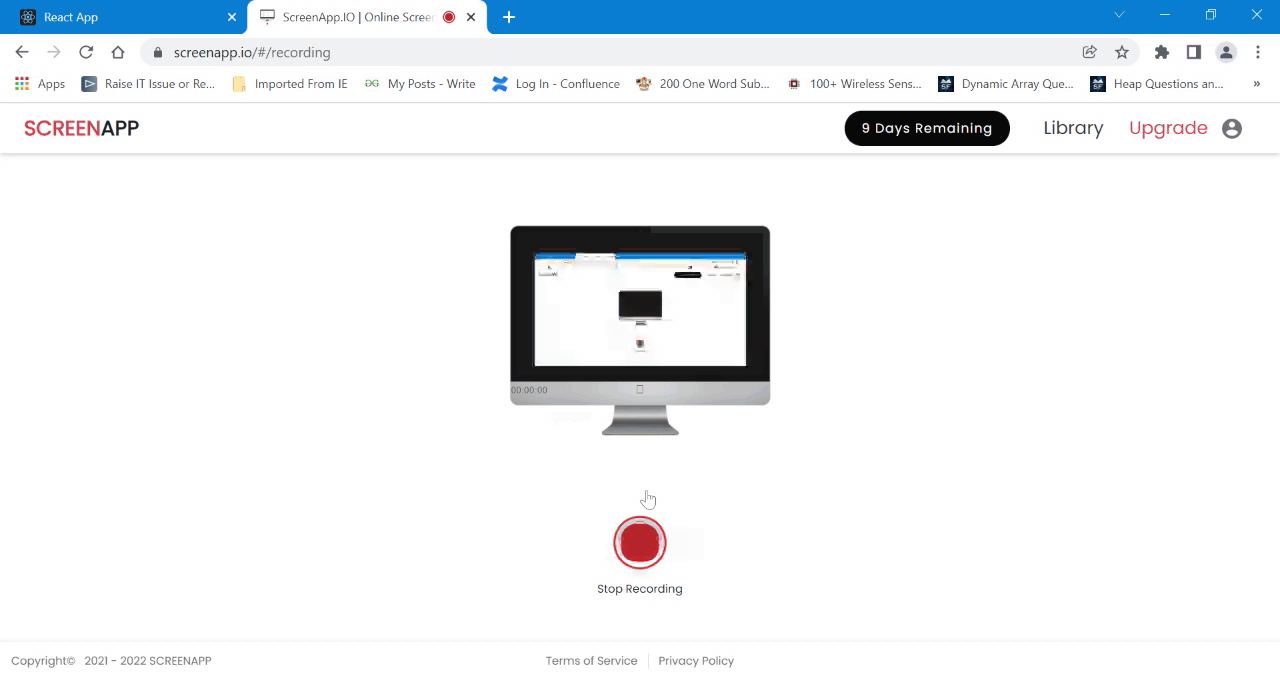
Share your thoughts in the comments
Please Login to comment...