How to change font color of the active nav-item in Bootstrap ?
Last Updated :
08 Sep, 2021
There are two ways that you can change the font color of the active nav-item. The default font-color is white of the active nav-link in the case of Bootstrap nav-active item. The first-way approach is to use a CSS styling file and changing the nav-item class when clicked.
The second-way approach is to change the color using jQuery .css() property and also changing the class status to active for a nav-item using jQuery.
Approach 1: Providing an Overriding CSS Styling: To change the color of the font an overriding styling file can be added to the HTML file. This style file will be used to change the font-color of the selected nav-item. When a nav-item is selected, this style file will be added to that particular nav-item.
Example:
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 ,
shrink-to-fit = no " />
< link rel = "stylesheet"
href =
integrity =
"sha384-TX8t27EcRE3e/ihU7zmQxVncDAy5uIKz4rEkgIXeMed4M0jlfIDPvg6uqKI2xXr2"
crossorigin = "anonymous" />
< script src =
</ script >
< script src =
</ script >
< title >Active Link using css style</ title >
< style >
/*Code to change color of active link*/
.navbar-nav > .active > a {
color: red;
}
</ style >
</ head >
< body >
< ul class = "navbar-nav" >
< li class = "nav-item active" >
< a class = "nav-link" href = "#" >
Home
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link" href = "#" >
GeeksForGeeks
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link" href = "#" >
Others
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link disabled"
href = "#" >Disabled</ a >
</ li >
</ ul >
< script >
$(document).ready(function () {
$("ul.navbar-nav > li").click(function (e) {
$("ul.navbar-nav > li").removeClass("active");
$(this).addClass("active");
});
});
</ script >
</ body >
</ html >
|
Output:
Here you can observer the color of the active link is changed to red.
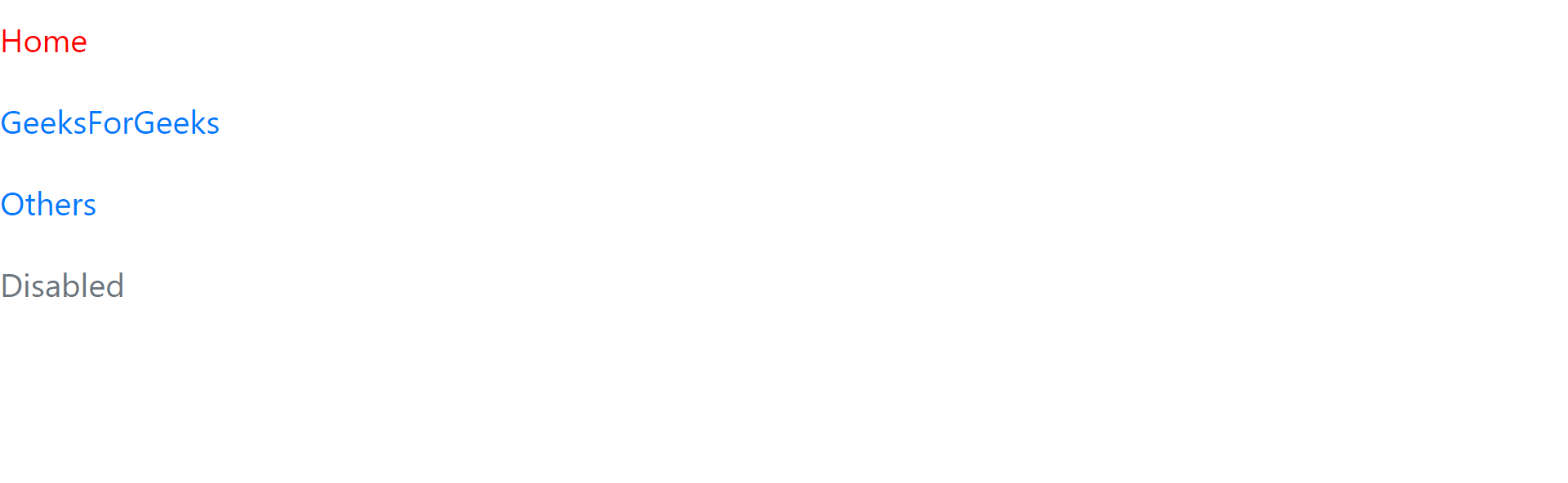
Home link is clicked and its font-color changed to red
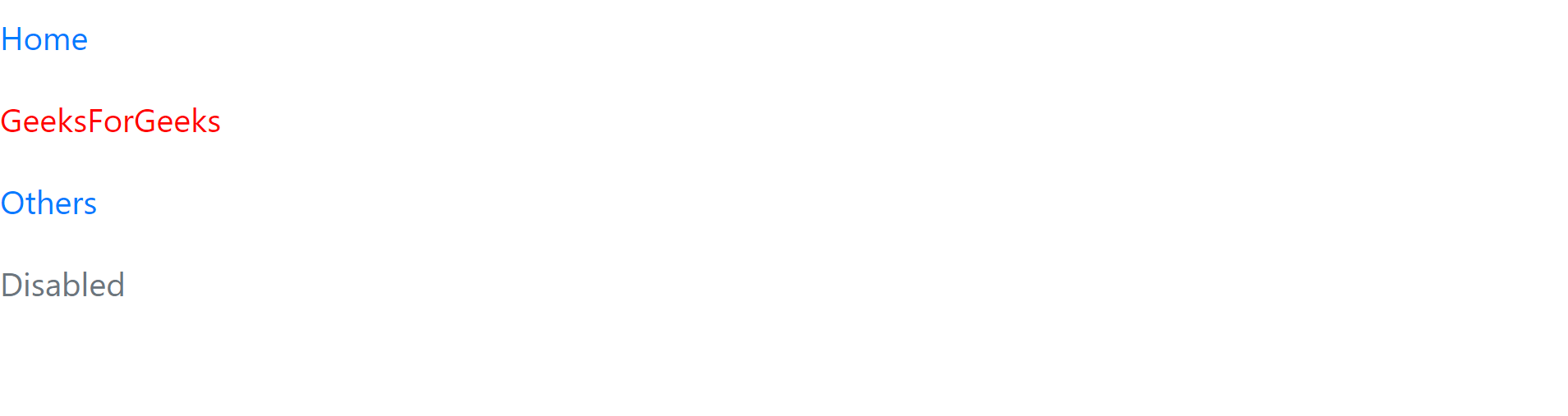
GeeksForGeeks nav item is clicked
Approach 2: To change font-color of active-nav using jQuery: To change the font-color of the active-nav link using jQuery, we need to add the active class to that particular nav-item and then we just need to apply the .css(property,value) to change the font- color of the active nav-item.
Example:
html
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 ,
shrink-to-fit = no " />
< link rel = "stylesheet"
href =
integrity =
"sha384-TX8t27EcRE3e/ihU7zmQxVncDAy5uIKz4rEkgIXeMed4M0jlfIDPvg6uqKI2xXr2"
crossorigin = "anonymous" />
< script src =
</ script >
< script src =
</ script >
< title >Active Link font color using jquery</ title >
</ head >
< body >
< ul class = "navbar-nav" >
< li class = "nav-item active" >
< a class = "nav-link" href = "#" >
Home
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link" href = "#" >
GeeksForGeeks
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link" href = "#" >
Others
</ a >
</ li >
< li class = "nav-item" >
< a class = "nav-link disabled"
href = "#" >Disabled</ a >
</ li >
</ ul >
< script type = "text/javascript" >
$(document).ready(function () {
$("ul.navbar-nav > li > a").click(
function (e) {
$("ul.navbar-nav > li").removeClass(
"active");
$("ul.navbar-nav > li > a").css(
"color", "");
$(this).addClass("active");
$(this).css("color", "red");
});
});
</ script >
</ body >
</ html >
|
Output:
The active link color changed to red.
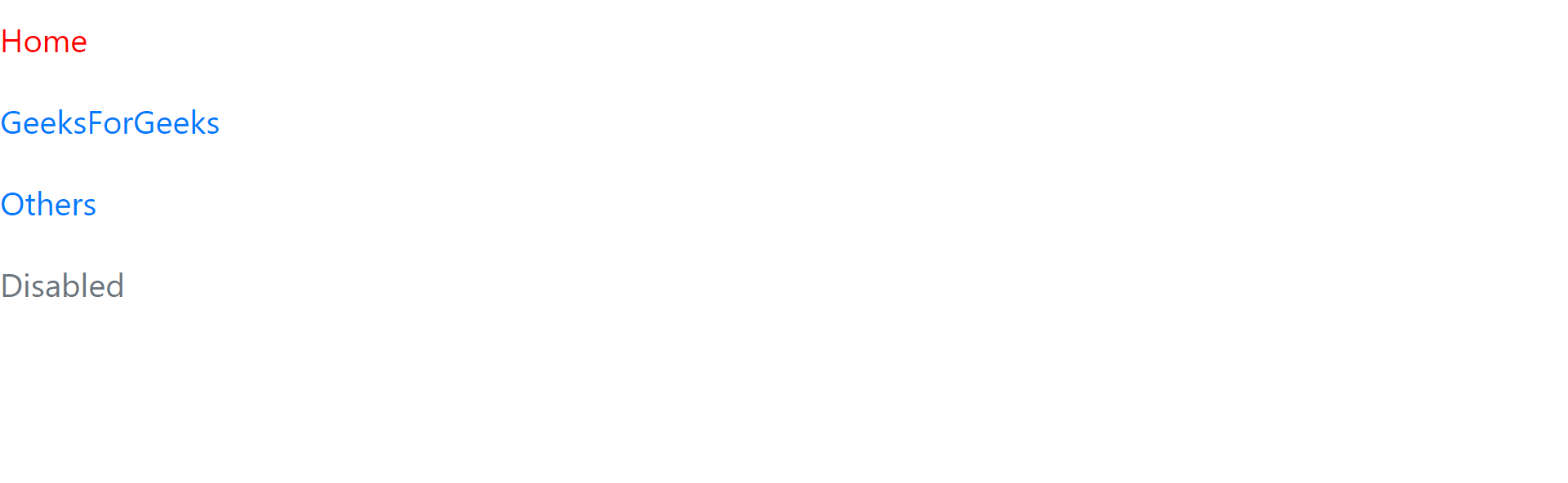
Home link is clicked and its font-color changed to red. Here color is changed using jquery.
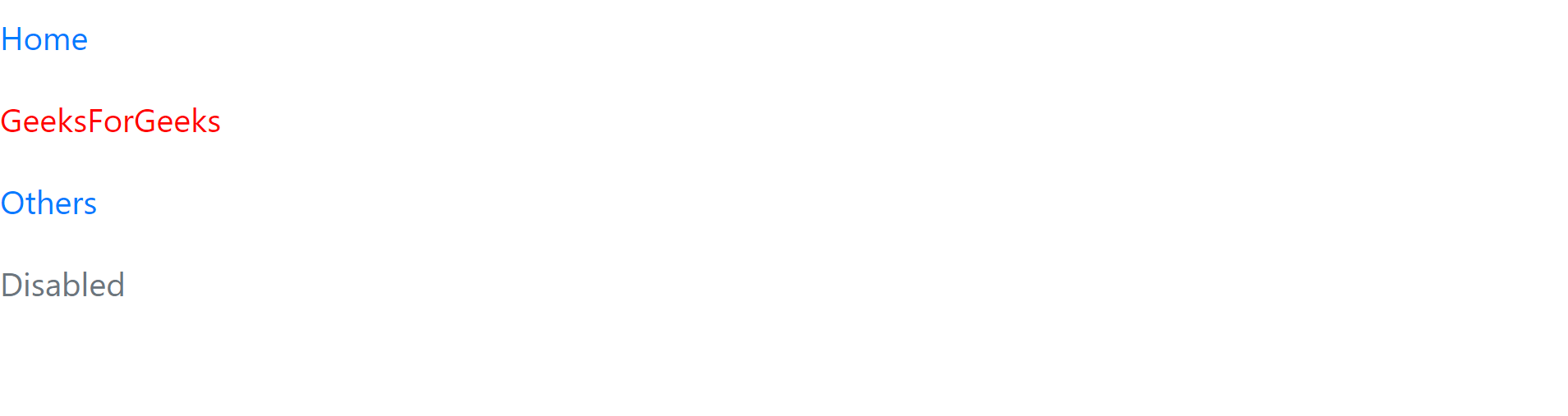
GeeksForGeeks nav item is clicked
Note: The Output of both method will be same. When a particular nav-item is clicked it will change the font-color.
Share your thoughts in the comments
Please Login to comment...