How to center a popup window on screen?
Last Updated :
22 Dec, 2023
JavaScript window.open() method is used to open a popup window. This popup window will be placed in the center of the screen.
- popupWinHeight: The height of the pop-up window on the screen.
- popupWinWidth: The width of the pop-up window on the screen.
Example 1: This example creates the pop-up window without placing it into the center.
html
<!DOCTYPE html>
< html >
< head >
< title >
Non-centered popup window
on the screen
</ title >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< p >
Non-centered popup window
on the screen
</ p >
< script >
function createPopupWin(pageURL, pageTitle,
popupWinWidth, popupWinHeight) {
let left = (screen.width);
let top = (screen.height);
let myWindow = window.open(pageURL, pageTitle,
'resizable=yes, width=' + popupWinWidth
+ ', height=' + popupWinHeight + ', top='
+ top + ', left=' + left);
}
</ script >
'GeeksforGeeks Website', 1200, 650);">
GeeksforGeeks
</ button >
</ body >
</ html >
|
Output:
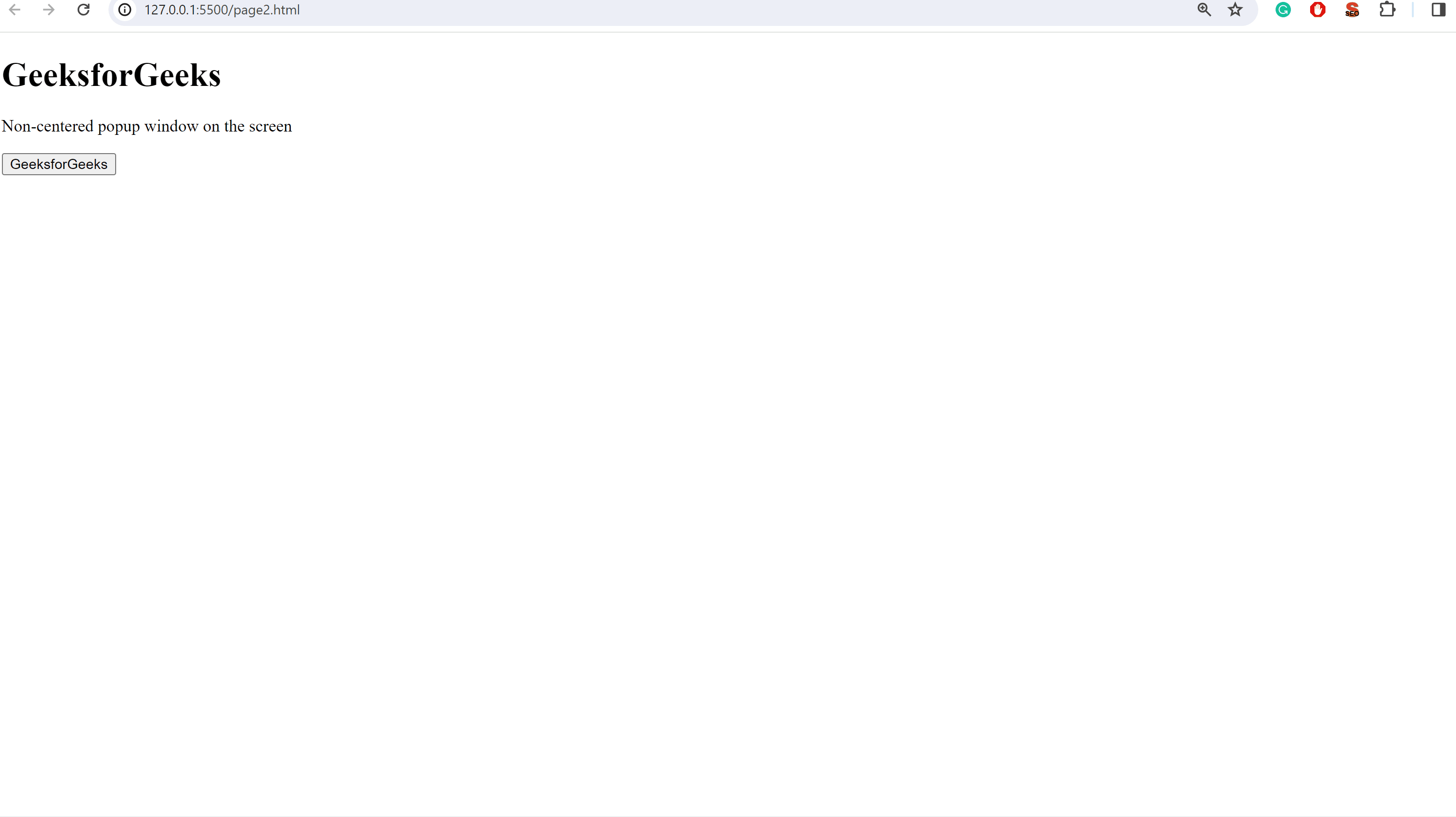
Opening popup anywhere at the screen
Center popup window: To center the popup window we are changing the values of parameters of open() method as follows:
- left = (screen.width – popupWinWidth) / 2
- top = (screen.height – popupWinHeight) / 4
Example 2: This example creates the pop up window and placing it into center.
html
<!DOCTYPE html>
< html >
< head >
< title >
Centered popup window
on the screen
</ title >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< p >
Centered popup window
on the screen
</ p >
< script >
function createPopupWin(pageURL, pageTitle,
popupWinWidth, popupWinHeight) {
let left = (screen.width - popupWinWidth) / 2;
let top = (screen.height - popupWinHeight) / 4;
let myWindow = window.open(pageURL, pageTitle,
'resizable=yes, width=' + popupWinWidth
+ ', height=' + popupWinHeight + ', top='
+ top + ', left=' + left);
}
</ script >
'GeeksforGeeks Website', 1200, 650);">
GeeksforGeeks
</ button >
</ body >
</ html >
|
Output:
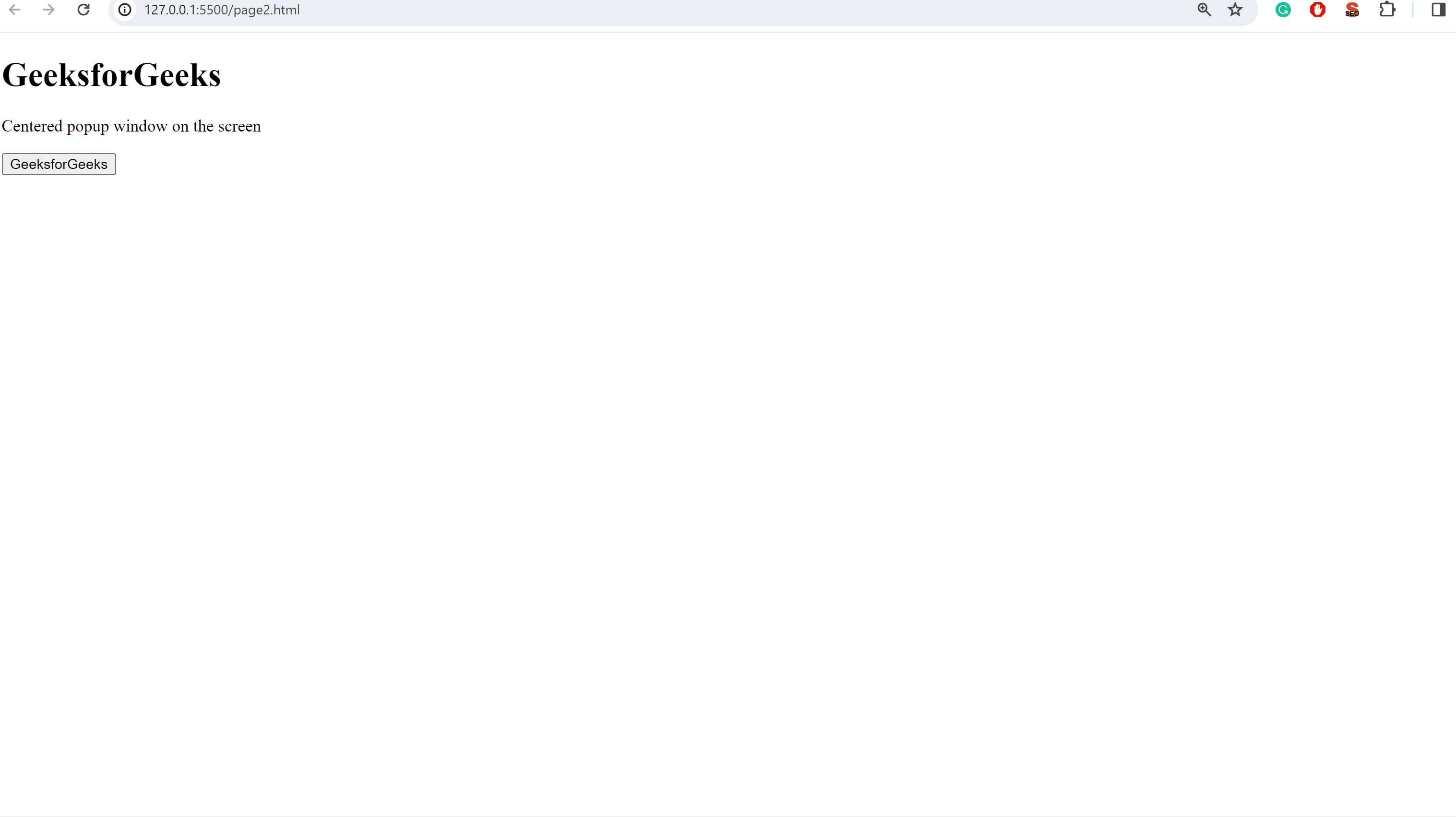
Opening popup box at the center of the screen
Share your thoughts in the comments
Please Login to comment...