How to Call a Vue.js Function on Page Load ?
Last Updated :
15 Jan, 2024
In this article, we will learn how to call a vue.js function on page load in VueJS. We will explore two different approaches with practical implementation in terms of examples and outputs.
Using mounted Hook
In this approach, are using the mounted lifecycle hook. We have defined a function as loadFn which is automatically called using a mounted hook. This hook is executed when the Vue instance is mounted to the DOM.
Syntax:
mounted() {
// logic to call function
}
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
< script src =
</ script >
< style >
body {
font-family: 'Arial', sans-serif;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 style = "color: green;" >
{{ message }}
</ h1 >
< h3 >
Approach 1: Using mounted Hook
</ h3 >
</ div >
< script >
new Vue({
el: '#app',
data: {
message: 'GeeksforGeeks',
},
mounted() {
this.loadFn();
},
methods: {
loadFn() {
alert
('Page Load. Function Executed');
},
},
});
</ script >
</ body >
</ html >
|
Output:
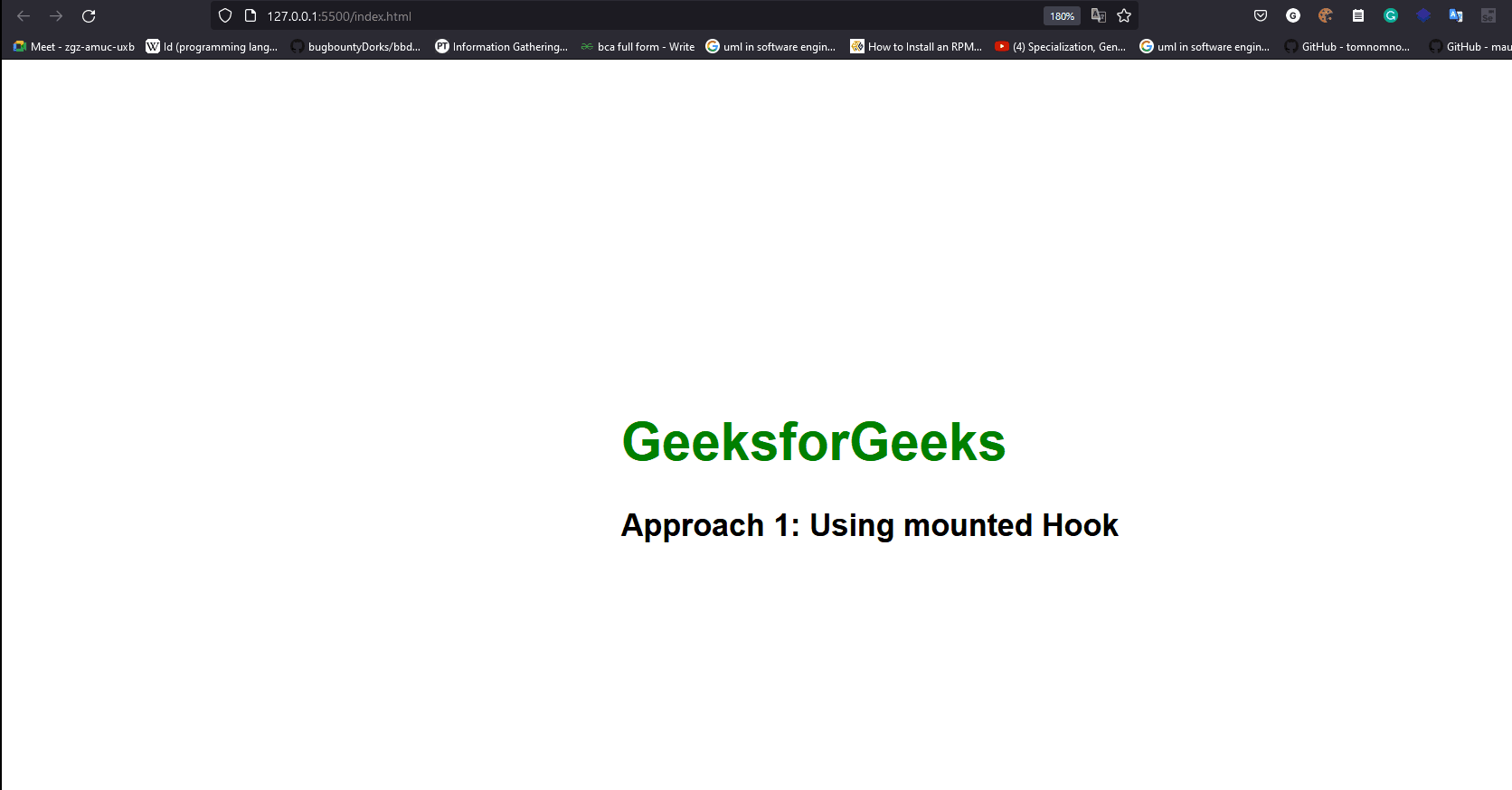
Using created Hook
In this approach, we are using the created Hook to call the loadFnLog method on instance creation. We are logging the initial message. When the user clicks on the button, the message is updated and it is also been logged in the console.
Syntax:
created() {
// logic to call load fn
},
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 2</ title >
< script src =
</ script >
< style >
body {
font-family: Arial, sans-serif;
text-align: center;
}
#app {
text-align: center;
color: green;
}
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 >
{{ message }}
</ h1 >
< h2 >
Approach 2: Using created Hook
</ h2 >
< button @ click = "changeMessage" >
Change Message
</ button >
</ div >
< script >
new Vue({
el: '#app',
data: {
message: 'Hello, GeeksforGeeks!'
},
created() {
this.loadFnLog();
},
methods: {
changeMessage() {
this.message = 'GFG';
this.loadFnLog();
},
loadFnLog() {
console.log
('Current Message:', this.message);
}
}
});
</ script >
</ body >
</ html >
|
Output:
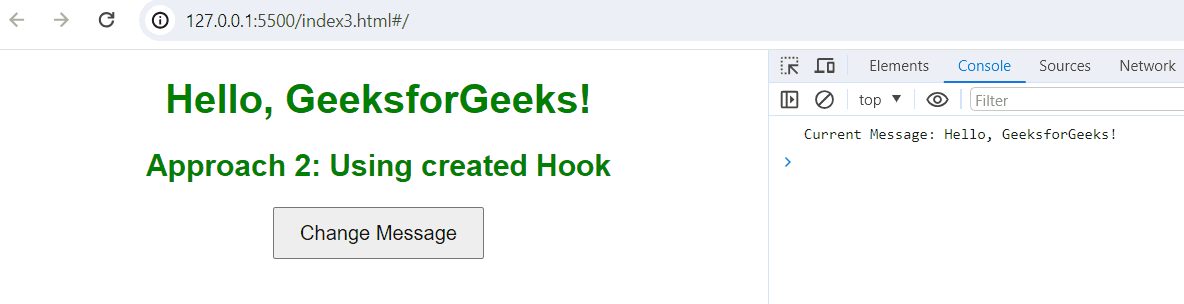
Share your thoughts in the comments
Please Login to comment...